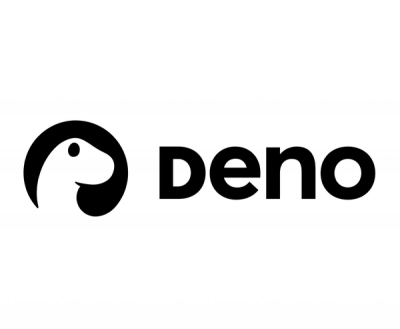
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Type-safe, DRY and OO redux. Implemented with typescript.
npm install --save redux-app
@component
class App {
counter = new Counter();
}
@component
class Counter {
value = 0;
increment() {
this.value = value + 1; // <--- see Important Notice below
}
}
const app = new ReduxApp(new App(), devToolsEnhancer(undefined));
console.log(app.root.counter.value); // 0
console.log(app.store.getState()); // { counter: { value: 0 } }
app.root.counter.increment(); // will dispatch COUNTER.INCREMENT redux action
console.log(app.root.counter.value); // 1
console.log(app.store.getState()); // { counter: { value: 1 } }
You should not mutate the object properties but rather assign them with new values.
That's why we write this.value = value + 1
and not this.value++
.
More examples can be found here redux-app-examples.
For each component
decorated class the library generates an underlying Component
object that holds the same properties and method.
The new Component object has it's prototype patched and all of it's methods replaced with dispatch() calls.
The generated Component also has a hidden 'REDUCER' property which is later on used by redux store. The 'REDUCER' property itself is
generated from the original object methods, replacing all 'this' values with the current state from the store on each call (using
Object.assign and Function.prototype.call).
Async actions and side effects are handled in redux-app by using either the sequence
decorator or the noDispatch
.
Both decorators does exactly the same and are actually aliases of the same underlying function. What they do is
to tell redux-app that the decorated method is a plain old javascript method and that it should not be patched (about
the patch process see How it works). So, to conclude, what these decorators actually do is to tell
redux-app to do nothing special with the method.
Usage:
working example can be found on the redux-app-examples page
@component
class MyComponent {
public setStatus(newStatus: string) { // <--- Not decorated. Will dispatch SET_STATUS action.
this.status = newStatus;
}
@sequence
public async fetchImage() {
// dispatch an action
this.setStatus('Fetching...');
// do async stuff
var response = await fetch('fetch something from somewhere');
var responseBody = await response.json();
// dispatch another action
this.setStatus('Adding unnecessary delay...');
// more async...
setTimeout(() => {
// more dispatch
this.setStatus('I am done.');
}, 2000);
}
@noDispatch
public doNothing() {
console.log('I am a plain old method. Nothing special here.');
}
}
The rule of the withId
decorator is double. From one hand, it enables the co-existence of two (or more) instances of the same component,
each with it's own separate state. From the other hand, it is used to keep two separate components in sync. The 'id' argument of the decorator
can be anything (string, number, object, etc.).
Example:
working example can be found on the redux-app-examples page
@component
export class App {
@withId('SyncMe')
public counter1 = new CounterComponent(); // <-- this counter is in sync with counter2
@withId('SyncMe')
public counter2 = new CounterComponent(); // <-- this counter is in sync with counter1
@withId(123)
public counter3 = new CounterComponent(); // <-- manual set ID
// this counter is not synced with the others
@withId()
public counter4 = new CounterComponent(); // <-- auto generated unique ID (unique within the scope of the application)
// this counter also has it's own unique state
}
You can supply the following options to the component
decorator.
class SchemaOptions {
/**
* Add the class name of the object that holds the action to the action name.
* Format: <class name>.<action name>.
* Default value: true.
*/
public actionNamespace?: boolean;
/**
* Use redux style action names. For instance, if a component defines a
* method called 'incrementCounter' the matching action name will be
* 'INCREMENT_COUNTER'.
* Default value: true.
*/
public uppercaseActions?: boolean;
/**
* By default each component is assigned (with some optimizations) with it's
* relevant sub state on each store change. Set this to false to disable
* this updating process. The store's state will still be updated as usual
* and can always be retrieved using store.getState().
* Default value: true.
*/
public updateState?: boolean;
}
Usage:
@component({ uppercaseActions: false })
class Counter {
value = 0;
increment() { // <-- Will now dispatch 'Counter.increment' instead of 'COUNTER.INCREMENT'. Everything else still works the same, no further change required.
this.value = value + 1;
}
}
Available global options:
class GlobalOptions {
logLevel: LogLevel;
/**
* Global defaults.
* Options supplied explicitly via the decorator will override options specified here.
*/
schema: SchemaOptions;
}
enum LogLevel {
/**
* Emit no logs
*/
None = 0,
Verbose = 1,
Debug = 2,
/**
* Emit no logs (same as None)
*/
Silent = 10
}
Usage:
ReduxApp.options.logLevel = LogLevel.Debug;
FAQs
Type-safe, DRY and OO redux. Implemented with typescript.
The npm package redux-app receives a total of 13 weekly downloads. As such, redux-app popularity was classified as not popular.
We found that redux-app demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.