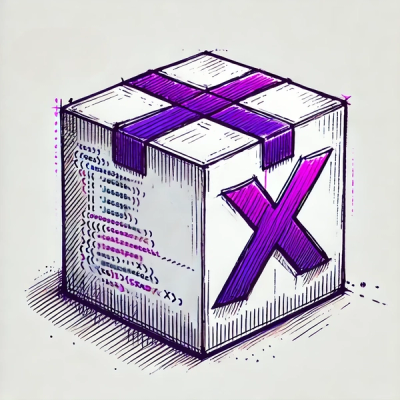
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
A small set of functionality used to create microservices that don't need to be aware of one-another's existence.
A small set of functionality used to create microservices that don't need to be aware of one-another's existence.
npm install remit
// Create our Remit connection
const remit = require('remit')({
url: 'localhost',
name: 'a.micro.service'
})
// Set up an endpoint with the name 'micro.service.info'
remit
.endpoint('micro.service.info')
// When the endpoint is hit, run this function
.data((data, callback) => {
console.log('Endpoint was hit!')
data.foo = 'bar'
// Reply with the mutated data
return callback(null, data)
// Once the endpoint is ready...
}).ready().then(() => {
// Make a request to the 'micro.service.save' endpoint
// with the data {name: 'a.micro.service'}
return remit
.request('micro.service.save')
.send({name: 'a.micro.service'})
}).then((result) => {
// When the reply comes back, log the response.
console.log('Saved microservice info', result)
}).catch((err) => {
// If the request failed (the replying microservice returned
// an error, the request timed out or couldn't be routed),
// log the error.
console.error('Couldn\'t seem to save microservice info', err)
})
Remit, primarily, makes use of four simple commands: request
, respond
, emit
and listen
.
request
requests data from an endpoint defined using respond
.
emit
"emits" data to any and all "listeners" defined using listen
.
A simple example here allows us to define an endpoint that increments a counter and emits that change to the rest of the system.
// This service sets up our counter and incrementer.
const remit = require('remit')()
let counter = 0
remit
.endpoint('counter.increment')
.data((event, callback) => {
remit.emit('counter.incremented').send(++counter)
return callback(null, counter)
})
// Here we set up a listener for when the counter's been incremented
const remit = require('remit')()
remit
.listen('counter.incremented')
.data((event, callback) => {
console.log(`Counter is now ${event.data}`)
return callback()
})
// And here we increment the counter!
const remit = require('remit')()
remit.request('counter.increment')()
Remit
A Remit instance representing a single connection to the AMQ in use.
require('remit')([options])
> Remit
^Creates a new Remit instance, using the given options, and connects to the message queue.
options
(Optional) An object containing a mixture of Remit and AMQ options. Acceptable values are currently:
url
(Optional, defaults to 'amqp://localhost'
) The RabbitMQ URI to use to connect to the AMQ instance. If not defined, tries to fall back to the REMIT_URL
environment variable before the default. Any query string options defined are overwritten.name
(Optional, defaults to ''
) The friendly connection name to give the service. This is used heavily for load balancing requests, so instances of the same service (that should load balance requests between themselves) should have the same name. If not defined, tries to fall back to the REMIT_NAME
environment variable before the default.exchange
(Optional, defaults to 'remit'
) The AMQ exchange to connect to.Remit
Request([data])
^A request set up for a specific endpoint, ready to send and receive data.
data
(Optional) Can be any JSON-compatible data that you wish to send to the endpoint.send
Synonymous with calling the Request
object.data(callback)
Provide a callback to be run when a reply is received from a request.sent(callback)
Provide a callback to be run when the request successfully sends data to an endpoint.Returns a promise that resolves when the reply is received. If timeout
or error
are emitted, the promise is rejected. If data
is emitted with a falsey err
, the promise is resolved. If the err
is truthy, the promise is rejected.
remit.request(endpoint[, options])
> Request
^Aliases: req
Sets up a request pointed at the specified endpoint
with the given options
.
endpoint
A string representing an endpoint, defined using respond
/ res
/ endpoint
.options
(Optional) An object containing a mixture of Remit and AMQ options. Acceptable values are currently:
something
data(callback)
Provide a global callback to be run when a reply is received from any request.sent(callback)
Provide a global callback to be run when any request successfully sends data to an endpoint.Request
Some basic options templates are also set up as separate functions to allow for some semantically-pleasing set-ups without having to skim through objects to figure out what's happening.
// remit.persistentRequest
// remit.preq
{
"some": "thing"
}
// remit.emit
{
"some": "thing"
}
// remit.delayedEmit
// remit.demit
{
"some": "thing"
}
amq.rabbitmq.reply-to
Response
^An active endpoint set up and ready to receive data.
data
Provide a callback to be run when a request is received.ready
Provide a callback to be run when the endpoint becomes live and ready.remit.respond(endpoint[, options])
> Response
^Aliases: res
, endpoint
endpoint
A string representing the name of the endpoint. This is used as a routing key (see the RabbitMQ Topics Tutorial) so the only allowed characters are a-zA-Z0-9_.-
.options
(Optional) An object containing a mixture of Remit and AMQ options. Acceptable values are currently:
something
data
Provide a global callback to be run when any request is received.ready
Provide a global callback to be run when any endpoint becomes live and ready.Response
Some basic options templates are also set up as separate functions to allow for some semantically-pleasing set-ups without having to skim through objects to figure out what's happening
// remit.listen
// remit.on
{
"some": "thing"
}
FAQs
A small set of functionality used to create microservices that don't need to be aware of one-another's existence.
The npm package remit receives a total of 21 weekly downloads. As such, remit popularity was classified as not popular.
We found that remit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.