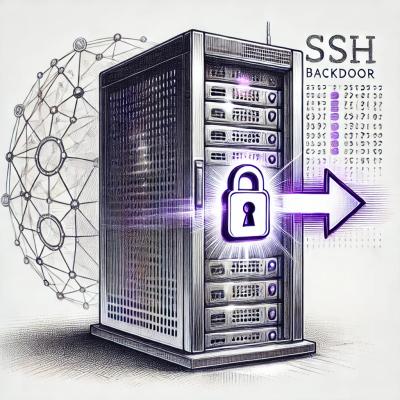
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Flexible, powerful and modern library for creating the running horizontal blocks effect, also known as ticker or the «marquee effect».
Flexible, powerful and modern library for creating the running horizontal blocks effect, also known as ticker or the «marquee effect».
The library uses GSAP, IntersectionObserver and ResizeObserver to achieve the best performance results.
⚠️ Notice: This library is currently in beta.
GSAP v3 (https://greensock.com/gsap/)
Reeller requires GSAP library to work.
npm install gsap --save
npm install reeller --save
Import GSAP, Reeller and initialize it:
import Reeller from 'reeller';
import gsap from 'gsap';
Reeller.registerGSAP(gsap);
const reeller = new Reeller({
container: '.my-reel',
wrapper: '.my-reel-wrap',
itemSelector: '.my-reel-item',
speed: 10,
});
If you don't want to include Reeller files in your project, you can use library from CDN:
<script src="https://unpkg.com/reeller@0/dist/reeller.min.js"></script>
Reeller requires GSAP to work. You need to import it above the Reeller if you didn't have it before:
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.10.4/gsap.min.js"></script>
<script src="https://unpkg.com/reeller@0/dist/reeller.min.js"></script>
<script>
var reeller = new Reeller.Reeller({
container: '.my-reel',
wrapper: '.my-reel-wrap',
itemSelector: '.my-reel-item',
speed: 10,
});
</script>
Note: All modules (Reeller, Filler, ScrollerPlugin) will be imported into Reeller
namespace when using from CDN, so you must use a prefix.
You can configure Reeller via options.
The following options with defaults is available:
const reeller = new Reeller({
container: null,
wrapper: null,
itemSelector: null,
cloneClassName: '-clone',
speed: 10,
ease: 'none',
initialSeek: 10,
loop: true,
paused: true,
reversed: false,
autoStop: true,
autoUpdate: true,
clonesOverflow: true,
clonesFinish: false,
clonesMin: 0,
});
Option | Type | Default | Description |
---|---|---|---|
container | string | HTMLElement | null | Required. Container element or selector. |
wrapper | string | HTMLElement | null | Required. Inner element or selector. |
itemSelector | string | null | Required. Items CSS selector. |
cloneClassName | string | -clone | Class name of the new clones. |
speed | number | 10 | Movement speed. |
ease | string | 'none' | Timing function. See gsap easing. |
initialSeek | number | 10 | Initial seek of timeline. |
loop | boolean | true | Loop movement. |
pause | boolean | true | Initialize in paused mode. |
reversed | boolean | false | Reverse mode. |
autoStop | boolean | true | Use IntersectionObserver to auto stop movement. |
autoUpdate | boolean | true | Use ResizeObserver to auto update clones number. |
clonesOverflow | boolean | true | Create artificial overflow with clones. |
clonesFinish | boolean | false | Bring the cycle of clones to an end. |
clonesMin | boolean | 0 | Minimum number of clones. |
plugins | Object | null | Options for plugins. See Plugins section. |
Method | Description |
---|---|
reeller.resume() | Resumes movement. |
reeller.pause() | Pauses movement. |
reeller.reverse([reversed=true]) | Set reversed moving. |
reeller.invalidate() | Refresh GSAP Timeline. |
reeller.update() | Calculates and sets the number of clones and update movement position. |
reeller.refresh(update=true) | Fully refresh and update all clones and position. Use this only after adding or removing original items. |
reeller.destroy(removeClones=true) | Destroy Reeller instance, detach all observers and remove clones. |
Reeller.registerGSAP(gsap) | Static method to register the gsap library. |
Reeller.use(...plugins) | Static method to register a plugins. |
Property | Type | Description |
---|---|---|
reeller.paused | boolean | Indicates that the movement is stopped. |
reeller.tl | Timeline | GSAP Timeline. |
reeller.filler | Filler | Inner Filler instance. See Filler section. |
reeller.options | ReellerOptions | Current Reeller options. |
reeller.plugin | Object | Initialized plugins. |
Reeller comes with a useful events you can listen. Events can be assigned in this way:
reeller.on('update', () => {
console.log('Reeller update happened!');
});
You can also delete an event that you no longer want to listen in these ways:
reeller.off('update');
reeller.off('update', myHandler);
Event | Arguments | Description |
---|---|---|
update | (reeller, calcData) | Event will be fired after update. |
refresh | (reeller) | Event will be fired after refresh. |
destroy | (reeller) | Event will be fired after destroy. |
Reeller support plugins to extend functionality.
At this moment there is only one plugin that comes with the official package: ScrollerPlugin.
This plugin allows you to attach movement to the scroll:
import {Reeller, ScrollerPlugin} from 'reeller';
import gsap from 'gsap';
Reeller.registerGSAP(gsap);
Reeller.use(ScrollerPlugin);
const reeller = new Reeller({
container: '.my-reel',
wrapper: '.my-reel-wrap',
itemSelector: '.my-reel-item',
speed: 10,
plugins: {
scroller: {
speed: 1,
multiplier: 0.5,
threshold: 1,
},
},
});
The following options of ScrollerPlugin is available:
Option | Type | Default | Description |
---|---|---|---|
speed | number | 1 | Movement and inertia speed. |
multiplier | number | 0.5 | Movement multiplier. |
threshold | number | 1 | Movement threshold. |
ease | string | 'expo.out' | Timing function. See gsap easing. |
overwrite | boolean | true | See gsap overwrite modes. |
bothDirection | boolean | true | Allow movement in both directions. |
reversed | boolean | false | Reverse scroll movement. |
stopOnEnd | boolean | false | Stop movement on end scrolling. |
scrollProxy | function | null | A function that returns the scroll position. Can be used in cases with custom scroll. |
In cases with using of custom scroll libraries (e.g. smooth-scrollbar),
you can use the scrollProxy
option to return the current scroll position:
import Scrollbar from 'smooth-scrollbar';
import {Reeller, ScrollerPlugin} from 'reeller';
import gsap from 'gsap';
Reeller.registerGSAP(gsap);
Reeller.use(ScrollerPlugin);
const scrollbar = Scrollbar.init(document.querySelector('#my-scrollbar'));
const reeller = new Reeller({
container: '.my-reel',
wrapper: '.my-reel-wrap',
itemSelector: '.my-reel-item',
speed: 10,
plugins: {
scroller: {
speed: 1,
multiplier: 0.5,
threshold: 1,
scrollProxy: () => scrollbar.scrollTop,
},
},
});
Reeller library works on top of the Filler module. This is a separate tool for automatically calculating the number of clones and filling the container with them.
You can use only Filler (without Reeller) if you need to fill the whole space with clones, without movement, animations and GSAP:
import {Filler} from 'reeller';
const filler = new Filler({
container: '.my-container',
itemSelector: '.-my-item',
cloneClassName: '-clone',
});
Option | Type | Default | Description |
---|---|---|---|
container | string | HTMLElement | null | Required. Container element or selector. |
itemSelector | string | null | Required. Items CSS selector. |
wrapper | string | HTMLElement | null | Inner element or selector. |
cloneClassName | string | '-clone' | Class name of the new clones. |
autoUpdate | boolean | true | Use ResizeObserver to auto update clones number. |
clonesOverflow | boolean | false | Create artificial overflow with clones. |
clonesFinish | boolean | false | Bring the cycle of clones to an end. |
clonesMin | boolean | false | Minimum number of clones. |
Method | Description |
---|---|
filler.addClones(count, offset=0) | Creates and adds clones to end in the desired number from given offset. |
filler.removeClones(count) | Removes the desired number of clones from the end. |
filler.setClonesCount(count) | Sets the desired number of clones. |
filler.getCalcData() | Returns a calculated data object (number of clones, widths, etc.) |
filler.update() | Calculates and sets the number of clones. |
filler.refresh(update=true) | Fully refresh and update all clones. Use this only after adding or removing original items. |
filler.destroy(removeClones=true) | Destroy Filler instance, detach all observers and remove clones. |
Property | Type | Description |
---|---|---|
filler.container | HTMLElement | Container element. |
filler.wrapper | HTMLElement | Inner element. |
filler.item | Array.<HTMLElement> | Items array. |
filler.calcData | Object | Calculated data (number of clones, widths, etc.). |
filler.options | ReellerOptions | Current Filler options. |
Event | Arguments | Description |
---|---|---|
update | (filler, calcData) | Event will be fired after update. |
refresh | (filler) | Event will be fired after refresh. |
destroy | (filler) | Event will be fired after destroy. |
FAQs
Flexible, powerful and modern library for creating the running horizontal blocks effect, also known as ticker or the «marquee effect».
The npm package reeller receives a total of 377 weekly downloads. As such, reeller popularity was classified as not popular.
We found that reeller demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.