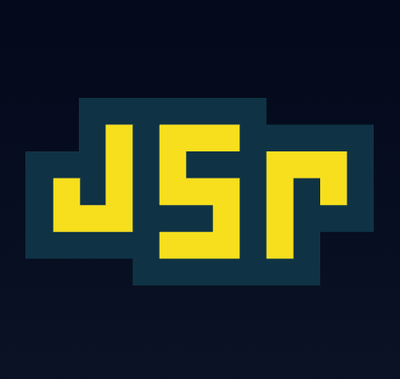
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The npm package 'redis' is a Node.js client for Redis, a fast, open-source, in-memory key-value data store for use as a database, cache, message broker, and queue. The package allows Node.js applications to interact with Redis servers using an asynchronous, event-driven model.
Connecting to Redis
This code sample demonstrates how to connect to a Redis server using the redis npm package. It requires the package, creates a client, and listens for the 'connect' event to confirm the connection.
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis');
});
Setting and Getting Data
This code sample shows how to set a key-value pair in Redis and then retrieve the value associated with a key. The 'redis.print' callback is used to output the result of the 'set' operation.
client.set('key', 'value', redis.print);
client.get('key', function(err, reply) {
console.log(reply); // prints 'value'
});
Working with Lists
This code sample illustrates how to work with Redis lists by pushing values to the end of a list and then retrieving the entire list.
client.rpush(['list', 'value1', 'value2'], redis.print);
client.lrange('list', 0, -1, function(err, reply) {
console.log(reply); // prints ['value1', 'value2']
});
Publish/Subscribe
This code sample demonstrates the publish/subscribe capabilities of Redis. It creates a subscriber client that listens for messages on a channel and a publisher client that publishes a message to that channel.
const subscriber = redis.createClient();
const publisher = redis.createClient();
subscriber.on('message', function(channel, message) {
console.log('Message: ' + message + ' on channel: ' + channel);
});
subscriber.subscribe('notification');
publisher.publish('notification', 'Hello, World!');
Transactions
This code sample shows how to use Redis transactions to execute multiple commands atomically using the 'multi' and 'exec' methods.
client.multi()
.set('key', 'value')
.incr('counter')
.exec(function(err, replies) {
console.log(replies); // prints results of all commands
});
ioredis is a robust, performance-focused, and full-featured Redis client for Node.js. It supports Redis Cluster, Sentinel, pipelining, Lua scripting, and more. Compared to the 'redis' package, ioredis offers a more modern interface with Promises support and better performance for certain operations.
node-redis is another Redis client for Node.js that is designed to be easy to use. It may not have as many features as 'redis' or 'ioredis', but it provides a straightforward way to interact with Redis servers for simple use cases.
redis-mock is a library that simulates a Redis server for testing purposes. It implements most of the Redis commands and can be used as a drop-in replacement for the 'redis' package during testing, without the need for an actual Redis server.
import { createClient } from 'redis';
const client = await createClient()
.on('error', err => console.log('Redis Client Error', err))
.connect();
await client.set('key', 'value');
const value = await client.get('key');
await client.close();
:warning: You MUST listen to
error
events. If a client doesn't have at least oneerror
listener registered and anerror
occurs, that error will be thrown and the Node.js process will exit. See theEventEmitter
docs for more details.
The above code connects to localhost on port 6379. To connect to a different host or port, use a connection string in the format redis[s]://[[username][:password]@][host][:port][/db-number]
:
createClient({
url: 'redis://alice:foobared@awesome.redis.server:6380'
});
You can also use discrete parameters, UNIX sockets, and even TLS to connect. Details can be found in the client configuration guide.
There is built-in support for all of the out-of-the-box Redis commands. They are exposed using the raw Redis command names (HSET
, HGETALL
, etc.) and a friendlier camel-cased version (hSet
, hGetAll
, etc.):
// raw Redis commands
await client.HSET('key', 'field', 'value');
await client.HGETALL('key');
// friendly JavaScript commands
await client.hSet('key', 'field', 'value');
await client.hGetAll('key');
Modifiers to commands are specified using a JavaScript object:
await client.set('key', 'value', {
expiration: {
type: 'EX',
value: 10
},
condition: 'NX'
});
NOTE: command modifiers that change the reply type (e.g.
WITHSCORES
forZDIFF
) are exposed as separate commands (e.g.ZDIFF_WITHSCORES
/zDiffWithScores
).
Replies will be mapped to useful data structures:
await client.hGetAll('key'); // { field1: 'value1', field2: 'value2' }
await client.hVals('key'); // ['value1', 'value2']
NOTE: you can change the default type mapping. See the Type Mapping documentation for more information.
If you want to run commands and/or use arguments that Node Redis doesn't know about (yet!) use .sendCommand()
:
await client.sendCommand(['SET', 'key', 'value', 'EX', '10', 'NX']); // 'OK'
await client.sendCommand(['HGETALL', 'key']); // ['key1', 'field1', 'key2', 'field2']
.close()
Gracefully close a client's connection to Redis. Wait for commands in process, but reject any new commands.
const [ping, get] = await Promise.all([
client.ping(),
client.get('key'),
client.close()
]); // ['PONG', null]
try {
await client.get('key');
} catch (err) {
// ClientClosedError
}
.close()
is just like.quit()
which was depreacted v5. See the relevant section in the migration guide for more information.
.destroy()
Forcibly close a client's connection to Redis.
try {
const promise = Promise.all([
client.ping(),
client.get('key')
]);
client.destroy();
await promise;
} catch (err) {
// DisconnectsClientError
}
try {
await client.get('key');
} catch (err) {
// ClientClosedError
}
.destroy()
is just like.disconnect()
which was depreated in v5. See the relevant section in the migration guide for more information.
Node Redis will automatically pipeline requests that are made during the same "tick".
client.set('Tm9kZSBSZWRpcw==', 'users:1');
client.sAdd('users:1:tokens', 'Tm9kZSBSZWRpcw==');
Of course, if you don't do something with your Promises you're certain to get unhandled Promise exceptions. To take advantage of auto-pipelining and handle your Promises, use Promise.all()
.
await Promise.all([
client.set('Tm9kZSBSZWRpcw==', 'users:1'),
client.sAdd('users:1:tokens', 'Tm9kZSBSZWRpcw==')
]);
To client exposes 2 boolean
s that track the client state:
isOpen
- the client is either connecting or connected.isReady
- the client is connected and ready to sendThe client extends EventEmitter
and emits the following events:
Name | When | Listener arguments |
---|---|---|
connect | Initiating a connection to the server | No arguments |
ready | Client is ready to use | No arguments |
end | Connection has been closed (via .quit() or .disconnect() ) | No arguments |
error | An error has occurred—usually a network issue such as "Socket closed unexpectedly" | (error: Error) |
reconnecting | Client is trying to reconnect to the server | No arguments |
sharded-channel-moved | See here | See here |
:warning: You MUST listen to
error
events. If a client doesn't have at least oneerror
listener registered and anerror
occurs, that error will be thrown and the Node.js process will exit. See theEventEmitter
docs for more details.
MULTI
/EXEC
).Node Redis is supported with the following versions of Redis:
Version | Supported |
---|---|
7.2.z | :heavy_check_mark: |
7.0.z | :heavy_check_mark: |
6.2.z | :heavy_check_mark: |
6.0.z | :heavy_check_mark: |
5.0.z | :heavy_check_mark: |
< 5.0 | :x: |
Node Redis should work with older versions of Redis, but it is not fully tested and we cannot offer support.
If you'd like to contribute, check out the contributing guide.
Thank you to all the people who already contributed to Node Redis!
This repository is licensed under the "MIT" license. See LICENSE.
FAQs
A modern, high performance Redis client
We found that redis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.