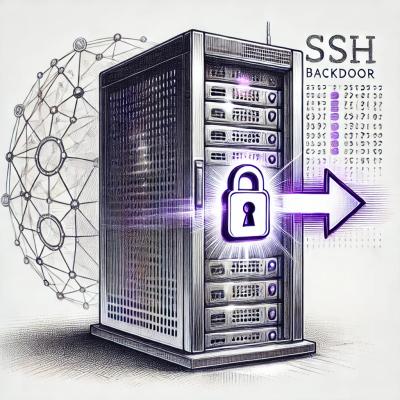
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
rewiremock
Advanced tools
Simple es6-friendly mocking library inspired by the best libraries:
By its nature rewiremock has same behavior as Mockery. But it can have any behavior. It covers any cases.
Rewiremock is an evolution of my way of explorations: the better proxyquire, the way ofresolveQuire, and magic of proxyquire-webpack-alias.
Rewiremock was initially named as mockImports or mockModule. But was renamed for WireMock.
We shall not use that name, but rewire - is one of existing micking library.
see d.ts file, or JSDoc in javascript sources.
default
es6 exportFirst - define your mocks. You can do it in any place, this is just a setup.
import rewiremock from 'rewiremock';
...
// totaly mock `fs` with your stub
rewiremock('fs')
.with({
readFile: yourFunction
});
// replace path, by other module
rewiremock('path')
.by('path-mock');
// replace default export of ES6 module
rewiremock('reactComponent')
.withDefault(MockedComponent)
// replace only part of some library and keep the rest
rewiremock('someLibrary')
.callThought()
.with({
onlyOneMethod
})
Just enable it, and dont forget to disable.
//in mocha tests
beforeEach( () => rewiremock.enable() );
//...
const someModule = require('someModule');
//...
afterEach( () => rewiremock.disable() );
On enable rewiremock will wipe from cache all mocked modules, and all modules which requares them.
Including your test.
On disable it will repeat operation.
All test unrelated modules will be keept. Node modules, react, common files - everything.
As result - it will run faster.
By default - rewiremock has limited features. You can extend it behavior by using plugins.
usual
node.js application. Will absolutize all paths.import rewiremock, { addPlugin } from 'rewiremock';
import webpackAliasPlugin from 'rewiremock/lib/plugins/webpack-alias';
addPlugin(webpackAliasPlugin);
Bad news - you cannot remove plugin, as you cannot remove mocks. Only reset everything. But you should not different setups/plugins in single test.
Each time you require rewiremock - you will get brand new rewiremock.
You cannot declare mocks library
- it will erase itself.
But solution exists, and it is simply -
// require rewiremock in test file
import rewiremock from 'rewiremock';
// require nested one in library file
import rewiremock from 'rewiremock/nested';
// now you can defile dictionary or library of mocks
See _test/nested.spec.js.
Unit testing requires all decencies to be mocked. All! To enable it, run
rewiremock.isolation();
//or
rewiremock.withoutIsolation();
Then active - rewiremock will throw error on require of any unknown module.
Unknown is module which is nor mocked, nor marked as passthrough.
To enable few modules to in invisible
to rewiremock run
rewiremock.passBy(/*pattern or function*/);
rewiremock.passBy(/common/);
rewiremock.passBy(/React/);
rewiremock.passBy(/node_modules/);
rewiremock.passBy((name) => name.indexOf('.node')>=0 )
In most cases you have to:
And it is not a good idea to do it in every test you have.
It is better to have one setup file, which will do everything for you
// this is your test file
// instead of importing original file - import your own one
// import rewiremock from 'rewiremock';
import rewiremock from 'test/rewiremock';
// this tests/rewiremock.js
import rewiremock, { addPlugin, overrideEntryPoint} from 'rewiremock';
// do anything you need
addPlugin(something);
rewiremock('somemodule').with(/*....*/);
// but dont forget to add some magic
overrideEntryPoint(module); // <-- set yourself as top module
// PS: rewiremock will wipe this module from cache to keep magics alive.
export default rewiremock;
Other libraries will always do a strange things with a cache:
Rewiremock uses a bit different, more smart way:
As result - it will never wipe something it should not.
As result - you can mock any file at any level. Sometimes it is usefull.
If you dont want this - just add relative
plugin. It will allow mocking only for modules
required from module with parent equals entryPoint.
PS: module with parent equals entryPoint - any module you require from test(it is an entry point). required from that module - first level require. Simple.
Dont forget - you can write your own plugins. plugin is an object with fields:
{
// to transform name. Used by alias or node.js module
fileNameTransformer: (fileName, parentModule) => fileName;
// check should you wipe module or not. Never used :)
wipeCheck: (stubs, moduleName) => boolean,
// check is mocking allowed for a module. User in relative plugin
shouldMock: (mock, requestFilename, parentModule, entryPoint) => boolean
}
Rewiremock stores some information in magic, unenumerable, constants:
'__esModule' - standard babel one
'__MI_overrideBy' - .by command information
'__MI_allowCallThought' - .callThought command information
'__MI_name' - mock original name
'__MI_module' - original module. If exists due to .callThought or .by commands.
MIT
Happy mocking!
FAQs
Advanced dependency mocking device.
The npm package rewiremock receives a total of 45,162 weekly downloads. As such, rewiremock popularity was classified as popular.
We found that rewiremock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.