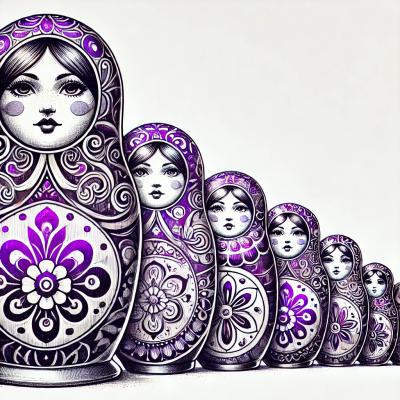
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
redux-immutable
Advanced tools
redux-immutable is used to create an equivalent function of Redux combineReducers that works with Immutable.js state.
The redux-immutable package is designed to work with Redux and Immutable.js, providing a way to use an Immutable.js Map as the root of your Redux state. This allows you to leverage the benefits of Immutable.js, such as structural sharing and efficient updates, while still using Redux for state management.
combineReducers
The combineReducers function from redux-immutable allows you to combine multiple reducers into a single reducer function, with the root state being an Immutable.js Map. This is similar to Redux's combineReducers but works with Immutable.js.
const { combineReducers } = require('redux-immutable');
const { Map } = require('immutable');
const { createStore } = require('redux');
const fooReducer = (state = 'foo', action) => state;
const barReducer = (state = 'bar', action) => state;
const rootReducer = combineReducers({
foo: fooReducer,
bar: barReducer
});
const initialState = Map();
const store = createStore(rootReducer, initialState);
console.log(store.getState().toJS());
getIn
The getIn method allows you to access nested properties within the Immutable.js Map. This is useful for deeply nested state structures, providing a convenient way to retrieve values.
const { combineReducers } = require('redux-immutable');
const { Map } = require('immutable');
const { createStore } = require('redux');
const fooReducer = (state = Map({ value: 'foo' }), action) => state;
const barReducer = (state = Map({ value: 'bar' }), action) => state;
const rootReducer = combineReducers({
foo: fooReducer,
bar: barReducer
});
const initialState = Map();
const store = createStore(rootReducer, initialState);
console.log(store.getState().getIn(['foo', 'value']));
Redux is a predictable state container for JavaScript apps. While it does not natively support Immutable.js, it can be used with Immutable.js by writing custom reducers and selectors. redux-immutable simplifies this process by providing utilities that work directly with Immutable.js.
Immutable.js provides persistent immutable data structures. It can be used with Redux to manage state immutably, but it requires additional boilerplate to integrate with Redux. redux-immutable bridges this gap by providing seamless integration.
Seamless-immutable is another library for immutable data structures. It is simpler and lighter than Immutable.js but offers fewer features. It can be used with Redux, but like Immutable.js, it requires additional setup compared to redux-immutable.
redux-immutable
redux-immutable
is used to create an equivalent function of Redux combineReducers
that works with Immutable.js state.
When Redux createStore
reducer
is created using redux-immutable
then initialState
must be an instance of Immutable.Collection
.
When createStore
is invoked with initialState
that is an instance of Immutable.Collection
further invocation of reducer will produce an error:
The initialState argument passed to createStore has unexpected type of "Object". Expected argument to be an object with the following keys: "data"
This is because Redux combineReducers
treats state
object as a plain JavaScript object.
combineReducers
created using redux-immutable
uses Immutable.js API to iterate the state.
Create a store with initialState
set to an instance of Immutable.Collection
:
import {
combineReducers
} from 'redux-immutable';
import {
createStore
} from 'redux';
const initialState = Immutable.Map();
const rootReducer = combineReducers({});
const store = createStore(rootReducer, initialState);
By default, if state
is undefined
, rootReducer(state, action)
is called with state = Immutable.Map()
. A different default function can be provided as the second parameter to combineReducers(reducers, getDefaultState)
, for example:
const StateRecord = Immutable.Record({
foo: 'bar'
});
const rootReducer = combineReducers({foo: fooReducer}, StateRecord);
// rootReducer now has signature of rootReducer(state = StateRecord(), action)
// state now must always have 'foo' property with 'bar' as its default value
When using Immutable.Record
it is possible to delegate default values to child reducers:
const StateRecord = Immutable.Record({
foo: undefined
});
const rootReducer = combineReducers({foo: fooReducer}, StateRecord);
// state now must always have 'foo' property with its default value returned from fooReducer(undefined, action)
In general, getDefaultState
function must return an instance of Immutable.Record
or Immutable.Collection
that implements get
, set
and withMutations
methods. Such collections are List
, Map
and OrderedMap
.
react-router-redux
react-router-redux
routeReducer
does not work with Immutable.js. You need to use a custom reducer:
import Immutable from 'immutable';
import {
LOCATION_CHANGE
} from 'react-router-redux';
const initialState = Immutable.fromJS({
locationBeforeTransitions: null
});
export default (state = initialState, action) => {
if (action.type === LOCATION_CHANGE) {
return state.set('locationBeforeTransitions', action.payload);
}
return state;
};
Pass a selector to access the payload state and convert it to a JavaScript object via the selectLocationState
option on syncHistoryWithStore
:
import {
browserHistory
} from 'react-router';
import {
syncHistoryWithStore
} from 'react-router-redux';
const history = syncHistoryWithStore(browserHistory, store, {
selectLocationState (state) {
return state.get('routing').toJS();
}
});
The 'routing'
path depends on the rootReducer
definition. This example assumes that routeReducer
is made available under routing
property of the rootReducer
.
FAQs
redux-immutable is used to create an equivalent function of Redux combineReducers that works with Immutable.js state.
The npm package redux-immutable receives a total of 389,895 weekly downloads. As such, redux-immutable popularity was classified as popular.
We found that redux-immutable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.