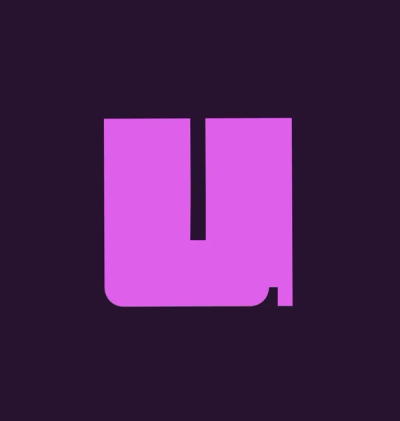
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Terminal emulator component for React!
npm install --save rebash
git clone https://github.com/lpan/rebash
cd rebash
npm install
npm start # server will listen on localhost:8080
import React from 'react';
import ReactDOM from 'react-dom';
import Terminal from 'rebash';
const files = {
'/home/lawrence/test.txt': 'I am a test',
};
const directories = [
'/etc/',
];
const initialPath = ['/home/goose'];
const commands = {
'open-window': args => { alert(args.targets[0]); },
'ghetto-cowsay': args => `cow says: "${args.targets[0]}"`,
};
const MyTerminal = () => (
<Terminal
files={files}
directories={directories}
commands={commands}
initialPath={initialPath}
/>,
document.getElementById('root')
);
export default MyTerminal;
Unix username to display on the terminal.
String
root
lawrence
A list of directories represented by their absolute paths
Array of Strings
[]
['/home', '/etc/nginx/']
Map used to look up files. {'/absolute/path': 'file content'}
note: There is no need to manually create the parent dirs for those files. Rebash will take care of them.
Map of Strings
{}
{'/home/goose.txt': 'Mr. Goose is life', '/lmao.txt': null}
Initial path when the Terminal
is rendered
String
/
'/home/lawrence'
A list of custom commands. See Custom commands for moreinformation.
Map of Functions
{}
{'say-hello': ({targets}) => 'hello ' + targets[0]}
Before you begin, you may want to take a look at src/commands/index.js for all the built-in commands and how they are implemented.
A command
is a JavaScript function which takes 2 parameters, namely args
and
self
.
A typical command will look like this:
const clearThenSayHello = (args, self) => {
self.setState({ visibles: [] });
return `Hello ${args.targets[0]}`;
};
const commads = { 'clear-hello': clearThenSayHello };
// then we pass commands as a prop to the Terminal component
An object consists of three fields: targets
, flags
and fulls
.
When clear-hello -b -dc --all myDir yourDir
is entered, the Rebash argument
parser will produce the following object and pass it to the command function as
args
.
{
targets: ['myDir', 'yourDir'],
flags: ['b', 'c', 'd'],
fulls: ['all'],
}
See src/utils/parseArgs.js for more information
Most of the time, you do not want to mess with self
unless you want to mutate
the internal state of Rebash. self
is just a reference of the instance of the
top level React Component. You can access the component state from self.state
,
mutate the state and trigger the component to rerender with self.setState
,
etc.
see src/Terminal.js for more information.
To print out some text, you can either return a string, or throw an error. The
clear-hello
command will print hello Lawrence
if I type clear-hello lawrence
.
FAQs
Bash interface for you website. Built with React
The npm package rebash receives a total of 3 weekly downloads. As such, rebash popularity was classified as not popular.
We found that rebash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.