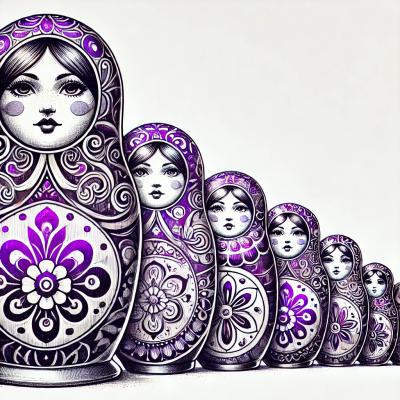
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Rewire is a testing utility for Node.js that allows you to modify the behavior of a module by overriding its private variables and functions. This is particularly useful for unit testing, as it enables you to test private functions and mock dependencies.
Accessing Private Variables
This feature allows you to access private variables within a module. In the code sample, `privateVar` is a private variable in `myModule` that is accessed using `__get__`.
const rewire = require('rewire');
const myModule = rewire('./myModule');
const privateVar = myModule.__get__('privateVar');
console.log(privateVar);
Mocking Private Functions
This feature allows you to mock private functions within a module. In the code sample, `privateFunction` is a private function in `myModule` that is mocked using `__set__`.
const rewire = require('rewire');
const myModule = rewire('./myModule');
myModule.__set__('privateFunction', function() { return 'mocked'; });
console.log(myModule.somePublicFunction());
Mocking Dependencies
This feature allows you to mock dependencies of a module. In the code sample, `dependency` is a dependency of `myModule` that is mocked using `__set__`.
const rewire = require('rewire');
const myModule = rewire('./myModule');
myModule.__set__('dependency', { someMethod: () => 'mocked' });
console.log(myModule.somePublicFunction());
Proxyquire is a module that allows you to override dependencies during testing. Unlike Rewire, which focuses on accessing and modifying private variables and functions, Proxyquire is more about replacing entire dependencies with mocks or stubs.
Sinon is a testing library that provides standalone test spies, stubs, and mocks. While it doesn't allow you to access private variables directly like Rewire, it is very powerful for creating mocks and stubs for functions and objects.
Testdouble is a library for creating test doubles (mocks, stubs, etc.). It focuses on providing a clean and simple API for creating test doubles, but it doesn't provide the ability to access or modify private variables like Rewire.
Dependency injection for node.js applications.
rewire adds a special setter and getter to modules so you can modify their behaviour for better unit testing. You may
rewire does not load the file and eval the contents to emulate node's require mechanism. In fact it uses node's own require to load the module. Thus your module behaves exactly the same in your test environment as under regular circumstances (except your modifications).
Furthermore rewire comes also with support for various client-side bundlers (see below).
npm install rewire
var rewire = require("rewire");
// rewire acts exactly like require.
var myModule = rewire("../lib/myModule.js");
// Just with one difference:
// Your module will now export a special setter and getter for private variables.
myModule.__set__("myPrivateVar", 123);
myModule.__get__("myPrivateVar"); // = 123
// This allows you to mock almost everything within the module e.g. the fs-module.
// Just pass the variable name as first parameter and your mock as second.
myModule.__set__("fs", {
readFile: function (path, encoding, cb) {
cb(null, "Success!");
}
});
myModule.readSomethingFromFileSystem(function (err, data) {
console.log(data); // = Success!
});
// You can set different variables with one call.
myModule.__set__({
fs: fsMock,
http: httpMock,
someOtherVar: "hello"
});
// You may also override globals. These changes are only within the module, so
// you don't have to be concerned that other modules are influenced by your mock.
myModule.__set__({
console: {
log: function () { /* be quiet */ }
},
process: {
argv: ["testArg1", "testArg2"]
}
});
// But be careful, if you do something like this you'll change your global
// console instance.
myModule.__set__("console.log", function () { /* be quiet */ });
// There is another difference to require:
// Every call of rewire() returns a new instance.
rewire("./myModule.js") === rewire("./myModule.js"); // = false
##API
###rewire(filename): rewiredModule
###rewiredModule.__set__(name, value)
var
in the top-leve scope of the module.###rewiredModule.__set__(env)
###rewiredModule.__get__(name): value
Returns the private variable.
##Client-Side Bundlers Since rewire relies heavily on node's require mechanism it can't be used on the client-side without adding special middleware to the bundling process. Currently supported bundlers are:
Please note: Unfortunately the line numbers in stack traces have an offset of +2 (browserify) / +1 (webpack). This is caused by generated code that is added during the bundling process. I'm working on that ... :)
###browserify
var b = browserify(),
bundleSrc;
// Add rewire as browserify middleware
// @see https://github.com/substack/node-browserify/blob/master/doc/methods.markdown#busefn
b.use(require("rewire").bundlers.browserify);
b.addEntry("entry.js");
bundleSrc = b.bundle();
###webpack
var webpackOptions = {
output: "bundle.js"
};
// This function modifies the webpack options object.
// It adds a postLoader and postProcessor to the bundling process.
// @see https://github.com/webpack/webpack#programmatically-usage
require("rewire").bundlers.webpack(webpackOptions);
webpack("entry.js", webpackOptions, function () {});
1.0.1
FAQs
Easy dependency injection for node.js unit testing
The npm package rewire receives a total of 340,684 weekly downloads. As such, rewire popularity was classified as popular.
We found that rewire demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.