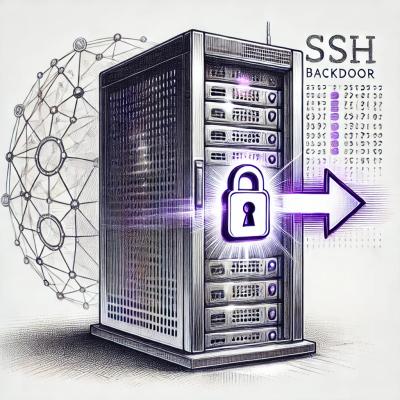
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
rewiremock
Advanced tools
Simple es6-friendly mocking library inspired by the best libraries:
By its nature rewiremock has same behavior as Mockery. But it can behave like others too. It covers any cases. It is the right way to mock your dependencies or perform dependency injection.
Rewiremock is an evolution of lessons I learned from: the better proxyquire, the way of resolveQuire, and magic of proxyquire-webpack-alias.
true
testing experience.see d.ts file, or JSDoc in javascript sources.
default
es6 exportIf something is not working - just check that you:
First - define your mocks. You can do it in any place, this is just a setup.
import rewiremock from 'rewiremock';
...
// totaly mock `fs` with your stub
rewiremock('fs')
.with({
readFile: yourFunction
});
// replace path, by other module
rewiremock('path')
.by('path-mock');
// replace default export of ES6 module
rewiremock('reactComponent')
.withDefault(MockedComponent)
// replace only part of some library and keep the rest
rewiremock('someLibrary')
.callThought()
.with({
onlyOneMethod
})
There is a simply way to do it: Just enable it, and dont forget to disable it later.
//in mocha tests
beforeEach( () => rewiremock.enable() );
//...
// here you will get some advantage in type casting and autocompleting.
// it will actually works...
const someModule = require('someModule');
//...
afterEach( () => rewiremock.disable() );
Once enabled, rewiremock will wipe all mocked modules from cache, and all modules which require them.
Including your test.
Once disabled it will restore everything.
All unrelated to test dependencies will be kept. Node modules, react, common files - everything.
As result - it will run faster.
Sometimes you will have independent tests in a single file, and you might need separate mocks for each one.
inScope
execute callback inside sandbox, and all mocks or plugins or anything else you have added will not leaks away.
rewiremock.inScope( () => {
rewiremock('something').with(something);
rewiremock.enable();
// is 'something' mocked? Yes
....
rewiremock.disable();
// is 'something' mocked? No
// is it still listed as mock? Yes
});
// is 'something' mocked or listed? No
And there is a bit harder way to do it - scope. inScope will create new internal scope, next you can add something new to it, and then it will be destroyed. It will also enable/disable rewiremock just in time.
This helps keep tests in isolation.
PS: scopes are nesting each other like javascript prototypes do.
rewiremock.around(
() => import('somemodule'), // load a module. Using import or require.
// just before it you can specify mocks or anything else
(mock) => {
addPlugin(nodePlugin);
mock('./lib/a/foo').with(() => 'aa');
mock('./lib/a/../b/bar').with(() => 'bb');
mock('./lib/a/../b/baz').with(() => 'cc');
}
) // at this point scope is dead
.then((mockedBaz) => {
expect(mockedBaz()).to.be.equal('aabbcc');
});
or just
rewiremock.around(() => import('somemodule')).then(mockedModule => doSomething)
or
rewiremock.around(
() => import('somemodule').then( mockedModule => doSomething),
(mock) => aPromise
);
Currently .inScope is the only API capable to handle es6 dynamic imports.
By default - rewiremock has limited features. You can extend its behavior via plugins.
usual
node.js application. Will absolutize all paths. Will wipe cache very accurately.import rewiremock, { addPlugin, removePlugin, plugins } from 'rewiremock';
addPlugin(plugins.webpackAlias);
removePlugin(plugins.webpackAlias);
If you import rewiremock from other place, for example to add some defaults mocks - it will not gonna work. Each instance of rewiremock in independent. You have to pass your instance of rewiremock to build a library. PS: note, rewiremock did have nested API, but it were removed.
Unit testing requires all decencies to be mocked. All! To enable it, run
rewiremock.isolation();
//or
rewiremock.withoutIsolation();
Then active - rewiremock will throw error on require of any unknown module.
Unknown is module which is nor mocked, nor marked as pass-through.
To make few modules to be invisible
to rewiremock, run
rewiremock.passBy(/*pattern or function*/);
rewiremock.passBy(/common/);
rewiremock.passBy(/React/);
rewiremock.passBy(/node_modules/);
rewiremock.passBy((name) => name.indexOf('.node')>=0 )
Sometimes you have to be sure, that you mock is actually was called.
Isolation will protect you then you add new dependencies, .toBeUsed
protect you from removal.
In most cases you have to:
And it is not a good idea to do it in every test you have.
It is better to have one setup file, which will do everything for you
// this is your test file
// instead of importing original file - import your own one
// import rewiremock from 'rewiremock';
import rewiremock from 'test/rewiremock';
// this tests/rewiremock.js
import rewiremock, { addPlugin, overrideEntryPoint} from 'rewiremock';
// do anything you need
addPlugin(something);
rewiremock('somemodule').with(/*....*/);
// but don't forget to add some magic
overrideEntryPoint(module); // <-- set yourself as top module
// PS: rewiremock will wipe this module from cache to keep magic alive.
export default rewiremock;
Other libraries will always do strange things with cache:
Rewiremock is using a bit different, smarter way:
As a result - it will not wipe things it should not wipe.
As a result - you can mock any file at any level. Sometimes it is useful.
If you don't want this - just add relative
plugin. It will allow mocking only for modules
required from module with parent equals entryPoint.
PS: module with parent equals entryPoint - any module you require from test(it is an entry point). required from that module - first level require. Simple.
Don't forget - you can write your own plugins. plugin is an object with fields:
{
// to transform name. Used by alias or node.js module
fileNameTransformer: (fileName, parentModule) => fileName;
// check should you wipe module or not. Never used :)
wipeCheck: (stubs, moduleName) => boolean,
// check is mocking allowed for a module. User in relative plugin
shouldMock: (mock, requestFilename, parentModule, entryPoint) => boolean
}
MIT
Happy mocking!
FAQs
Advanced dependency mocking device.
The npm package rewiremock receives a total of 45,162 weekly downloads. As such, rewiremock popularity was classified as popular.
We found that rewiremock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.