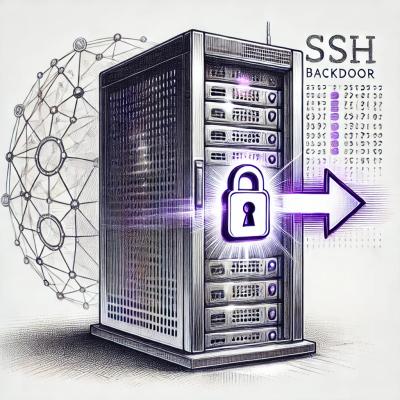
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
resource-client
Advanced tools
Easily create node API clients for your APIs. Inspired by Angular Resource.
npm install resource-client --save
var resourceClient = require('resource-client');
var Product = resourceClient({
url: 'http://www.mysite.com/api/products/:_id',
headers: {
'X-Secret-Token': 'ABCD1234'
}
});
Product.query({isActive: true}, function(err, products) {
product = products[0]
product.name = 'apple'
product.save()
});
products/:name
{_id: '@_id'}
.var resourceClient = require('resource-client');
var Product = resourceClient({
url: 'http://www.mysite.com/api/products/:_id',
params: {_id: '@_id'}
headers: {'X-Secret-Token': 'ABCD1234'}
})
products/:name
{_id: '@_id'}
.var resourceClient = require('resource-client');
var Product = resourceClient({
url: 'http://www.mysite.com/api/products/:_id',
headers: {
'X-Secret-Token': 'ABCD1234'
}
});
Product.action('getActive', {
method: 'GET'
isArray: true
params: {isActive: true}
});
Product.action('queryOne', {
method: 'GET'
isArray: true
returnFirst: true
});
If the method is GET, you can use it as a class method:
Class.method([params], [options], callback)
Product.action('get', {
method: 'GET'
});
// class method
Product.get({_id: 1234}, function (err, product) { ... })
If the method is PUT, POST, or DELETE, you can use it as both a class or an instance method:
Class.method([params], [body], [options], callback)
instance.method([params], [options], callback)
Product.action('save', {
method: 'POST'
});
// class method
Product.save({name: 'apple'}, function (err, product) { ... });
// instance method
product = new Product({name: 'apple'});
product.save(function(err) { ... });
Every new resource will come with these methods by default. However, we recommend you explicitly define an action for each endpoint exposed by your API.
If you need to pass custom request options for an individual request, (for example, to identify a user with an authentication token), the action methods also take optional parameters, like so:
Product.save(
{}, // params
{name: 'apple'}, // body
{ headers: {} }, // options
function (err, product) { ... }
);
Product.getById(urlParams, queryParams, otherOptions, callback);
Please follow our Code of Conduct when contributing to this project.
$ git clone https://github.com/goodeggs/resource-client && cd resource-client
$ npm install
$ npm test
Module scaffold generated by generator-goodeggs-npm.
FAQs
Easily create api clients for your server side resources.
The npm package resource-client receives a total of 30 weekly downloads. As such, resource-client popularity was classified as not popular.
We found that resource-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.