Regent: Business Rules in JavaScript
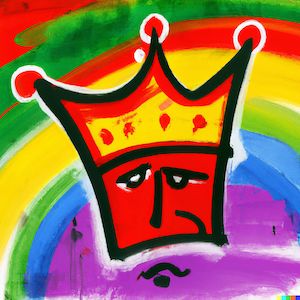
Regent lets you query a data structure by asking true or false questions. Regent logic is written as single responsibility rules that are self-documenting, composable, and human readable. Let's look at an example.
import { equals } from 'regent';
const isRaining = equals('@isRaining', true);
const data = { isRaining: true };
const isUmbrellaNeeded = isRaining(data);
equals
predicate documentation
Taking the previous example a bit further, we can refine the scenario to be more precise. We can create and combine multiple rules to test this condition
If it is raining and the wind isn't so strong the umbrella will turn inside-out and blow out of our hands, we need an umbrella.
import { and, equals, lessThan } from 'regent';
const isRaining = equals('@isRaining', true);
const isCalm = lessThan('@windSpeedInMph', 25);
const isRainingAndCalm = and(isRaining, isCalm);
const data = { isRaining: true, windSpeedInMph: 20 };
isRainingAndCalm(data);
composition
documentation
Regent also provides a simple way to find or filter array items based on a regent rule.
import { equals, lessThan, find, filter } from 'regent';
const isRaining = equals('@isRaining', true);
const isSunny = lessThan('@cloudCover', 10);
const isCold = lessThan('@temperature', 60);
const data = {
isRaining: false,
cloudCover: 0,
temperature: 42
};
const items = [
{ item: 'Winter hat', rule: isCold },
{ item: 'Sunglasses', rule: isSunny },
{ item: 'Umbrella', rule: isRaining },
]
filter(items, data)
Installation
npm install --save regent
License
MIT