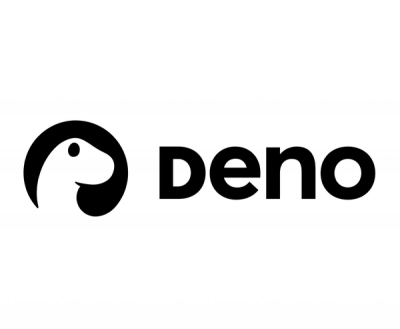
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
recombee-js-api-client
Advanced tools
Client-side js library for easy use of the Recombee recommendation API
A javascript library for easy use of the Recombee recommendation API.
It is intended for usage in browsers and other client side integrations (such as in React Native / NativeScript mobile apps). For Node.js SDK please see this repository.
Documentation of the API can be found at docs.recombee.com.
The library is UMD compatible.
You can download recombee-api-client.min.js and host it at your site, or use a CDN such as jsDelivr CDN:
<script src="https://cdn.jsdelivr.net/gh/recombee/js-api-client@2.3.0/dist/recombee-api-client.min.js"></script>
Use npm
also for React Native / NativeScript applications.
npm install recombee-js-api-client --save
bower install recombee-js-api-client -S
This library allows you to request recommendations and send interactions between users and items (views, bookmarks, purchases ...) to Recombee. It uses the public token for authentication.
It is intentionally not possible to change item catalog (properties of items) with public token, so you should use one of the following ways to send it to Recombee:
// Initialize client with name of your database and PUBLIC token
var client = new recombee.ApiClient('name-of-your-db', '...db-public-token...');
//Interactions take Id of user and Id of item
client.send(new recombee.AddBookmark('user-13434', 'item-256'));
client.send(new recombee.AddCartAddition('user-4395', 'item-129'));
client.send(new recombee.AddDetailView('user-9318', 'item-108'));
client.send(new recombee.AddPurchase('user-7499', 'item-750'));
client.send(new recombee.AddRating('user-3967', 'item-365', 0.5));
client.send(new recombee.SetViewPortion('user-4289', 'item-487', 0.3));
You can recommend items to user or recommend items to item.
It is possible to use callbacks or Promises.
Callback function take two parameters:
null
if request succeeds or Error
object
var callback = function (err, res) {
if(err) {
console.log(err);
// use fallback ...
return;
}
console.log(res.recomms);
}
// Get 5 recommendations for user-13434
client.send(new recombee.RecommendItemsToUser('user-13434', 5), callback);
// Get 5 recommendations related to 'item-365' viewed by 'user-13434'
client.send(new recombee.RecommendItemsToItem('item-356', 'user-13434', 5))
.then(function(res) {
console.log(res.recomms);
})
.catch(function(error) {
console.log(error);
// use fallback ...
});
Recommendation requests accept various optional parameters (see the docs). Following example shows some of them:
client.send(new recombee.RecommendItemsToUser('user-13434', 5,
{
returnProperties: true, // Return properties of the recommended items
includedProperties: ['title', 'img_url', 'url', 'price'], // Use these properties to show
// the recommended items to user
filter: "'title' != null AND 'availability' == \"in stock\"",
// Recommend only items with filled title
// which are in stock
scenario: 'homepage' // Label particular usage
}
), callback);
You can use a script or set a product feed at Recombee web admin. We will set following sample Google Merchant product feed: product_feed_sample.xml. You will see the items in web interface after the feed is processed.
Let's assume we want to show recommendations at product page of pants product-270
to user with id user-1539
. The following HTML+js sample send the detail view of the product by the user and request 3 related items from Recombee:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row">
<h1>Related products</h1>
<div class="col-md-12">
<div class="row" id='relatedProducts'>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/gh/recombee/js-api-client@2.3.0/dist/recombee-api-client.min.js"></script>
<script type="text/javascript">
// A simple function for rendering a box with recommended product
function showProduct(title, description, link, imageLink, price){
return [
'<div class="col-md-4 text-center col-sm-6 col-xs-6">',
' <div class="thumbnail product-box" style="min-height:300px">',
' <img src="' + imageLink +'" alt="" />',
' <div class="caption">',
' <h3><a href="' + link +'">' + title + '</a></h3>',
' <p>Price : <strong>$ ' + price + '</strong> </p>',
' <p>' + description+ '</p>',
' <a href="' + link +'" class="btn btn-primary" role="button">See Details</a></p>',
' </div>',
' </div>',
'</div>'
].join("\n")
}
// Initialize client
var client = new recombee.ApiClient('js-client-example', 'dXx2Jw4VkkYQP1XU4JwBAqGezs8BNzwhogGIRjDHJi39Yj3i0tWyIZ0IhKKw5Ln7');
var itemId = 'product-270';
var userId = 'user-1539'
// Send detail view
client.send(new recombee.AddDetailView(userId, itemId));
// Request recommended items
client.send(new recombee.RecommendItemsToItem(itemId, userId, 3,
{
returnProperties: true,
includedProperties: ['title', 'description', 'link', 'image_link', 'price'],
filter: "'title' != null AND 'availability' == \"in stock\"",
scenario: 'related_items'
}),
(err, resp) => {
if(err) {
console.log("Could not load recomms: ", err);
return;
}
// Show recommendations
var recomms_html = resp.recomms.map(r => r.values).
map(vals => showProduct(vals['title'], vals['description'],
vals['link'], vals['image_link'], vals['price']));
document.getElementById("relatedProducts").innerHTML = recomms_html.join("\n");
}
);
</script>
</body>
</html>
You should see something like this:
Please notice how the properties returned by returnProperties
&includedProperties
were used to show titles, images, descriptions and URLs.
In order to achieve personalization, you need a unique identifier for each user. An easy way can be using Google Analytics for this purpose. The example then becomes:
ga('create', 'UA-XXXXX-Y', 'auto'); // Create a tracker if you don't have one
// Replace the UA-XXXXX-Y with your UA code from Google Analytics.
var client = new recombee.ApiClient('js-client-example', 'dXx2Jw4VkkYQP1XU4JwBAqGezs8BNzwhogGIRjDHJi39Yj3i0tWyIZ0IhKKw5Ln7');
ga(function(tracker) {
var userId = tracker.get('clientId'); // Get id from GA
client.send(new recombee.RecommendItemsToUser(userId, 3,
{
returnProperties: true,
includedProperties: ['title', 'description', 'link', 'image_link', 'price'],
filter: "'title' != null AND 'availability' == \"in stock\"",
scenario: 'homepage'
}),
(err, resp) => { ... }
);
});
This time RecommendItemsToUser is used - it can be used for example at your homepage.
FAQs
Client-side js library for easy use of the Recombee recommendation API
The npm package recombee-js-api-client receives a total of 2,463 weekly downloads. As such, recombee-js-api-client popularity was classified as popular.
We found that recombee-js-api-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.