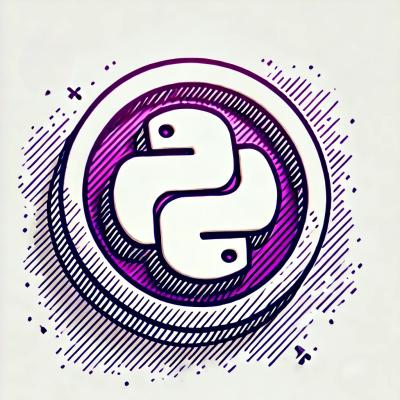
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Standalone library for ajax requests.
Execute this command in your project for install the package:
npm install --save req-ajax
After the download you should install the dependencies and build es2015 version with babel.js, so execute this:
cd node_modules/req-ajax; npm run build; cd -
The file ajax.js
and ajax.min.js
are in dist
directory.
Simple external script in your html file:
<script src="node_modules/req-ajax/dist/ajax.min.js"></script>
All methods return a
Promise
object.
ajax.query(Object)
the Object is the config of that request, this object can have 3 properties:
url
{String} is required.method
{String} is required.params
{Object} is optional, this property is for specific queries. The queries like this foo=bar&bar=foo
can be transformed to { foo: 'bar', bar: 'foo' }
in the JavaScript object notation, so is much better for make queries and easy.Example:
ajax.query({
url: 'somepath',
method: 'GET',
params: {
foo: 'bar'
}
// the url should be 'somepath?foo=bar'.
}).then(function (res) {
// Do something with the response...
}).catch(function (err) {
// Manage the error of the request (if appear).
})
ajax.get(url [, json])
This method is an shorthand for GET requests.
Params:
url
{String} is required.json
{Boolean} is optional, default false
. This param is for when the response is json string, if json
is true
the response is parser for convert it to a object normal.Per Example the file somefile.json
contain { "foo": "bar" }
:
ajax.get('somefile.json', true)
.then(function (res) {
if (res.foo === 'bar') {
// Do something...
}
}).catch(function (err) {
// Trap the error.
})
ajax.post(url, data [, json])
This method is an shorthand for POST requests.
Params:
url
{String} is required.data
{String|Number|Object|Boolean} is required.json
{Boolean} is optional, default false
. This param is for when the data is object normal and will send it as a json string.Example:
const data = { foo: 'bar' }
// The data is transformed to '{ "foo": "bar"}'
ajax.post('somepath', data, true)
.then(function ()
// The post request was complete.
}).catch(function (err) {
// Something it's wrong.
})
For more examples and test execute npm run test
and open your browser in the url http://localhost:8080
.
FAQs
Standalone library for ajax requests
The npm package req-ajax receives a total of 1 weekly downloads. As such, req-ajax popularity was classified as not popular.
We found that req-ajax demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.