reactjs-calendar-date-picker
Advanced tools
Comparing version 0.0.2 to 0.0.3
{ | ||
"name": "reactjs-calendar-date-picker", | ||
"author": "Amin Shamouni (https://shamouni.github.io)", | ||
"license": "MIT", | ||
"private": false, | ||
"version": "0.0.2", | ||
"version": "0.0.3", | ||
"type": "module", | ||
@@ -26,2 +28,8 @@ "main": "dist/index.umd.js", | ||
}, | ||
"keywords": [ | ||
"React calendar date picker", | ||
"Calendar", | ||
"React date picker", | ||
"Airbnb calendar" | ||
], | ||
"scripts": { | ||
@@ -36,4 +44,3 @@ "dev": "vite", | ||
"react": "^18.2.0", | ||
"react-dom": "^18.2.0", | ||
"reactjs-calendar-date-picker": "^0.0.1" | ||
"react-dom": "^18.2.0" | ||
}, | ||
@@ -40,0 +47,0 @@ "devDependencies": { |
@@ -1,27 +0,66 @@ | ||
# React + TypeScript + Vite | ||
# React Calendar Date Picker | ||
This template provides a minimal setup to get React working in Vite with HMR and some ESLint rules. | ||
A date picker calendar component built with react that supports date selection and reserved days. | ||
Currently, two official plugins are available: | ||
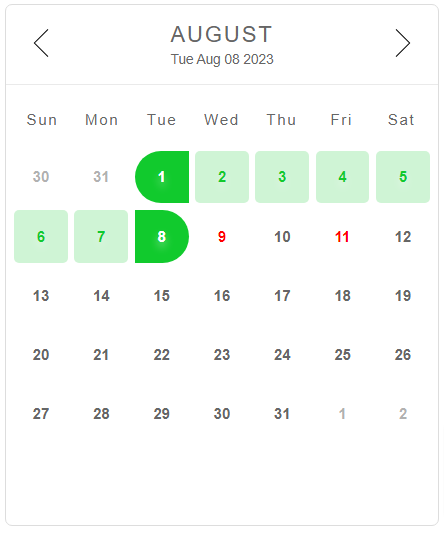 | ||
- [@vitejs/plugin-react](https://github.com/vitejs/vite-plugin-react/blob/main/packages/plugin-react/README.md) uses [Babel](https://babeljs.io/) for Fast Refresh | ||
- [@vitejs/plugin-react-swc](https://github.com/vitejs/vite-plugin-react-swc) uses [SWC](https://swc.rs/) for Fast Refresh | ||
## Installation | ||
## Expanding the ESLint configuration | ||
``` | ||
npm install reactjs-calendar-date-picker | ||
``` | ||
If you are developing a production application, we recommend updating the configuration to enable type aware lint rules: | ||
```js | ||
import Calendar, { TCalDate } from "reactjs-calendar-date-picker"; | ||
``` | ||
- Configure the top-level `parserOptions` property like this: | ||
You need to import the css style | ||
```js | ||
parserOptions: { | ||
ecmaVersion: 'latest', | ||
sourceType: 'module', | ||
project: ['./tsconfig.json', './tsconfig.node.json'], | ||
tsconfigRootDir: __dirname, | ||
}, | ||
import "reactjs-calendar-date-picker/dist/style.css"; | ||
``` | ||
- Replace `plugin:@typescript-eslint/recommended` to `plugin:@typescript-eslint/recommended-type-checked` or `plugin:@typescript-eslint/strict-type-checked` | ||
- Optionally add `plugin:@typescript-eslint/stylistic-type-checked` | ||
- Install [eslint-plugin-react](https://github.com/jsx-eslint/eslint-plugin-react) and add `plugin:react/recommended` & `plugin:react/jsx-runtime` to the `extends` list | ||
## Examples | ||
```js | ||
import { useState } from "react"; | ||
import Calendar, { TCalDate } from "reactjs-calendar-date-picker"; | ||
import "reactjs-calendar-date-picker/dist/style.css"; | ||
type TState = { | ||
fromDate: string; | ||
toDate: string; | ||
}; | ||
function App() { | ||
const [selected, setSelected] = useState<TState>(); | ||
const onChange = (arg: TCalDate) => { | ||
const { from, to } = arg; | ||
const fromDate = new Date(from).toLocaleDateString(); | ||
const toDate = to ? new Date(to).toLocaleDateString() : ""; | ||
setSelected({ fromDate, toDate }); | ||
}; | ||
const { fromDate, toDate } = selected || {}; | ||
return ( | ||
<div className="container"> | ||
<Calendar onChange={onChange} /> | ||
<p className="note"> | ||
{fromDate && toDate && ( | ||
<> | ||
<span>from: {fromDate}</span> | ||
<span>to: {toDate}</span> | ||
</> | ||
)} | ||
</p> | ||
</div> | ||
); | ||
} | ||
export default App; | ||
``` |
No contributors or author data
MaintenancePackage does not specify a list of contributors or an author in package.json.
Found 1 instance in 1 package
No License Found
License(Experimental) License information could not be found.
Found 1 instance in 1 package
204888
2
0
1
67
- Removedreactjs-calendar-date-picker@^0.0.1
- Removedreactjs-calendar-date-picker@0.0.1(transitive)