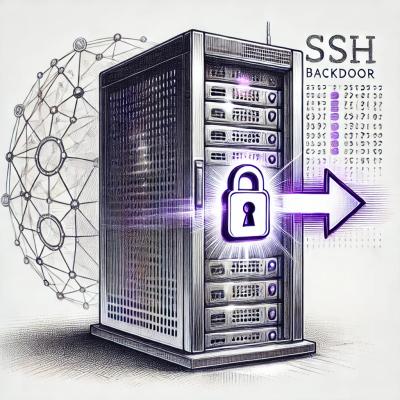
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
reactive-property
Advanced tools
This tiny library abstracts the getter-setter pattern described in Towards Reusable Charts (by Mike Bostock, 2012).
It also adds the ability to react to changes in state.
Install the library by running the command
npm install reactive-property
Require it in your code like this:
var ReactiveProperty = require("reactive-property");
Create your first property.
var a = ReactiveProperty();
Set its value.
a(5);
Get its value.
a();
Listen for changes.
a.on(function(){
console.log("The value of 'a' changed!");
});
Cancel your subscription.
var listener = a.on(function(){
console.log("The value of 'a' changed!");
});
a.off(listener);
Here's some example code from the tests that demonstrates the functionality of this library.
var ReactiveProperty = require("reactive-property");
var assert = require("assert");
describe("Getter-setters", function() {
it("Should construct a property with a default value and get the value.", function () {
var a = ReactiveProperty(5);
assert.equal(a(), 5);
});
it("Should set and get and the value.", function () {
var a = ReactiveProperty();
a(10);
assert.equal(a(), 10);
});
it("Should set the value, overriding the default value.", function () {
var a = ReactiveProperty(5);
a(10);
assert.equal(a(), 10);
});
});
In addition to setting and getting values, you can also listen for changes using the on
function.
it("Should react to changes.", function (done) {
var a = ReactiveProperty();
a.on(function (){
assert.equal(a(), 10);
done();
});
a(10);
});
it("Should react to the default value.", function (done) {
var a = ReactiveProperty(5);
a.on(function (){
assert.equal(a(), 5);
done();
});
});
it("Should not react to no value.", function () {
var a = ReactiveProperty();
var numInvocations = 0;
a.on(function (){ numInvocations++; });
assert.equal(numInvocations, 0);
});
it("Should react synchronously.", function () {
var a = ReactiveProperty();
var numInvocations = 0;
a.on(function (){ numInvocations++; });
assert.equal(numInvocations, 0);
a(10);
assert.equal(numInvocations, 1);
a(15);
assert.equal(numInvocations, 2);
});
it("Should pass the value to the listener as an argument.", function (done) {
var a = ReactiveProperty();
a.on(function (value){
assert.equal(value, 10);
done();
});
a(10);
});
Here's how you can unregister a listener.
it("Should stop reacting to changes.", function () {
var a = ReactiveProperty();
var numInvocations = 0;
var listener = a.on(function (){ numInvocations++; });
a(10);
assert.equal(numInvocations, 1);
a.off(listener);
a(15);
assert.equal(numInvocations, 1);
});
I hope you enjoy this library! Feel free to open GitHub issues if you have any questions, comments or suggestions.
FAQs
A small library for getter-setter functions that react to changes.
The npm package reactive-property receives a total of 1 weekly downloads. As such, reactive-property popularity was classified as not popular.
We found that reactive-property demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.