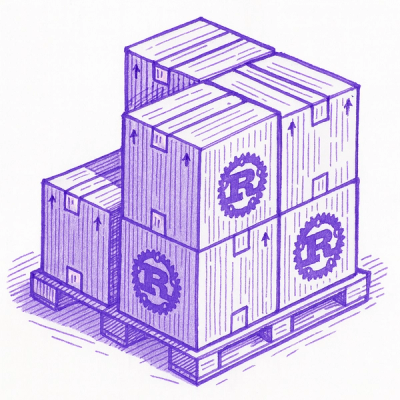
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
redis-simple-rpc
Advanced tools
This is a very simple rpc client / server using Redis lists. It suports async callbacks and streaming.
Both the client and worker for node.js use proxy objects, making them as easy to use as local functions.
Defining a worker:
redisConnection = {
host: '127.0.0.1',
port: 1234
options: {}
};
new Worker(namespace, redisConnection);
Example:
var Worker = require('redis-simple-rpc').Worker,
calc = new Worker('calc');
calc.add = function (a, b, cb) {
cb(null, a + b);
};
Defining a client:
new Client(namespace, clientId, redisConnection, timeout);
Example:
var Client = require('redis-simple-rpc').Client,
calc = new Client('calc');
calc.add(1, 2, function (err, answer) {
if (err) {
throw err;
}
assert.equal(1 + 2, answer);
});
The node redis driver will throw an exception if a client does not have any listeners on error
. Each client or worker has two redis clients.
var Client = require('redis-simple-rpc').Client,
calc = new Client('calc'),
logError;
logError = function () {
return console.error.apply(console, arguments);
};
calc.clients.sender.on('error', logError);
calc.clients.receiver.on('error', logError);
FAQs
Simple RPC with Redis lists
The npm package redis-simple-rpc receives a total of 1 weekly downloads. As such, redis-simple-rpc popularity was classified as not popular.
We found that redis-simple-rpc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.