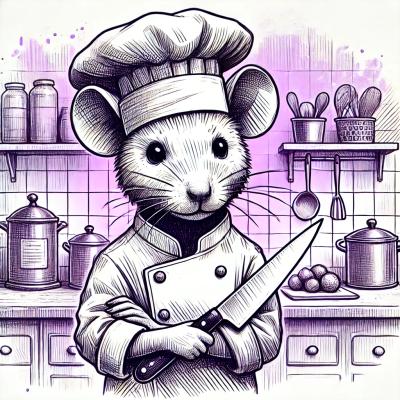
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
redux-devtools-extension
Advanced tools
The redux-devtools-extension package is a powerful tool for debugging Redux applications. It provides a set of tools to help developers track state changes, actions, and store updates in real-time, making it easier to understand and debug the state management in their applications.
Time Travel Debugging
Time travel debugging allows developers to go back and forth through the state changes, making it easier to identify where things went wrong. The code sample shows how to integrate the Redux DevTools Extension with a Redux store using the `composeWithDevTools` function.
import { createStore } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(reducer, composeWithDevTools());
Action Logging
Action logging provides a detailed log of all actions dispatched to the store, along with the state before and after each action. The code sample demonstrates dispatching an action and how it would be logged by the Redux DevTools Extension.
import { createStore } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(reducer, composeWithDevTools());
store.dispatch({ type: 'INCREMENT' });
State Inspection
State inspection allows developers to view the current state of the Redux store at any point in time. The code sample shows how to create a store and log its current state, which can be inspected using the Redux DevTools Extension.
import { createStore } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(reducer, composeWithDevTools());
console.log(store.getState());
redux-logger is a middleware for Redux that logs actions and state changes to the console. It provides a simpler, more lightweight alternative to redux-devtools-extension, focusing primarily on logging rather than providing a full suite of debugging tools.
redux-saga-devtools is a tool specifically designed for debugging Redux Saga middleware. It provides insights into the effects and actions managed by sagas, making it easier to debug complex asynchronous flows. While it is more specialized than redux-devtools-extension, it offers deeper integration with Redux Saga.
Install:
npm install --save redux-devtools-extension
and use like that:
import { createStore, applyMiddleware } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(
reducer,
composeWithDevTools(
applyMiddleware(...middleware)
// other store enhancers if any
)
);
or if needed to apply extension’s options:
import { createStore, applyMiddleware } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
const composeEnhancers = composeWithDevTools({
// Specify here name, actionsBlacklist, actionsCreators and other options
});
const store = createStore(
reducer,
composeEnhancers(
applyMiddleware(...middleware)
// other store enhancers if any
)
);
There’re just few lines of code. If you don’t want to allow the extension in production, just use ‘redux-devtools-extension/developmentOnly’ instead of ‘redux-devtools-extension’.
MIT
FAQs
Wrappers for Redux DevTools Extension.
The npm package redux-devtools-extension receives a total of 549,998 weekly downloads. As such, redux-devtools-extension popularity was classified as popular.
We found that redux-devtools-extension demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.