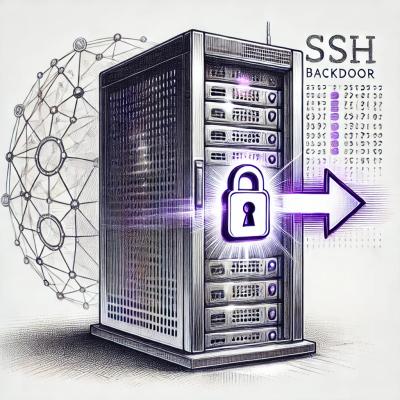
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Regular Expression Transform Stream for Node.js
Create a transform stream which will buffer incoming data and push regex results
when matches can be made, i.e. when regex.exec
returns non-null value. You will probably want to
set the g
flag on regexes most of the time.
const restream = require('restream')
const Readable = require('stream').Readable
const Writable = require('stream').Writable
// your input readable stream which outputs strings
const input = 'test-string-{12345}-{67890}'
const rs = new Readable({
read: () => {
rs.push(input)
rs.push(null)
},
})
// your output writable sream which saves incoming data
const ws = new Writable({ objectMode: true })
const data = []
ws._write = (chunk, _, next) => {
data.push(chunk)
next()
}
const regex = /{(\d+)}/g
const rts = restream(regex)
rs.pipe(rts).pipe(ws)
ws.once('finish', () => {
console.log(data)
})
[ [ '{12345}',
'12345',
index: 12,
input: 'test-string-{12345}-{67890}' ],
[ '{67890}',
'67890',
index: 20,
input: 'test-string-{12345}-{67890}' ] ]
Create a Readable
stream which will make data available
when an incoming chunk has been updated according to the regex
input, which can
be either a single regex object ({ re: /regex/, replacement: 'hello-world' }
),
or an array of such objects. A replacement
can be either a string, or a function,
which will be called by str.replace
const restream = require('restream')
const Catchment = require('catchment')
const Readable = require('stream').Readable
function createReadable() {
const rs = new Readable({
read: () => {
rs.push('Hello {{ name }}, your username is {{ user }}'
+ ' and you have {{ stars }} stars')
rs.push(null)
},
})
return rs
}
const regexes = [{
re: /{{ user }}/,
replacement: 'fred',
}, {
re: /{{ name }}/g,
replacement: 'Fred',
}, {
re: /{{ stars }}/,
replacement: '5',
}]
const stream = restream.replaceStream(regexes)
const rs = createReadable()
rs.pipe(stream)
const catchment = new Catchment()
stream.pipe(catchment)
catchment.promise
.then((res) => {
console.log(res)
})
Output:
Hello Fred, your username is fred and you have 5 stars
You can replace matches using a function. See (MDN) for more documentation.
const restream = require('restream')
const Catchment = require('catchment')
const Readable = require('stream').Readable
function createReadable() {
const rs = new Readable({
read: () => {
rs.push('Hello __Fred__, your username is __fred__'
+ ' and you have __5__ stars')
rs.push(null)
},
})
return rs
}
const regexes = [{
re: /__(\S+)__/g,
replacement: (match, p1) => {
return `<em>${p1}</em>`
},
}]
const stream = restream.replaceStream(regexes)
const rs = createReadable()
rs.pipe(stream)
const catchment = new Catchment()
stream.pipe(catchment)
catchment.promise
.then((res) => {
console.log(res)
})
Output:
Hello <em>Fred</em>, your username is <em>fred</em> and you have <em>5</em> stars
2017, Sobesednik Media
FAQs
Regular Expression Detection & Replacement streams.
We found that restream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.