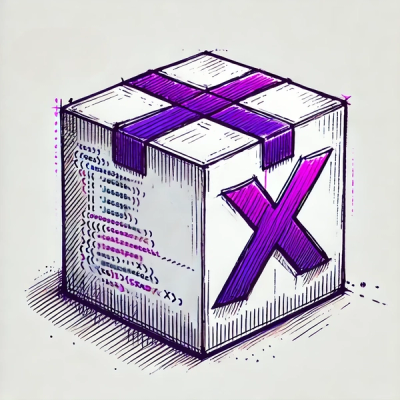
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Remixin is the aspect-oriented mixin library developed and in use at SoundCloud. It is inspired by Twitter's advice.js and Joose.
For an introduction about why you'd want to use a mixin library, Angus Croll and Dan Webb from Twitter gave a good talk about the concept and Angus blogged on the subject.
Remixin runs in the browser and NodeJS (available as an npm module). It only has one dependency: underscore.js (or lodash). This library is included automatically as a dependency in node, but is not bundled for browser usage, since you likely are already using underscore or lodash in your application anyway. To use Remixin in the browser, it uses dependency injection to get access to underscore:
// node.js
var Mixin = require('remixin'); // ready to go!
<!-- used in the browser -->
<html>
<script src="underscore.js"></script>
<script src="remixin-global.js"></script> <!-- creates window.Remixin -->
<script>
Mixin = Remixin(_); // pass underscore to the global object and it returns the class ready to go.
</script>
Alternatively, if you're using requirejs, browserify or something similar for browser usage, as long as require('underscore')
will return something useful, you can use the commonjs version the same as node.
There are 4 files provided in the repository:
remixin-cjs.js
remixin-global.js
remixin-dev-cjs.js
remixin-dev-global.js
Files with 'cjs' use CommonJS modules. Remixin is exported on module.exports
and gets access to underscore via require('underscore')
.
Files with 'global' export Remixin as a property on the global object (window
, in the browser). This needs to be injected with underscore before use, as shown above.
Files with 'dev' are for development mode: they are not minified, and include error checking.
Since it's not a real JS library readme if there's not a mention of its footprint, Remixin is 772 bytes gzipped.
mixin = new Mixin(modifiers)
mixin.applyTo(object)
mixin.applyTo(object, options)
curried = mixin.withOptions(options)
combined = new Mixin(mixin1, [mixin2, ...], modifiers)
When defining a mixin, there are several key words to define method modifiers:
before
: {Object.<String,Function>}
after
: {Object.<String,Function>}
before
, this has the same signature, but can not modify the return value of the function.around
: {Object.<String,Function>}
requires
: {Array.<String>}
requirePrototype
: {Object}
override
: {Object.<String,*>}
defaults
: {Object.<String,*>}
merge
: {Object.<String,Array|Object|String}
_.defaults(target.obj, mixin.obj)
.All other keys are copied onto the target object unless that key already exists. If overriding these keys is desired,
then it should be defined in the override
block. If a default implementation is desired, then it should be defined in
the defaults
block.
If using the development build of Remixin, incorrect use of these modifiers will throw an error. For example, if a
field declared in requires
is not found, or trying to apply a before
on a non-function. The production build skips
these checks altogether, either failing silently or creating unwanted behaviour when used. For this reason, you should
definitely use the development version while working.
applyTo
If custom code is required for your mixin, then defining a key named 'applyTo' allows a custom method to be executed when the mixin is applied. This method is passed two arguments: the target object and any options defined by the calling code:
zoomable = new Mixin({
applyTo: function (obj, options) {
this.extend(obj, {
zoom: function () {
this.width *= options.zoomRatio;
this.height *= options.zoomRatio;
}
});
}
});
zoomable.applyTo(MyCanvasObject.prototype, { zoomRatio: 2 });
All of the standard modifier names (eg: after, around, before) are available in the context, as well as extend
to add
new properties.
Taking the example from above, sometimes it's more convenient to have the options curried into the mixin already. For
this, use .withOptions
which will return a new mixin with those options stored. For example:
var standardDPI = zoomable.withOptions({ zoomRatio: 1});
var highDPI = zoomable.withOptions({ zoomRatio: 2 });
Sometimes, one mixin will necessitate the target object also having another mixin. For example, you might have a mixin which gives a View the behaviour of a drop-down menu. Drop-down menus have some shared behaviour with other overlays, such as modal dialogues. These can be combined into a single mixin, to hide the implementation from the class which requires the combined behaviour:
overlay = new Mixin({
merge: {
events: {
'click .closeButton': 'onCloseClick'
}
},
show: function () { ... },
hide: function () { ... },
onCloseClick: function () {
this.hide();
}
});
dropDownMenu = new Mixin(overlay, {
after: {
onCloseClick: function () {
this.parentButton.focus();
}
}
});
ProfileButton = View.extend({ ... });
dropDownMenu.applyTo(ProfileButton.prototype);
Any number of mixins can be combined into one:
megaMixin = new Mixin(mixin1, mixin2, mixin3, mixin4, {});
To create the builds:
make
To run the tests in node:
make test
To run the tests in your browser:
npm run test-server
...and then open your browser to http://127.0.0.1:8080/test.html
To see a coverage report:
make coverage
1.0.0 (2015-01-18)
FAQs
Aspect-oriented, mixin library
The npm package remixin receives a total of 1 weekly downloads. As such, remixin popularity was classified as not popular.
We found that remixin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.