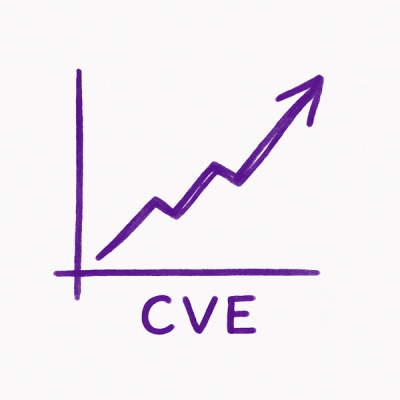
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
reactive-graphql
Advanced tools
GraphQL reactive executor
Execute GraphQL queries against reactive resolvers (resolvers that return Observable) to get a reactive results.
$ npm i reactive-graphql --save
The usage is very similar to graphql-js
's graphql
function, except that:
import { graphql } from "reactive-graphql";
import { makeExecutableSchema } from "graphql-tools";
import { timer } from "rxjs";
const typeDefs = `
type Query {
time: Int!
}
`;
const resolvers = {
Query: {
// resolvers can return an Observable
time: () => {
// Observable that emits increasing numbers every 1 second
return timer(1000, 1000);
}
}
};
const schema = makeExecutableSchema({
typeDefs,
resolvers
});
const query = `
query {
time
}
`;
const stream = graphql(schema, query);
// stream is an Observable
stream.subscribe(res => console.log(res));
outputs
{ data: { time: 0 } }
{ data: { time: 1 } }
{ data: { time: 2 } }
{ data: { time: 3 } }
{ data: { time: 4 } }
...
GraphQL Subscriptions are not supported (see issue #27) as everything is treated as subscriptions.
import React from "react";
import ReactDOM from "react-dom";
import "./styles.css";
import { graphql } from "reactive-graphql";
import { makeExecutableSchema } from "graphql-tools";
import { from, interval, of } from "rxjs";
import { map, merge, scan, combineLatest } from "rxjs/operators";
import { componentFromStream } from "@dcos/data-service";
// mocked API clients that return Observables
const oldPosts = from(["my first post", "a second post"]);
const newPosts = interval(3000).pipe(map(v => `Blog Post #${v + 1}`));
const fetchPosts = () =>
oldPosts.pipe(
merge(newPosts),
scan((acc, item) => [...acc, item], [])
);
const votesStore = {};
const fetchVotesForPost = name => of(votesStore[name] || 0);
const schema = makeExecutableSchema({
typeDefs: `
type Post {
id: Int!
title: String!
votes: Int!
}
# the schema allows the following query:
type Query {
posts: [Post]
}
# this schema allows the following mutation:
type Mutation {
upvotePost (
postId: Int!
): Post
}
`,
resolvers: {
Query: {
posts(parent, args, context) {
return fetchPosts().pipe(
map(emittedValue =>
emittedValue.map((value, index) => ({ id: index, title: value }))
)
);
}
},
Post: {
votes(parent, args, context) {
return fetchVotesForPost(parent.title);
}
}
}
});
const query = `
query {
posts {
title
votes
}
}
`;
const postStream = graphql(schema, query);
const PostsList = componentFromStream(propsStream =>
propsStream.pipe(
combineLatest(postStream, (props, result) => {
const {
data: { posts }
} = result;
return posts.map(post => (
<div>
<h3>{post.title}</h3>
</div>
));
})
)
);
function App() {
return (
<div className="App">
<PostsList />
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
Apache 2.0
FAQs
> GraphQL reactive executor
The npm package reactive-graphql receives a total of 30 weekly downloads. As such, reactive-graphql popularity was classified as not popular.
We found that reactive-graphql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.