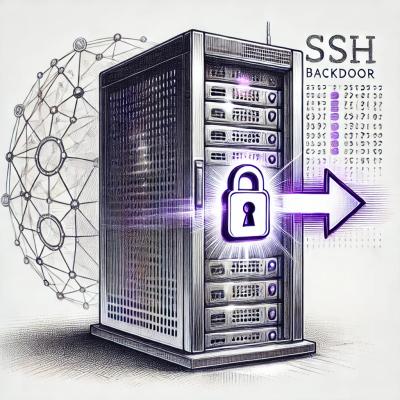
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
rewiremock
Advanced tools
Simple es6-friendly mocking library inspired by the best libraries:
By its nature rewiremock has same behavior as Mockery. But it can behave like others too. It covers any cases. It is the right way to mock your dependencies or perform dependency injection.
Rewiremock is an evolution of lessons I learned from: the better proxyquire, the way of resolveQuire, and magic of proxyquire-webpack-alias.
true
testing experience.I have wrote some articles about these ideas - https://medium.com/tag/rewiremock/latest
default
es6 exportYep - there is 4 top level ways to activate a mock - inScope, around, proxy or just enable.
Which one to choose? Any! It just depends:
#Usage
// 1. proxy will load a file by it's own ( keep in mind - name resolution is a complex thing)
const mock = rewiremock.proxy('somemodule', (r) => ({
'dep1': { name: 'override' },
'dep2': r.with({name: 'override' }).toBeUsed().directChildOnly() // use all `mocking API`
}));
// 2. you can require a file by yourself. ( yep, proxy is a god function)
const mock = rewiremock.proxy(() => require('somemodule'), {
'dep1': { name: 'override' },
'dep2': { onlyDump: 'stubs' }
}));
// 3. or use es6 import (not for node.js mjs `real` es6 modules)
// PS: module is an async version of proxy, so you can use imports
const mock = await rewiremock.module(() => import('somemodule'), {
'dep1': { name: 'override' },
'dep2': { onlyDump: 'stubs' }
}));
// 3. another version of .module, where you can do just ~anything~.
const mock = await rewiremock.around(() => import('somemodule'), () => {
rewiremock('dep1').with('something');
callMom();
}));
// 4. Low level API
rewiremock('someThing').with('someThingElse')
rewiremock.enable();
rewiremock.disable();
Rewiremock is able to provide a type-safe mocks. To enable type-safety follow these steps:
// @flow
import rewiremock from 'rewiremock';
rewiremock.around(
() => import('./a.js'),
mock => {
mock(() => import('./b.js'))
.withDefault(() => "4")
.with({testB: () => 10})
.nonStrict() // turn off type system
.with({ absolutely: "anything" })
}
);
If default export is not exists on module 'b', or there is no named export testB, or types do not match - type system will throw.
If you will declare an async mock, you it will not be resolved by the time of execution - Rewiremock will throw on Error.
If you have async imports inside mocked file, follow this syntax
rewiremock.around(
() => import('./a.js'),
mock => {
// just before loader function rewiremock enabled itself
mock(() => import('./b.js').then(mock=>mock)) // mocks `live` one `tick` more
// just after loader function resolved rewiremock disables itself
mock => {
....
}
}
);
Just use it. You can also activate nodejs, which will double check all modules names on a real FS, but.. everything might work out of the box.
PS: Just use usedByDefault to ensure module names are resolved correctly.
Rewiremock can emulate
few webpack features(like aliases) in node.js environment, but it also can be run inside webpack.
Actually rewiremock is the first client side mocking library
But not so fast, hanny. First you have to have 3(!) Plugins enabled.
plugins: [
new webpack.NamedModulesPlugin(),
new webpack.HotModuleReplacementPlugin(),
new (require("rewiremock/webpack/plugin"))()
]
That's all. Now all magic will happens at client side.
It is better to use .proxy/module command with direct require/import and leave all names conversion to webpack.
First - define your mocks. You can do it in any place, this is just a setup.
import rewiremock from 'rewiremock';
...
// totaly mock `fs` with your stub
rewiremock('fs')
.with({
readFile: yourFunction
});
// replace path, by other module
rewiremock('path')
.by('path-mock');
// replace enzyme by preconfigured one (from https://medium.com/airbnb-engineering/unlocking-test-performance-migrating-from-mocha-to-jest-2796c508ec50)
rewiremock('enzyme')
.by(({requireActual}) => {
// see rest of possible params in d.ts file
const enzyme = requireActual('enzyme');
if (!mockSetup) {
const chai = requireActual('chai');
const chaiEnzyme = requireActual('chai-enzyme');
chai.use(chaiEnzyme());
}
return enzyme;
});
// replace default export of ES6 module
rewiremock('reactComponent')
.withDefault(MockedComponent)
// replace only part of some library and keep the rest
rewiremock('someLibrary')
.callThrough()
.with({
onlyOneMethod
})
// secure yourself and from 'unexpected' mocks
rewiremock('myDep')
.with(mockedDep)
.calledFromMock()
There is a simply way to do it: Just enable it, and dont forget to disable it later.
//in mocha tests
beforeEach( () => rewiremock.enable() );
//...
// here you will get some advantage in type casting and autocompleting.
// it will actually works...
const someModule = require('someModule');
//...
afterEach( () => rewiremock.disable() );
Once enabled, rewiremock will wipe all mocked modules from cache, and all modules which require them.
Including your test.
Once disabled it will restore everything.
All unrelated to test dependencies will be kept. Node modules, react, common files - everything.
As result - it will run faster.
Sometimes you will have independent tests in a single file, and you might need separate mocks for each one.
inScope
execute callback inside sandbox, and all mocks or plugins or anything else you have added will not leaks away.
rewiremock.inScope( () => {
rewiremock('something').with(something);
rewiremock.enable();
// is 'something' mocked? Yes
....
rewiremock.disable();
// is 'something' mocked? No
// is it still listed as mock? Yes
});
// is 'something' mocked or listed? No
And there is a bit harder way to do it - scope. inScope will create new internal scope, next you can add something new to it, and then it will be destroyed. It will also enable/disable rewiremock just in time.
This helps keep tests in isolation.
PS: scopes are nesting each other like javascript prototypes do.
rewiremock.around(
() => import('somemodule'), // load a module. Using import or require.
// just before it you can specify mocks or anything else
(mock) => {
addPlugin(nodePlugin);
mock('./lib/a/foo').with(() => 'aa');
mock('./lib/a/../b/bar').with(() => 'bb');
mock('./lib/a/../b/baz').with(() => 'cc');
}
) // at this point scope is dead
.then((mockedBaz) => {
expect(mockedBaz()).to.be.equal('aabbcc');
});
or just
rewiremock.around(() => import('somemodule')).then(mockedModule => doSomething)
or
rewiremock.around(
() => import('somemodule').then( mockedModule => doSomething),
(mock) => aPromise
);
Currently .inScope is the only API capable to handle es6(not node [m]js!) dynamic imports.
Sometimes it is much easier to combine all the things together.
// preferred way - crete stubs using a function, where R is mock creator
rewiremock.proxy('somemodule', (r) => ({
'dep1': { name: 'override' },
'dep2': r.with({name: 'override' }).toBeUsed().directChildOnly() // same powerfull syntax
}));
// straight way - just provide stubs.
rewiremock.proxy('somemodule', {
'dep1': { name: 'override' },
'dep2': { name: 'override' }
}));
By default - rewiremock has limited features. You can extend its behavior via plugins.
usual
node.js application. Will absolutize all paths. Will wipe cache very accurately.import rewiremock, { addPlugin, removePlugin, plugins } from 'rewiremock';
addPlugin(plugins.webpackAlias);
removePlugin(plugins.webpackAlias);
If you import rewiremock from other place, for example to add some defaults mocks - it will not gonna work. Each instance of rewiremock in independent. You have to pass your instance of rewiremock to build a library. PS: note, rewiremock did have nested API, but it were removed.
Unit testing requires all decencies to be mocked. All! To enable it, run
rewiremock.isolation();
//or
rewiremock.withoutIsolation();
Then active - rewiremock will throw error on require of any unknown module.
Unknown is module which is nor mocked, nor marked as pass-through.
To make few modules to be invisible
to rewiremock, run
rewiremock.passBy(/*pattern or function*/);
rewiremock.passBy(/common/);
rewiremock.passBy(/React/);
rewiremock.passBy(/node_modules/);
rewiremock.passBy((name) => name.indexOf('.node')>=0 )
Sometimes you have to be sure, that you mock is actually was called.
Isolation will protect you then you add new dependencies, .toBeUsed
protect you from removal.
Jest is a very popular testing framework, but it has one issue - is already contain mocking support.
To use rewiremock with Jest add to the beginning of your file
// better to disable automock
jest.disableAutomock();
// Jest breaks the rules, and you have to restore nesting of a modules.
rewiremock.overrideEntryPoint(module);
// There is no way to use overload by Jest require or requireActual.
// use the version provided by rewiremock.
require = rewiremock.requireActual;
!!! the last line here may disable Jest sandboxing. !!!
It is better just to use rewiremock.requireActual
, without overriding global require.
In most cases you have to:
And it is not a good idea to do it in every test you have.
It is better to have one setup file, which will do everything for you
// this is your test file
// instead of importing original file - import your own one
// import rewiremock from 'rewiremock';
import rewiremock from 'test/rewiremock';
// this tests/rewiremock.js
import rewiremock, { addPlugin, overrideEntryPoint} from 'rewiremock';
// do anything you need
addPlugin(something);
rewiremock('somemodule').with(/*....*/);
// but don't forget to add some magic
overrideEntryPoint(module); // <-- set yourself as top module
// PS: rewiremock will wipe this module from cache to keep magic alive.
export default rewiremock;
Other libraries will always do strange things with cache:
Rewiremock is using a bit different, smarter way:
As a result - it will not wipe things it should not wipe.
As a result - you can mock any file at any level. Sometimes it is useful.
If you don't want this - just add relative
plugin. It will allow mocking only for modules
required from module with parent equals entryPoint.
PS: module with parent equals entryPoint - any module you require from test(it is an entry point). required from that module - first level require. Simple.
Don't forget - you can write your own plugins. plugin is an object with fields:
{
// to transform name. Used by alias or node.js module
fileNameTransformer: (fileName, parentModule) => fileName;
// check should you wipe module or not. Never used :)
wipeCheck: (stubs, moduleName) => boolean,
// check is mocking allowed for a module. User in relative plugin
shouldMock: (mock, requestFilename, parentModule, entryPoint) => boolean
}
If something is not working - just check that you:
added a plugin to transform names (nodejs, webpackAlias or relative)
use .toBeUsed for each mocks And they actually were mocked. If not - rewiremock will throw an Error.
MIT
Happy mocking!
FAQs
Advanced dependency mocking device.
The npm package rewiremock receives a total of 45,162 weekly downloads. As such, rewiremock popularity was classified as popular.
We found that rewiremock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.