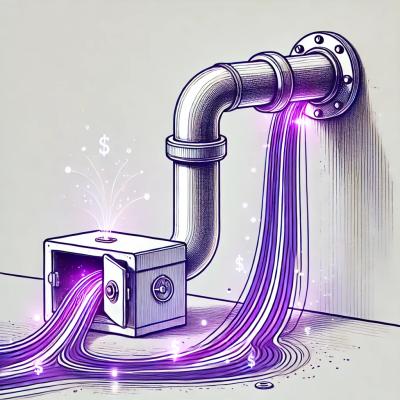
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
request-promise
Advanced tools
The world-famous HTTP client 'Request' now Promises/A+ compliant. Powered by Bluebird.
The request-promise npm package is a popular HTTP client for making HTTP requests from Node.js. It is built on top of the 'request' package, providing a simplified way to make HTTP calls and process responses using promises instead of callbacks.
Simple HTTP GET requests
This feature allows you to perform simple HTTP GET requests and process the response as a string.
const rp = require('request-promise');
rp('http://example.com')
.then(function (htmlString) {
// Process html...
})
.catch(function (err) {
// Handle error...
});
HTTP requests with options
This feature allows you to perform HTTP requests with a full set of options, including query strings, headers, and automatic JSON parsing.
const rp = require('request-promise');
const options = {
uri: 'http://api.example.com',
qs: {
access_token: 'my-token' // -> uri + '?access_token=my-token'
},
headers: {
'User-Agent': 'Request-Promise'
},
json: true // Automatically parses the JSON string in the response
};
rp(options)
.then(function (repos) {
// Process repos...
})
.catch(function (err) {
// Handle error...
});
POST requests with form data
This feature allows you to perform HTTP POST requests with form data.
const rp = require('request-promise');
const options = {
method: 'POST',
uri: 'http://api.example.com/form',
form: {
key: 'value',
another_key: 'another_value'
},
headers: {
/* 'content-type': 'application/x-www-form-urlencoded' */ // Is set automatically
}
};
rp(options)
.then(function (parsedBody) {
// POST succeeded...
})
.catch(function (err) {
// POST failed...
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides an easy-to-use API for making HTTP requests and can be used in both the front-end and back-end. It supports interceptors, request and response transformations, and automatic JSON data transformation, making it more feature-rich compared to request-promise.
Got is a human-friendly and powerful HTTP request library for Node.js. It is designed to be a simpler and more lightweight alternative to request-promise, with support for streams and promises. Got also provides a more modern API with built-in support for JSON and better error handling.
node-fetch is a light-weight module that brings the Fetch API to Node.js. It is a minimalistic and straightforward API that closely resembles the browser's fetch API, making it ideal for developers who prefer the fetch syntax and are looking for a simple way to make HTTP requests in Node.js.
Superagent is a small progressive client-side HTTP request library. It has a flexible and chainable API that allows for easy construction of complex queries. It is often compared to request-promise for its simplicity and ease of use but is more focused on being used in web browsers, although it works in Node.js as well.

The world-famous HTTP client "Request" now Promises/A+ compliant. Powered by Bluebird.
Bluebird and Request are pretty awesome, but I found myself using the same design pattern. Request-Promise adds a Bluebird-powered .then(...)
method to Request call objects. By default, http response codes other than 2xx will cause the promise to be rejected. This can be overwritten by setting options.simple
to false
.
This module is installed via npm:
npm install request-promise
Request-Promise depends on loosely defined versions of Request and Bluebird. If you want to use specific versions of those modules please install them beforehand.
var rp = require('request-promise');
rp('http://www.google.com')
.then(function (htmlString) {
// Process html...
})
.catch(function (err) {
// Crawling failed...
});
var cheerio = require('cheerio'); // Basically jQuery for node.js
var options = {
uri: 'http://www.google.com',
transform: function (body) {
return cheerio.load(body);
}
};
rp(options)
.then(function ($) {
// Process html like you would with jQuery...
})
.catch(function (err) {
// Crawling failed or Cheerio choked...
});
var options = {
uri: 'https://api.github.com/user/repos',
qs: {
access_token: 'xxxxx xxxxx' // -> uri + '?access_token=xxxxx%20xxxxx'
},
headers: {
'User-Agent': 'Request-Promise'
},
json: true // Automatically parses the JSON string in the response
};
rp(options)
.then(function (repos) {
console.log('User has %d repos', repos.length);
})
.catch(function (err) {
// API call failed...
});
var options = {
method: 'POST',
uri: 'http://posttestserver.com/post.php',
body: {
some: 'payload'
},
json: true // Automatically stringifies the body to JSON
};
rp(options)
.then(function (parsedBody) {
// POST succeeded...
})
.catch(function (err) {
// POST failed...
});
var options = {
method: 'POST',
uri: 'http://posttestserver.com/post.php',
form: {
some: 'payload' // Will be urlencoded
},
headers: {
/* 'content-type': 'application/x-www-form-urlencoded' */ // Set automatically
}
};
rp(options)
.then(function (body) {
// POST succeeded...
})
.catch(function (err) {
// POST failed...
});
var options = {
method: 'DELETE',
uri: 'http://my-server/path/to/resource/1234',
resolveWithFullResponse: true // <--- <--- <--- <---
};
rp(options)
.then(function (response) {
console.log("DELETE succeeded with status %d", response.statusCode);
})
.catch(function (err) {
// Delete failed...
});
var options = {
uri: 'http://www.google.com/this-page-does-not-exist.html',
simple: false // <--- <--- <--- <---
};
rp(options)
.then(function (body) {
// Request succeeded but might as well be a 404
// Usually combined with resolveWithFullResponse = true to check response.statusCode
})
.catch(function (err) {
// Request failed due to technical reasons...
});
For more options checkout the Request docs.
Consider Request-Promise being:
require('request-promise') == require('request')
so to say.pipe(...)
) is DISCOURAGED because Request-Promise would grow the memory footprint for large requests unnecessarily high. Use the original Request library for that. You can use both libraries in the same project.rp(...).then(...)
or e.g. rp.post(...).then(...)
which turn rp(...)
and rp.post(...)
into promisesrp(...).catch(...)
or e.g. rp.del(...).catch(...)
which is the same method as provided by Bluebird promisesrp(...).finally(...)
or e.g. rp.put(...).finally(...)
which is the same method as provided by Bluebird promisesrp(...).promise()
or e.g. rp.head(...).promise()
which returns the underlying promise so you can access the full Bluebird APIsimple
which is a boolean to set whether status codes other than 2xx should also reject the promiseresolveWithFullResponse
which is a boolean to set whether the promise should be resolve with the full response or just the response bodytransform
which takes a function to transform the response into a custom value with which the promise is resolvedThe objects returned by request calls like rp(...)
or e.g. rp.post(...)
are regular Promises/A+ compliant promises and can be assimilated by any compatible promise library.
The methods .then(...)
, .catch(...)
, and .finally(...)
- which you can call on the request call objects - return a full-fledged Bluebird promise. That means you have the full Bluebird API available for further chaining. E.g.: rp(...).then(...).spread(...)
If, however, you need a method other than .then(...)
, .catch(...)
, or .finally(...)
to be FIRST in the chain, use .promise()
: rp(...).promise().bind(...).then(...)
// As a Request user you would write:
var request = require('request');
request('http://google.com', function (err, response, body) {
if (err) {
handleError({ error: err, response: response, ... });
} else if (!(/^2/.test('' + response.statusCode))) { // Status Codes other than 2xx
handleError({ error: body, response: response, ... });
} else {
process(body);
}
});
// As a Request-Promise user you can now write the equivalent code:
var rp = require('request-promise');
rp('http://google.com')
.then(process, handleError);
// The same is available for all http method shortcuts:
request.post('http://example.com/api', function (err, response, body) { ... });
rp.post('http://example.com/api').then(...);
rp('http://google.com')
.catch(handleError);
// ... is syntactical sugar for:
rp('http://google.com')
.then(null, handleError);
// By the way, this:
rp('http://google.com')
.then(process)
.catch(handleError);
// ... is equivalent to:
rp('http://google.com')
.then(process, handleError);
rp('http://google.com')
.finally(function () {
// This is called after the request finishes either successful or not successful.
});
In order to not pollute the Request call objects with the methods of the underlying Bluebird promise, only .then(...)
, .catch(...)
, and .finally(...)
were exposed to cover most use cases. The effect is that any methods of a Bluebird promise other than .then(...)
, .catch(...)
, or .finally(...)
cannot be used as the FIRST method in the promise chain:
// This works:
rp('http://google.com').then(function () { ... });
rp('http://google.com').catch(function () { ... });
// This works as well since additional methods are only used AFTER the FIRST call in the chain:
rp('http://google.com').then(function () { ... }).spread(function () { ... });
rp('http://google.com').catch(function () { ... }).error(function () { ... });
// Using additional methods as the FIRST call in the chain does not work:
// rp('http://google.com').bind(this).then(function () { ... });
// Use .promise() in these cases:
rp('http://google.com').promise().bind(this).then(function () { ... });
resolveWithFullResponse
option// Per default the body is passed to the fulfillment handler:
rp('http://google.com')
.then(function (body) {
// Process the html of the Google web page...
});
// The resolveWithFullResponse options allows to pass the full response:
rp({ uri: 'http://google.com', resolveWithFullResponse: true })
.then(function (response) {
// Access response.statusCode, response.body etc.
});
simple
option// The rejection handler is called with a reason object...
rp('http://google.com')
.catch(function (reason) {
// Handle failed request...
});
// ... and would be equivalent to this Request-only implementation:
var options = { uri: 'http://google.com' };
request(options, function (err, response, body) {
var reason;
if (err) {
reason = {
cause: err,
error: err,
options: options,
response: response
};
} else if (!(/^2/.test('' + response.statusCode))) { // Status Codes other than 2xx
reason = {
statusCode: response.statusCode,
error: body,
options: options,
response: response
};
}
if (reason) {
// Handle failed request...
}
});
// If you pass the simple option as false...
rp({ uri: 'http://google.com', simple: false })
.catch(function (reason) {
// Handle failed request...
});
// ... the equivalent Request-only code would be:
request(options, function (err, response, body) {
if (err) {
var reason = {
cause: err,
error: err,
options: options,
response: response
};
// Handle failed request...
}
});
// E.g. a 404 would now fulfill the promise.
// Combine it with resolveWithFullResponse = true to check the status code in the fulfillment handler.
With version 0.4 the reason objects became Error objects with identical properties to ensure backwards compatibility. These new Error types allow targeted catch blocks:
var errors = require('request-promise/errors');
rp('http://google.com')
.catch(errors.StatusCodeError, function (reason) {
// The server responded with a status codes other than 2xx.
// Check reason.response.statusCode.
})
.catch(errors.RequestError, function (reason) {
// The request failed due to technical reasons.
// reason.cause is the Error object Request would pass into a callback.
});
transform
functionYou can pass a function to options.transform
to generate a custom fulfillment value when the promise gets resolved.
// Just for fun you could reverse the response body:
var options = {
uri: 'http://google.com',
transform: function (body, response, resolveWithFullResponse) {
return body.split('').reverse().join('');
}
};
rp(options)
.then(function (reversedBody) {
// ;D
});
// However, you could also do something useful:
var $ = require('cheerio'); // Basically jQuery for node.js
function autoParse(body, response, resolveWithFullResponse) {
// FIXME: The content type string could contain additional values like the charset.
if (response.headers['content-type'] === 'application/json') {
return JSON.parse(body);
} else if (response.headers['content-type'] === 'text/html') {
return $.load(body);
} else {
return body;
}
}
options.transform = autoParse;
rp(options)
.then(function (autoParsedBody) {
// :)
});
// You can go one step further and set the transform as the default:
var rpap = rp.defaults({ transform: autoParse });
rpap('http://google.com')
.then(function (autoParsedBody) {
// :)
});
rpap('http://echojs.com')
.then(function (autoParsedBody) {
// =)
});
The third resolveWithFullResponse
parameter of the transform function is equivalent to the option passed with the request. This allows to distinguish whether just the transformed body or the whole response shall be returned by the transform function:
function reverseBody(body, response, resolveWithFullResponse) {
response.body = response.body.split('').reverse().join('');
return resolveWithFullResponse ? response : response.body;
}
Continuation Local Storage (CLS) is a great mechanism for backpacking data along asynchronous call chains that is best explained in these slides. If you want to use CLS you need to install the continuation-local-storage package. Request-Promise internally uses the cls-bluebird package to enable CLS also within the Bluebird promises.
Just call rp.bindCLS(ns)
ONCE before your first request to activate CLS:
var rp = require('request-promise');
var cls = require('continuation-local-storage');
var ns = cls.createNamespace('testNS');
rp.bindCLS(ns);
ns.run(function () {
ns.set('value', 'hi');
rp('http://google.com')
.then(function () {
console.log(ns.get('value')); // -> hi
});
});
Since the cls-bluebird package currently is just a quick and dirty implementation the CLS support is only experimental.
The ways to debug the operation of Request-Promise are the same as described for Request. These are:
NODE_DEBUG=request node script.js
(lib,request,otherlib
works too).require('request-promise').debug = true
at any time (this does the same thing as #1).require('request-debug')(rp);
.Usually you want to mock the whole request function which is returned by require('request-promise')
. This is not possible by using a mocking library like sinon.js alone. What you need is a library that ties into the module loader and makes sure that your mock is returned whenever the tested code is calling require('request-promise')
. Mockery is one of such libraries.
@florianschmidt1994 kindly shared his solution:
before(function (done) {
var filename = "fileForResponse";
mockery.enable({
warnOnReplace: false,
warnOnUnregistered: false,
useCleanCache: true
});
mockery.registerMock('request-promise', function () {
var response = fs.readFileSync(__dirname + '/data/' + filename, 'utf8');
return Bluebird.resolve(response.trim());
});
done();
});
after(function (done) {
mockery.disable();
mockery.deregisterAll();
done();
});
describe('custom test case', function () {
// Test some function/module/... which uses request-promise
// and it will always receive the predefined "fileForResponse" as data, e.g.:
var rp = require('request-promise');
rp(...).then(function(data) {
// ➞ data is what is in fileForResponse
});
});
Based on that you may now build a more sophisticated mock. Sinon.js may be of help as well.
To set up your development environment:
cd
to the main folder,npm install
,npm install gulp -g
if you haven't installed gulp globally yet, andgulp dev
. (Or run node ./node_modules/.bin/gulp dev
if you don't want to install gulp globally.)gulp dev
watches all source files and if you save some changes it will lint the code and execute all tests. The test coverage report can be viewed from ./coverage/lcov-report/index.html
.
If you want to debug a test you should use gulp test-without-coverage
to run all tests without obscuring the code by the test coverage instrumentation.
resolveWithFullResponse = true
is used)
(Thanks to @zcei for proposing the change in issue #58)transform
function by a third resolveWithFullResponse
parametertypeof reason === 'object'
still holds true and the error objects have the same properties as the previous reason objects. If the reject handler only accesses the properties on the reason object - which is usually the case - no migration is required..finally(...)
to allow using it as the first method in the promise chain
(Thanks to @hjpbarcelos for his pull request #28).promise()
method for advanced Bluebird API usage
(Thanks to @devo-tox for his feedback in issue #27)See the LICENSE file for details.
FAQs
The simplified HTTP request client 'request' with Promise support. Powered by Bluebird.
The npm package request-promise receives a total of 1,069,919 weekly downloads. As such, request-promise popularity was classified as popular.
We found that request-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.