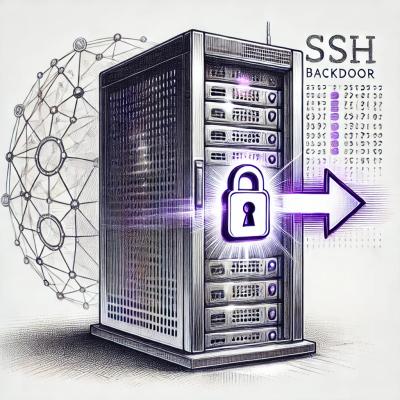
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
RecordRTC is a server-less (entire client-side) JavaScript library that can be used to record WebRTC audio/video media streams. It supports cross-browser audio/video recording.
WebRTC JavaScript Library for Audio+Video+Screen+Canvas (2D+3D animation) Recording
Chrome Extension or Dozens of Simple-Demos and it is Open-Sourced and has API documentation
A demo using promises:
let stream = await navigator.mediaDevices.getUserMedia({video: true, audio: true});
let recorder = new RecordRTCPromisesHandler(stream, {
type: 'video'
});
recorder.startRecording();
const sleep = m => new Promise(r => setTimeout(r, m));
await sleep(3000);
await recorder.stopRecording();
let blob = await recorder.getBlob();
invokeSaveAsDialog(blob);
A demo using normal coding:
navigator.mediaDevices.getUserMedia({
video: true,
audio: true
}).then(async function(stream) {
let recorder = RecordRTC(stream, {
type: 'video'
});
recorder.startRecording();
const sleep = m => new Promise(r => setTimeout(r, m));
await sleep(3000);
recorder.stopRecording(function() {
let blob = recorder.getBlob();
invokeSaveAsDialog(blob);
});
});
Browser | Operating System | Features |
---|---|---|
Google Chrome | Windows + macOS + Ubuntu + Android | audio + video + screen |
Firefox | Windows + macOS + Ubuntu + Android | audio + video + screen |
Opera | Windows + macOS + Ubuntu + Android | audio + video + screen |
Edge (new) | Windows (7 or 8 or 10) and MacOSX | audio + video + screen |
Safari | macOS + iOS (iPhone/iPad) | audio + video |
Browser | Video | Audio |
---|---|---|
Chrome | VP8, VP9, H264, MKV | OPUS/VORBIS, PCM |
Opera | VP8, VP9, H264, MKV | OPUS/VORBIS, PCM |
Firefox | VP8, H264 | OPUS/VORBIS, PCM |
Safari | VP8 | OPUS/VORBIS, PCM |
Edge (new) | VP8, VP9, H264, MKV | OPUS/VORBIS, PCM |
<!-- recommended -->
<script src="https://www.WebRTC-Experiment.com/RecordRTC.js"></script>
<!-- use 5.6.0 or any other version on cdnjs -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/RecordRTC/5.6.0/RecordRTC.js"></script>
<!-- NPM i.e. "npm install recordrtc" -->
<script src="node_modules/recordrtc/RecordRTC.js"></script>
<!-- bower -->
<script src="bower_components/recordrtc/RecordRTC.js"></script>
const recorder = RecordRTC(stream, {
// audio, video, canvas, gif
type: 'video',
// audio/webm
// video/webm;codecs=vp9
// video/webm;codecs=vp8
// video/webm;codecs=h264
// video/x-matroska;codecs=avc1
// video/mpeg -- NOT supported by any browser, yet
// video/mp4 -- NOT supported by any browser, yet
// audio/wav
// audio/ogg -- ONLY Firefox
// demo: simple-demos/isTypeSupported.html
mimeType: 'video/webm',
// MediaStreamRecorder, StereoAudioRecorder, WebAssemblyRecorder
// CanvasRecorder, GifRecorder, WhammyRecorder
recorderType: MediaStreamRecorder,
// disable logs
disableLogs: true,
// get intervals based blobs
// value in milliseconds
timeSlice: 1000,
// requires timeSlice above
// returns blob via callback function
ondataavailable: function(blob) {},
// auto stop recording if camera stops
checkForInactiveTracks: false,
// requires timeSlice above
onTimeStamp: function(timestamp) {},
// both for audio and video tracks
bitsPerSecond: 128000,
// only for audio track
audioBitsPerSecond: 128000,
// only for video track
videoBitsPerSecond: 128000,
// used by CanvasRecorder and WhammyRecorder
// it is kind of a "frameRate"
frameInterval: 90,
// if you are recording multiple streams into single file
// this helps you see what is being recorded
previewStream: function(stream) {},
// used by CanvasRecorder and WhammyRecorder
// you can pass {width:640, height: 480} as well
video: HTMLVideoElement,
// used by CanvasRecorder and WhammyRecorder
canvas: {
width: 640,
height: 480
},
// used by StereoAudioRecorder
// the range 22050 to 96000.
sampleRate: 96000,
// used by StereoAudioRecorder
// the range 22050 to 96000.
// let us force 16khz recording:
desiredSampRate: 16000,
// used by StereoAudioRecorder
// Legal values are (256, 512, 1024, 2048, 4096, 8192, 16384).
bufferSize: 16384,
// used by StereoAudioRecorder
// 1 or 2
numberOfAudioChannels: 2,
// used by WebAssemblyRecorder
frameRate: 30,
// used by WebAssemblyRecorder
bitrate: 128000,
// used by MultiStreamRecorder - to access HTMLCanvasElement
elementClass: 'multi-streams-mixer'
});
MediaStream parameter accepts following values:
let recorder = RecordRTC(MediaStream || HTMLCanvasElement || HTMLVideoElement || HTMLElement, {});
RecordRTC.prototype = {
// start the recording
startRecording: function() {},
// stop the recording
// getBlob inside callback function
stopRecording: function(blobURL) {},
// pause the recording
pauseRecording: function() {},
// resume the recording
resumeRecording: function() {},
// auto stop recording after specific duration
setRecordingDuration: function() {},
// reset recorder states and remove the data
reset: function() {},
// invoke save as dialog
save: function(fileName) {},
// returns recorded Blob
getBlob: function() {},
// returns Blob-URL
toURL: function() {},
// returns Data-URL
getDataURL: function(dataURL) {},
// returns internal recorder
getInternalRecorder: function() {},
// initialize the recorder [deprecated]
initRecorder: function() {},
// fired if recorder's state changes
onStateChanged: function(state) {},
// write recorded blob into indexed-db storage
writeToDisk: function(audio: Blob, video: Blob, gif: Blob) {},
// get recorded blob from indexded-db storage
getFromDisk: function(dataURL, type) {},
// [deprecated]
setAdvertisementArray: function([webp1, webp2]) {},
// [deprecated] clear recorded data
clearRecordedData: function() {},
// clear memory; clear everything
destroy: function() {},
// get recorder's state
getState: function() {},
// [readonly] property: recorder's state
state: string,
// recorded blob [readonly] property
blob: Blob,
// [readonly] array buffer; useful only for StereoAudioRecorder
buffer: ArrayBuffer,
// RecordRTC version [readonly]
version: string,
// [readonly] useful only for StereoAudioRecorder
bufferSize: integer,
// [readonly] useful only for StereoAudioRecorder
sampleRate: integer
}
Please check documentation here: https://recordrtc.org/
// "bytesToSize" returns human-readable size (in MB or GB)
let size = bytesToSize(recorder.getBlob().size);
// to fix video seeking issues
getSeekableBlob(recorder.getBlob(), function(seekableBlob) {
invokeSaveAsDialog(seekableBlob);
});
// this function invokes save-as dialog
invokeSaveAsDialog(recorder.getBlob(), 'video.webm');
// use these global variables to detect browser
let browserInfo = {isSafari, isChrome, isFirefox, isEdge, isOpera};
// use this to store blobs into IndexedDB storage
DiskStorage = {
init: function() {},
Fetch: function({audioBlob: Blob, videoBlob: Blob, gifBlob: Blob}) {},
Store: function({audioBlob: Blob, videoBlob: Blob, gifBlob: Blob}) {},
onError: function() {},
dataStoreName: function() {}
};
<video>.muted=true
and <video>.volume=0
audio: {echoCancellation:true}
on getUserMediamuazkh => gmail
Library | Usage |
---|---|
Recorderjs | StereoAudioRecorder |
webm-wasm | WebAssemblyRecorder |
jsGif | GifRecorder |
whammy | WhammyRecorder |
Framework | Github | Article |
---|---|---|
Angular2 | github | article |
React.js | github | article |
Video.js | github | None |
Meteor | github | None |
RecordRTC.js is released under MIT license . Copyright (c) Muaz Khan.
FAQs
RecordRTC is a server-less (entire client-side) JavaScript library that can be used to record WebRTC audio/video media streams. It supports cross-browser audio/video recording.
We found that recordrtc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.