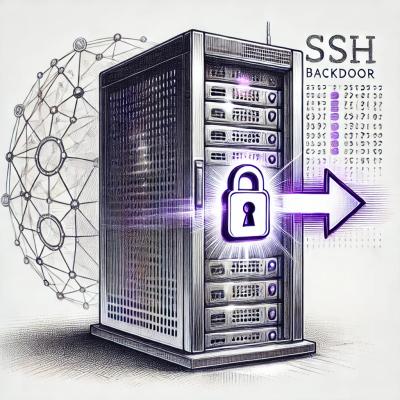
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
read-file-relative
Advanced tools
Read files with path relative to the current module without annoying boilerplate code
The read-file-relative npm package allows you to read files relative to the current module or process's current working directory. It simplifies the process of accessing files without needing to construct absolute paths manually.
Read file relative to the current module
This feature allows you to read a file relative to the current module's location. The `readSync` method reads the file synchronously.
const readFileRelative = require('read-file-relative');
readFileRelative.readSync('./relative/path/to/file.txt');
Read file relative to the current working directory
This feature allows you to read a file relative to the current working directory. The `readSyncFromCwd` method reads the file synchronously.
const readFileRelative = require('read-file-relative');
readFileRelative.readSyncFromCwd('./relative/path/to/file.txt');
Asynchronous file reading
This feature allows you to read a file asynchronously relative to the current module's location. The `read` method reads the file asynchronously and provides a callback for handling the file content or errors.
const readFileRelative = require('read-file-relative');
readFileRelative.read('./relative/path/to/file.txt', (err, content) => {
if (err) throw err;
console.log(content);
});
Asynchronous file reading from current working directory
This feature allows you to read a file asynchronously relative to the current working directory. The `readFromCwd` method reads the file asynchronously and provides a callback for handling the file content or errors.
const readFileRelative = require('read-file-relative');
readFileRelative.readFromCwd('./relative/path/to/file.txt', (err, content) => {
if (err) throw err;
console.log(content);
});
fs-extra is a package that extends the native Node.js fs module with additional methods and promises support. It provides similar functionality for reading files but also includes many other file system operations like copying, moving, and removing files and directories.
The path module is a core Node.js module that provides utilities for working with file and directory paths. While it doesn't directly read files, it helps in constructing absolute paths which can then be used with the fs module to read files.
read-file is a simple package for reading files asynchronously or synchronously. It provides basic file reading capabilities similar to read-file-relative but does not include the relative path convenience methods.
Read files with path relative to the current module without annoying boilerplate code
Well, I've expected @sindresorhus has a module for this, but he didn't.
If you have code like this:
var fs = require('fs');
var path = require('path');
var data = fs.readFileSync(path.join(__dirname, '/my-awesome-file')).toString();
Now you can replace it with:
var readSync = require('read-file-relative').readSync;
var data = readSync('/my-awesome-file');
That's it.
You want a plain buffer instead of string? No problem - just use optional second argument:
var readSync = require('read-file-relative').readSync;
var buffer = readSync('/my-awesome-file', true);
You like it the async way (didn't you :wink:)? Do it this way:
var read = require('read-file-relative').read;
read('/my-awesome-file', function(err, content) {
...
});
You can pass options or encoding like for regular fs.readFile
:
var read = require('read-file-relative').read;
read('/my-awesome-file', 'utf8', function(err, content) {
...
});
BTW, you can just convert given path to absolute:
var toAbsPath = require('read-file-relative').toAbsPath;
var absPath = toAbsPath('/my-awesome-file');
npm install read-file-relative
FAQs
Read files with path relative to the current module without annoying boilerplate code
We found that read-file-relative demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.