Introduction
rethinkdb-job-queue
is a persistent job or task queue backed by RethinkDB.
It has been built as an alternative to the many queues available on NPM.

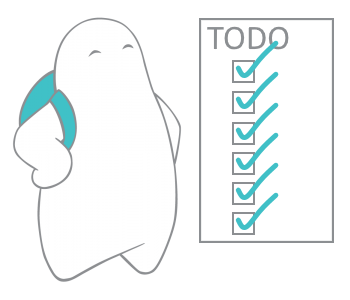

Please Star on GitHub / NPM and Watch for updates.
Features
Documentation
For full documentation please see the wiki
See the Change Log for recent changes.
Project Status
- This
rethinkdb-job-queue
module is fully functional. - There are over 1400 integration tests.
- Updated to v1.0.0 to support SemVer.
Quick Start
Installation
Note: You will need to install RethinkDB before you can use rethinkdb-job-queue
After installing RethinkDB install the job queue using the following command.
npm install rethinkdb-job-queue --save
WARNING: If you are using an older version of Node.js you may need the Babel Pollyfill. The index.js
file points to the dist
directory, which has been transpiled by Babel. Babel only transforms syntax.
Simple Example
const Queue = require('rethinkdb-job-queue')
const qOptions = {
name: 'Mathematics'
}
const cxnOptions = {
db: 'JobQueue',
}
const q = new Queue(cxnOptions, qOptions)
const job = q.createJob({
numerator: 123,
denominator: 456
})
q.process((job, next) => {
try {
let result = job.numerator / job.denominator
return next(result)
} catch (err) {
console.error(err)
return next(err)
}
})
return q.addJob(job).catch((err) => {
console.error(err)
})
E-Mail Job Example using nodemailer
const nodemailer = require('nodemailer')
const transporter = nodemailer.createTransport({
service: 'Mailgun',
auth: {
user: 'postmaster@superheros.com',
pass: 'your-api-key-here'
}
})
var mailOptions = {
from: '"Registration" <support@superheros.com>',
subject: 'Registration',
text: 'Click here to complete your registration',
html: '<b>Click here to complete your registration</b>'
}
const Queue = require('rethinkdb-job-queue')
const qOptions = {
name: 'RegistrationEmail',
masterInterval: 310000,
changeFeed: true,
concurrency: 100,
removeFinishedJobs: 2592000000,
}
const cxnOptions = {
host: 'localhost',
port: 28015,
db: 'JobQueue',
}
const q = new Queue(cxnOptions, qOptions)
q.jobOptions = {
priority: 'normal',
timeout: 300000,
retryMax: 3,
retryDelay: 600000
}
const job = q.createJob()
job.recipient = 'batman@batcave.com'
q.process((job, next) => {
mailOptions.to = job.recipient
return transporter.sendMail(mailOptions).then((info) => {
console.dir(info)
return next(info)
}).catch((err) => {
return next(err)
})
})
return q.addJob(job).then((savedJobs) => {
}).catch((err) => {
console.error(err)
})
About the Owner
I, Grant Carthew, am a technologist, trainer, and Dad from Queensland, Australia. I work on code in a number of personal projects and when the need arises I build my own packages.
This project exists because there were no functional job queues built on the RethinkDB database. I wanted an alternative to the other job queues on NPM.
Everything I do in open source is done in my own time and as a contribution to the open source community.
Contributing
- Fork it!
- Create your feature branch:
git checkout -b my-new-feature
- Commit your changes:
git commit -am 'Add some feature'
- Push to the branch:
git push origin my-new-feature
- Submit a pull request :D
Please see the debugging and testing documents for more detail.
Credits
Thanks to the following marvelous packages and people for their hard work:
This list could go on...
License
MIT