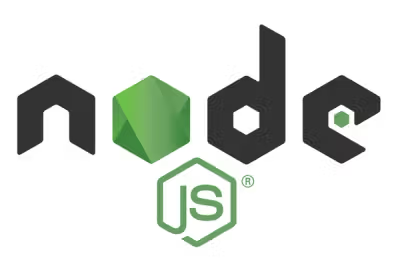
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
request-light
Advanced tools
The request-light npm package is a lightweight HTTP client for making HTTP requests. It is designed to be simple and efficient, providing basic functionalities for making GET, POST, PUT, and DELETE requests.
GET Request
This feature allows you to make a GET request to a specified URL and handle the response.
const request = require('request-light');
request.xhr({ url: 'https://api.example.com/data', type: 'GET' }).then(response => {
console.log(response.responseText);
}).catch(error => {
console.error(error);
});
POST Request
This feature allows you to make a POST request to a specified URL with a JSON payload.
const request = require('request-light');
request.xhr({ url: 'https://api.example.com/data', type: 'POST', data: JSON.stringify({ key: 'value' }) }).then(response => {
console.log(response.responseText);
}).catch(error => {
console.error(error);
});
PUT Request
This feature allows you to make a PUT request to a specified URL with a JSON payload to update existing data.
const request = require('request-light');
request.xhr({ url: 'https://api.example.com/data/1', type: 'PUT', data: JSON.stringify({ key: 'newValue' }) }).then(response => {
console.log(response.responseText);
}).catch(error => {
console.error(error);
});
DELETE Request
This feature allows you to make a DELETE request to a specified URL to delete existing data.
const request = require('request-light');
request.xhr({ url: 'https://api.example.com/data/1', type: 'DELETE' }).then(response => {
console.log(response.responseText);
}).catch(error => {
console.error(error);
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more powerful and flexible API compared to request-light, including support for interceptors, automatic JSON transformation, and more.
Node-fetch is a lightweight module that brings `window.fetch` to Node.js. It is similar to request-light in terms of simplicity but follows the Fetch API standard, making it a good choice for those familiar with the Fetch API in the browser.
Got is a human-friendly and powerful HTTP request library for Node.js. It offers a more extensive feature set than request-light, including retries, streams, and advanced error handling.
A lightweight request library intended to be used by VSCode extensions.
configure
or HTTP_PROXY
and HTTPS_PROXY
env variables to configure the HTTP proxy addresses.import { xhr, XHRResponse, getErrorStatusDescription } from 'request-light';
const headers = { 'Accept-Encoding': 'gzip, deflate' };
return xhr({ url: url, followRedirects: 5, headers }).then(response => {
return response.responseText;
}, (error: XHRResponse) => {
throw new Error(error.responseText || getErrorStatusDescription(error.status) || error.toString());
});
FAQs
Lightweight request library. Promise based, with proxy support.
We found that request-light demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.