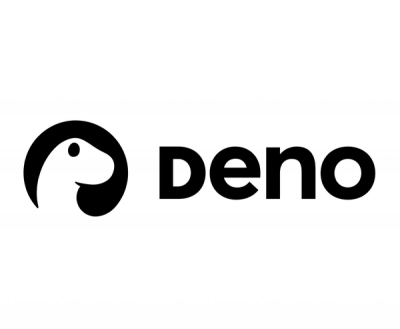
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
reclaim-identity-js
Advanced tools
Reclaim Identity is a JavaScript SDK that provides seamless integration with Reclaim Protocol's authentication system. The SDK manages OAuth 2.0 flows, popup windows, token management, and user session handling.
Reclaim Identity is a JavaScript SDK that provides seamless integration with Reclaim Protocol's authentication system. The SDK manages OAuth 2.0 flows, popup windows, token management, and user session handling.
npm install @reclaim/identity-js
Include directly via CDN:
<script src="https://unpkg.com/@reclaim/identity-js@latest"></script>
const clientId = 'your-client-id';
const clientSecret = 'your-client-secret'
const redirectUri = 'your-redirect-uri';
const reclaimAuth = getReclaimAuth(clientId, clientSecret,redirectUri);
// Sign In
reclaimAuth.signIn()
.then(user => {
console.log('Signed in:', user);
})
.catch(error => {
if (error.message.includes('popup')) {
console.error('Popup was blocked');
} else {
console.error('Authentication error:', error);
}
});
// Sign Out
reclaimAuth.signOut()
.then(() => {
console.log('Signed out successfully');
})
.catch(error => {
console.error('Sign out error:', error);
});
// Get Current User
const user = reclaimAuth.getCurrentUser();
// Listen for Auth State Changes
const unsubscribe = reclaimAuth.onAuthStateChanged(user => {
if (user) {
console.log('User state changed:', user);
} else {
console.log('User signed out');
}
});
// Clean up listener when done
unsubscribe();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Reclaim Identity Demo</title>
</head>
<body>
<div>
<button id="signInBtn">Sign In</button>
<button id="signOutBtn">Sign Out</button>
<div id="userInfo"></div>
</div>
<script src="https://unpkg.com/@reclaim/identity-js@latest"></script>
<script>
const auth = getReclaimAuth(
'your-client-id',
'your-redirect-uri'
);
// Handle Auth State Changes
auth.onAuthStateChanged(user => {
const userInfo = document.getElementById('userInfo');
if (user) {
userInfo.textContent = JSON.stringify(user, null, 2);
} else {
userInfo.textContent = 'No user signed in';
}
});
// Sign In Handler
document.getElementById('signInBtn').addEventListener('click', async () => {
try {
const user = await auth.signIn();
console.log('Signed in successfully:', user);
} catch (error) {
console.error('Sign in failed:', error);
}
});
// Sign Out Handler
document.getElementById('signOutBtn').addEventListener('click', async () => {
try {
await auth.signOut();
console.log('Signed out successfully');
} catch (error) {
console.error('Sign out failed:', error);
}
});
</script>
</body>
</html>
Returns a singleton instance of the ReclaimAuth class. Both parameters are required for initial setup.
Initiates the authentication flow by opening a popup window. Returns a promise that resolves with the user data.
Signs out the current user and clears authentication state.
Returns the currently authenticated user or null if not authenticated.
Subscribes to authentication state changes. Returns an unsubscribe function.
The SDK uses the following defaults:
{
apiBaseUrl: 'https://identity.reclaimprotocol.org',
env: 'production',
popupWidth: 600,
popupHeight: 600
}
The SDK requires browsers with support for:
Common error scenarios:
try {
await auth.signIn();
} catch (error) {
switch (true) {
case error.message.includes('popup'):
// Handle popup blocked
break;
case error.message.includes('code'):
// Handle authorization code error
break;
case error.message.includes('token'):
// Handle token exchange error
break;
default:
// Handle other errors
}
}
class AuthenticationManager {
constructor() {
this.auth = getReclaimAuth(clientId, clientSecret, redirectUri);
this.setupAuthListeners();
}
setupAuthListeners() {
this.unsubscribe = this.auth.onAuthStateChanged(user => {
if (user) {
this.handleSignedIn(user);
} else {
this.handleSignedOut();
}
});
}
async handleSignedIn(user) {
// Handle successful sign in
}
async handleSignedOut() {
// Handle sign out
}
cleanup() {
// Clean up listeners
this.unsubscribe();
}
}
Error Handling
Security
User Experience
Common issues and solutions:
Popup Blocked
Authentication Failures
Security Errors
For issues, questions, or feature requests:
MIT License - see LICENSE for details
Contributions are welcome! Please read our contributing guidelines for details.
FAQs
Reclaim Identity is a JavaScript SDK that provides seamless integration with Reclaim Protocol's authentication system. The SDK manages OAuth 2.0 flows, popup windows, token management, and user session handling.
The npm package reclaim-identity-js receives a total of 6 weekly downloads. As such, reclaim-identity-js popularity was classified as not popular.
We found that reclaim-identity-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.