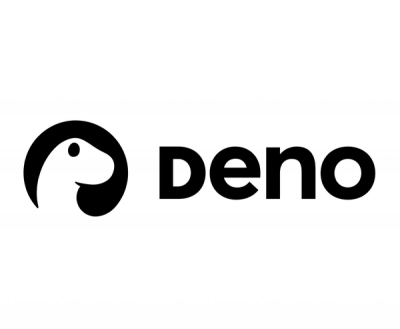
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
remote-pay-cloud
Advanced tools
Current version: 1.3.1-rc1.2
This SDK provides an API to allow your application using Javascript to interface with a Clover® Mini device (https://www.clover.com/pos-hardware/mini)
The API is available on GitHub for download, and can be used:
require
directive, hosted on NPMSale()
, VoidTransaction()
, ManualRefund()
, etc.onSaleResponse
, onRefundPaymentResponse
, etc.Payment
, CardTransaction
, Order
, etc. These objects will match those defined in clover-android-sdkIf used from a browser, the library requires the browser you use to support WebSockets. See WebSocket Browser Support.
For more developer documentation and information about the Semi-Integration program, please visit our [semi-integration developer documents] (https://docs.clover.com/build/integration-overview-requirements/).
A sale/refund UI example project that connects to a device via the Clover cloud Clover Cloud Connector Example is available for download and deployment, or direct deployment to a Heroku server.
A example project composed of small examples that connect to a device via the Clover cloud - Clover Cloud Connector Unit Examples is available for download and deployment, or direct deployment to a Heroku server.
Please report any questions/comments/concerns to us by emailing semi-integrations@clover.com.
Clover's cloud connector API. Published as an NPM package. Intended for use in a browser environment, or in a NodeJS application.
This shows how you can make a connection using plain javascript in the browser to a Clover device using the Cloud Pay Display.
var $ = require('jQuery');
var clover = require("remote-pay-cloud");
var log = clover.Logger.create();
var connector = new clover.CloverConnectorFactory().createICloverConnector({
"oauthToken": "1e7a9007-141a-293d-f41d-f603f0842139",
"merchantId": "BBFF8NBCXEMDV",
"clientId": "3RPTN642FHXTX",
"remoteApplicationId": "com.yourname.yourapplication:1.0.0-beta1",
"deviceSerialId": "C031UQ52340015",
"domain": "https://sandbox.dev.clover.com/"
});
var ExampleCloverConnectorListener = function(cloverConnector) {
clover.remotepay.ICloverConnectorListener.call(this);
this.cloverConnector = cloverConnector;
};
ExampleCloverConnectorListener.prototype = Object.create(clover.remotepay.ICloverConnectorListener.prototype);
ExampleCloverConnectorListener.prototype.constructor = ExampleCloverConnectorListener;
ExampleCloverConnectorListener.prototype.onReady = function (merchantInfo) {
var saleRequest = new clover.remotepay.SaleRequest();
saleRequest.setExternalId(clover.CloverID.getNewId());
saleRequest.setAmount(10000);
this.cloverConnector.sale(saleRequest);
};
ExampleCloverConnectorListener.prototype.onVerifySignatureRequest = function (request) {
log.info(request);
this.cloverConnector.acceptSignature(request);
};
ExampleCloverConnectorListener.prototype.onConfirmPaymentRequest = function (request) {
this.cloverConnector.acceptPayment(request.payment);
};
ExampleCloverConnectorListener.prototype.onSaleResponse = function (response) {
log.info(response);
connector.dispose();
if(!response.getIsSale()) {
console.error("Response is not an sale!");
console.error(response);
}
};
var connectorListener = new ExampleCloverConnectorListener(connector);
connector.addCloverConnectorListener(connectorListener);
connector.initializeConnection();
// Close the connection cleanly on exit. This should be done with all connectors.
$(window).on('beforeunload ', function () {
try {
connector.dispose();
} catch (e) {
console.log(e);
}
});
var clover = require("remote-pay-cloud");
This will require gathering the configuration information to create the connector. In this example, the configuration is hard coded. The creation of the connector is done using the connector factory.
var connector = new clover.CloverConnectorFactory().createICloverConnector({
"merchantId": "BBFF8NBCXEMDT",
"clientId": "3RPTN642FHXTC",
"remoteApplicationId": "com.yourname.yourapplication:1.0.0-beta1",
"deviceSerialId": "C031UQ52340045",
"domain": "https://sandbox.dev.clover.com/"
});
There are several ways the Clover Connector object can be configured.
Examples of configurations that can be used when creating the Clover Connector object:
{
"clientId" : "3BZPZ6A6FQ8ZM",
"remoteApplicationId": "com.yourname.yourapplication:1.0.0-beta1",
"domain" : "https://sandbox.dev.clover.com/",
"merchantId" : "VKYQ0RVGMYHRS",
"deviceSerialId" : "C021UQ52341078"
}
{
"oauthToken" : "6e6313e8-fe33-8662-7ff2-3a6690e0ff14",
"domain" : "https://sandbox.dev.clover.com/",
"merchantId" : "VKYQ0RVGMYHRS",
"clientId" : "3BZPZ6A6FQ8ZM",
"remoteApplicationId": "com.yourname.yourapplication:1.0.0-beta1",
"deviceSerialId" : "C021UQ52341078"
}
The functions implemented will be called as the connector encounters the events. These functions can be found in the clover.remotepay.ICloverConnectorListener.
// This overrides/implements the constructor function. This example
// expects that a clover connector implementation instance is passed to the created listener.
var ExampleCloverConnectorListener = function(cloverConnector) {
clover.remotepay.ICloverConnectorListener.call(this);
this.cloverConnector = cloverConnector;
};
ExampleCloverConnectorListener.prototype = Object.create(clover.remotepay.ICloverConnectorListener.prototype);
ExampleCloverConnectorListener.prototype.constructor = ExampleCloverConnectorListener;
// The ICloverConnectorListener function that is called when the device is ready to be used.
// This example starts up a sale for $100
ExampleCloverConnectorListener.prototype.onReady: function (merchantInfo) {
var saleRequest = new clover.remotepay.SaleRequest();
saleRequest.setExternalId(clover.CloverID.getNewId());
saleRequest.setAmount(10000);
this.cloverConnector.sale(saleRequest);
};
// The ICloverConnectorListener function that is called when the device needs to have a signature
// accepted, or rejected.
// This example accepts the signature, sight unseen
ExampleCloverConnectorListener.prototype.onVerifySignatureRequest = function (request) {
log.info(request);
this.cloverConnector.acceptSignature(request);
};
// The ICloverConnectorListener function that is called when the device detects a possible duplicate transaction,
// due to the same card being used in a short period of time. This example accepts the duplicate payment challenge, sight unseen
ExampleCloverConnectorListener.prototype.onConfirmPaymentRequest = function (request) {
this.cloverConnector.acceptPayment(request.payment);
};
// The ICloverConnectorListener function that is called when a sale request is completed.
// This example logs the response, and disposes of the connector. If the response is not an expected
// type, it will log an error.
ExampleCloverConnectorListener.prototype.onSaleResponse = function (response) {
log.info(response);
connector.dispose();
if(!response.getIsSale()) {
console.error("Response is not an sale!");
console.error(response);
}
};
var connectorListener = new ExampleCloverConnectorListener(connector);
connector.addCloverConnectorListener(connectorListener);
connector.initializeConnection();
This example uses jQuery to add a hook for the window beforeunload
event that ensures that the connector is displosed of.
$(window).on('beforeunload ', function () {
try {
connector.dispose();
} catch (e) {
console.log(e);
}
});
This shows how you can make a connection using typescript in the browser to a Clover device using the Network Pay Display.
var $ = require('jQuery');
import * as Clover from 'remote-pay-cloud';
export class StandAloneExampleWebsocketPairedCloverDeviceConfiguration extends Clover.WebSocketPairedCloverDeviceConfiguration {
public constructor() {
super(
"wss://Clover-C030UQ50550081.local.:12345/remote_pay",
"test.js.test:0.0.1",
"My_Pos_System",
"8675309142856",
null,
Clover.BrowserWebSocketImpl.createInstance
);
}
public onPairingCode(pairingCode: string): void {
console.log("Pairing code is " + pairingCode + " you will need to enter this on the device.");
}
public onPairingSuccess(authToken: string): void {
console.log("Pairing succeeded, authToken is " + authToken);
}
}
export class StandAloneExampleCloverConnectorListener extends Clover.remotepay.ICloverConnectorListener {
protected cloverConnector: Clover.remotepay.ICloverConnector;
private testStarted: boolean;
constructor(cloverConnector: Clover.remotepay.ICloverConnector) {
super();
this.cloverConnector = cloverConnector;
this.testStarted = false;
}
protected onReady(merchantInfo: Clover.remotepay.MerchantInfo): void {
console.log("In onReady, starting test", merchantInfo);
if(!this.testStarted) {
this.testStarted = true;
}
let saleRequest:Clover.remotepay.SaleRequest = new Clover.remotepay.SaleRequest();
saleRequest.setExternalId(Clover.CloverID.getNewId());
saleRequest.setAmount(10);
console.log({message: "Sending sale", request: saleRequest});
this.cloverConnector.sale(saleRequest);
}
public onSaleResponse(response:Clover.remotepay.SaleResponse): void {
try{
console.log({message: "Sale response received", response: response});
if (!response.getIsSale()) {
console.error("Response is not a sale!");
}
console.log("Test Completed. Cleaning up.");
this.cloverConnector.showWelcomeScreen();
this.cloverConnector.dispose();
} catch (e) {
console.error(e);
}
}
protected onConfirmPaymentRequest(request: Clover.remotepay.ConfirmPaymentRequest): void {
console.log({message: "Automatically accepting payment", request: request});
this.cloverConnector.acceptPayment(request.getPayment());
}
protected onVerifySignatureRequest(request: Clover.remotepay.onVerifySignatureRequest): void {
console.log({message: "Automatically accepting signature", request: request});
this.cloverConnector.acceptSignature(request);
}
protected onDeviceError(deviceErrorEvent: Clover.remotepay.CloverDeviceErrorEvent): void {
console.error("onDeviceError", deviceErrorEvent);
}
}
let configuration = {};
configuration[Clover.CloverConnectorFactoryBuilder.FACTORY_VERSION] = Clover.CloverConnectorFactoryBuilder.VERSION_12;
let connectorFactory: Clover.ICloverConnectorFactory = Clover.CloverConnectorFactoryBuilder.createICloverConnectorFactory(
configuration
);
let cloverConnector: Clover.remotepay.ICloverConnector =
connectorFactory.createICloverConnector( new StandAloneExampleWebsocketPairedCloverDeviceConfiguration());
cloverConnector.addCloverConnectorListener(new StandAloneExampleCloverConnectorListener(cloverConnector));
$(window).on('beforeunload ', function () {
try {
cloverConnector.dispose();
} catch (e) {
console.log(e);
}
});
cloverConnector.initializeConnection();
import * as Clover from 'remote-pay-cloud';
Depending on the mode of configuration, you may choose to use a WebSocketPairedCloverDeviceConfiguration, or a WebSocketCloudCloverDeviceConfiguration.
export class StandAloneExampleWebsocketPairedCloverDeviceConfiguration extends
Clover.WebSocketPairedCloverDeviceConfiguration {
...
}
There are many ways the Clover Connector object can be configured. This includes a direct connection with a browser as shown here, connecting using a browser via the cloud similar to the above example, and connecting using a NodeJS application.
The functions implemented will be called as the connector encounters the events. These functions can be found in the clover.remotepay.ICloverConnectorListener.
export class StandAloneExampleCloverConnectorListener extends Clover.remotepay.ICloverConnectorListener {
...
}
The factory can be obtained using the builder. If unspecified, the factory will produce 1.1.0 compatible connectors. Here we specify the 1.2 version.
let configuration = {};
configuration[Clover.CloverConnectorFactoryBuilder.FACTORY_VERSION] =
Clover.CloverConnectorFactoryBuilder.VERSION_12;
let connectorFactory: Clover.ICloverConnectorFactory =
Clover.CloverConnectorFactoryBuilder.createICloverConnectorFactory(configuration);
Using the configuration object you created, call the factory function to get an instance of a Clover Connector.
let cloverConnector: Clover.remotepay.ICloverConnector =
connectorFactory.createICloverConnector(
new StandAloneExampleWebsocketPairedCloverDeviceConfiguration());
cloverConnector.addCloverConnectorListener(new StandAloneExampleCloverConnectorListener(cloverConnector));
cloverConnector.initializeConnection();
This example uses jQuery to add a hook for the window beforeunload
event that ensures that the connector is displosed of.
$(window).on('beforeunload ', function () {
try {
connector.dispose();
} catch (e) {
console.log(e);
}
});
This library has been tested against the following Browser type and versions:
API documentation is generated when npm install
is run.
Online Docs and
Online API class Docs
Added support for Custom Activities
SEMI-889 Remove automatic transition to welcome screen when device is ready
SEMI-695 Addition of response to resetDevice
call.
SEMI-795 Addition of retrievePayment functionality.
SEMI-777 Addition of custom activity support.
require("remote-pay-cloud").DebugConfig.loggingEnabled = true;
A deprecated beta version of the Connector (Clover.js) is included in this version with require
directive syntax, but will removed in the future.
The beta version includes the earliest library as well as a server with examples of the functions.
FAQs
Access Clover devices through the cloud.
We found that remote-pay-cloud demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.