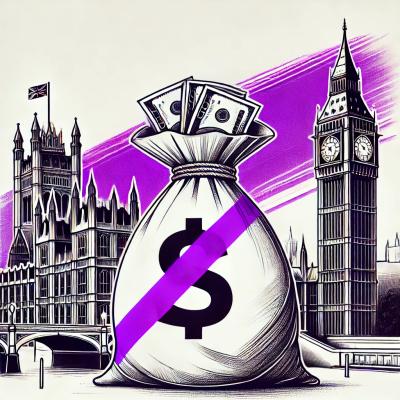
Security News
UK Officials Consider Banning Ransomware Payments from Public Entities
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
rest-client-sdk
Advanced tools
Rest client SDK for API for Javascript usage.
This client tries to avoid the complexity of implementing a custom SDK for every API you have. You just have to implements your model and a little configuration and it will hide the complexity for you.
npm install rest-client-sdk
import { AbstractClient } from 'rest-client-sdk';
class SomeEntityClient extends AbstractClient {
getPathBase() {
return '/v2/some_entities';
}
getName() {
return 'SomeEntity'; // this will be passed to the entity factory
}
}
export default SomeEntityClient;
import { TokenStorage } from 'rest-client-sdk';
const tokenGeneratorConfig = { path: 'oauth.me', foo: 'bar' };
const tokenGenerator = new SomeTokenGenerator(tokenGeneratorConfig); // Some token generators are defined in `src/TokenGenerator/`
const storage = AsyncStorage; // create a storage instance if you are not on RN. In browser and node, localforage works fine
const tokenStorage = new TokenStorage(tokenGenerator, storage);
The token generator is a class implementing generateToken
and refreshToken
.
Those methods must return an array containing an access_token
key.
The storage needs to be a class implementing setItem(key, value)
, getItem(key)
and removeItem(key)
. Those functions must return a promise.
At Mapado we use localforage in a browser environment and React Native AsyncStorage for React Native.
import RestClientSdk from 'rest-client-sdk';
const config = {
path: 'api.me',
scheme: 'https',
port: 443,
segment: '/my-api',
authorizationType: 'Bearer', // default to "Bearer", but can be "Basic" or anything
useDefaultParameters: true,
}; // path and scheme are mandatory
const clients = {
someEntity: SomeEntityClient,
// ...
};
const sdk = new RestClientSdk(tokenStorage, config, clients);
You can now call the clients this way:
sdk.someEntity.find(8); // will find the entity with id 8. ie. /v2/some_entities/8
sdk.someEntity.findAll(); // will find all entities. ie. /v2/some_entities
sdk.someEntity.findBy({ foo: 'bar' }); // will find all entities for the request: /v2/some_entities?foo=bar
You can inject a custom entity factory to the SDK. All entities will be send to the entityFactory.
The default entity factory is the immutable function fromJS
function entityFactory(input, listOrItem, clientName = null) {
if (listOrItem === 'list') {
// do stuff with your list input
return output;
} else { // listOrItem === 'item'
const output = // ... do stuff with your input
return output;
}
}
const sdk = new RestClientSdk(tokenStorage, config, clients, entityFactory);
FAQs
Rest Client SDK for API
The npm package rest-client-sdk receives a total of 12 weekly downloads. As such, rest-client-sdk popularity was classified as not popular.
We found that rest-client-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.