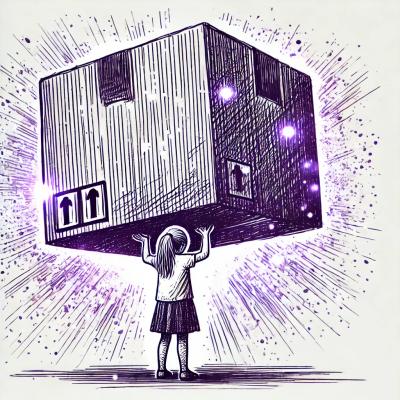
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Rework is a plugin framework for CSS preprocessing. It allows you to manipulate CSS using JavaScript, providing a way to transform stylesheets with various plugins.
CSS Parsing
Rework can parse CSS strings into an Abstract Syntax Tree (AST), which can then be manipulated programmatically.
const rework = require('rework');
const css = 'body { color: red; }';
const ast = rework(css).toString();
console.log(ast);
CSS Transformation
Rework allows you to transform CSS using plugins. For example, the rework-npm plugin lets you import CSS from npm packages.
const rework = require('rework');
const reworkNPM = require('rework-npm');
const css = '@import "normalize.css";';
const output = rework(css).use(reworkNPM()).toString();
console.log(output);
Vendor Prefixing
Rework can automatically add vendor prefixes to CSS properties using plugins like rework-plugin-prefix.
const rework = require('rework');
const reworkPrefix = require('rework-plugin-prefix');
const css = 'body { display: flex; }';
const output = rework(css).use(reworkPrefix('webkit')).toString();
console.log(output);
PostCSS is a tool for transforming CSS with JavaScript plugins. It is more modern and widely used compared to Rework, offering a larger ecosystem of plugins and better performance.
Less is a CSS pre-processor that extends the CSS language, adding features like variables, mixins, and functions. Unlike Rework, Less is a full-fledged pre-processor rather than a plugin framework.
Sass is another CSS pre-processor that provides advanced features like variables, nested rules, and mixins. Sass is more feature-rich and has a larger community compared to Rework.
CSS manipulations built on css, allowing you to automate vendor prefixing, create your own properties, inline images, anything you can imagine! Also works in the browser as a component.
with node:
$ npm install rework
or in the browser:
$ component install visionmedia/rework
Usage: rework [options]
Options:
-h, --help output usage information
-V, --version output the version number
-v, --vendors <list> specify list of vendors
-e, --ease add additional easing functions
--vars add css variable support
for example:
$ rework -v webkit,moz < my.css > my.reworked.css
Return a new Rework
instance for the given string of css
.
Define vendor prefixes
that plugins may utilize,
however most plugins do and should accept direct passing
of vendor prefixes as well.
Use the given plugin fn
. A rework "plugin" is simply
a function accepting the stylesheet object and Rework
instance,
view the definitions in ./lib/plugins
for examples.
Return the string representation of the manipulated css. Optionally
you may compress the output with .toString({ compress: true })
The following plugins are bundled with rework
:
url()
s with a callback functionrgba(#fc0, .5)
height: @width
etcextend: selector
supportAdd support for extending existing rulesets:
button {
padding: 5px 10px;
border: 1px solid #eee;
border-bottom-color: #ddd;
}
.green {
background: green;
padding: 10px 15px
}
a.join {
extend: button;
extend: .green;
}
a.button
input[type='submit'],
input[type='button'] {
extend: button
}
yields:
button,
a.button,
input[type='submit'],
input[type='button'],
a.join {
padding: 5px 10px;
border: 1px solid #eee;
border-bottom-color: #ddd;
}
.green,
a.join {
background: green;
padding: 10px 15px
}
Define media macros with the given obj
.
For example define two contrived custom media types, "phone" and "phone-landscape":
style.use(rework.media({
'phone': 'only screen and (min-device-width : 320px) and (max-device-width : 480px)',
'phone-landscape': 'only screen and (min-width : 321px)'
}))
@media phone {
body {
background: 'green'
}
}
@media phone-landscape {
body {
background: 'red'
}
}
yields:
@media only screen and (min-device-width : 320px) and (max-device-width : 480px) {
body {
background: 'green'
}
}
@media only screen and (min-width : 321px) {
body {
background: 'red'
}
}
Adds the following list of additional easing functions:
ease-in-out-back
-- cubic-bezier(0.680, -0.550, 0.265, 1.550)
ease-in-out-circ
-- cubic-bezier(0.785, 0.135, 0.150, 0.860)
ease-in-out-expo
-- cubic-bezier(1.000, 0.000, 0.000, 1.000)
ease-in-out-sine
-- cubic-bezier(0.445, 0.050, 0.550, 0.950)
ease-in-out-quint
-- cubic-bezier(0.860, 0.000, 0.070, 1.000)
ease-in-out-quart
-- cubic-bezier(0.770, 0.000, 0.175, 1.000)
ease-in-out-cubic
-- cubic-bezier(0.645, 0.045, 0.355, 1.000)
ease-in-out-quad
-- cubic-bezier(0.455, 0.030, 0.515, 0.955)
ease-out-back
-- cubic-bezier(0.175, 0.885, 0.320, 1.275)
ease-out-circ
-- cubic-bezier(0.075, 0.820, 0.165, 1.000)
ease-out-expo
-- cubic-bezier(0.190, 1.000, 0.220, 1.000)
ease-out-sine
-- cubic-bezier(0.390, 0.575, 0.565, 1.000)
ease-out-quint
-- cubic-bezier(0.230, 1.000, 0.320, 1.000)
ease-out-quart
-- cubic-bezier(0.165, 0.840, 0.440, 1.000)
ease-out-cubic
-- cubic-bezier(0.215, 0.610, 0.355, 1.000)
ease-out-quad
-- cubic-bezier(0.250, 0.460, 0.450, 0.940)
ease-in-back
-- cubic-bezier(0.600, -0.280, 0.735, 0.045)
ease-in-circ
-- cubic-bezier(0.600, 0.040, 0.980, 0.335)
ease-in-expo
-- cubic-bezier(0.950, 0.050, 0.795, 0.035)
ease-in-sine
-- cubic-bezier(0.470, 0.000, 0.745, 0.715)
ease-in-quint
-- cubic-bezier(0.755, 0.050, 0.855, 0.060)
ease-in-quart
-- cubic-bezier(0.895, 0.030, 0.685, 0.220)
ease-in-cubic
-- cubic-bezier(0.550, 0.055, 0.675, 0.190)
ease-in-quad
-- cubic-bezier(0.550, 0.085, 0.680, 0.530)
To view them online visit easings.net.
Add retina support for images, with optional vendor
prefixes,
defaulting to .vendors()
.
.logo {
background-image: url('component.png');
width: 289px;
height: 113px
}
yields:
.logo {
background-image: url('component.png');
width: 289px;
height: 113px
}
@media all and (-webkit-min-device-pixel-ratio: 1.5) {
.logo {
background-image: url("component@2x.png");
background-size: contain
}
}
Prefix property
or array of properties
with optional vendors
defaulting to .vendors()
.
.button {
border-radius: 5px;
}
yields:
.button {
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
border-radius: 5px;
}
Prefix value
with optional vendors
defaulting to .vendors()
.
button {
transition: height, transform 2s, width 0.3s linear;
}
yields:
button {
-webkit-transition: height, -webkit-transform 2s, width 0.3s linear;
-moz-transition: height, -moz-transform 2s, width 0.3s linear;
transition: height, transform 2s, width 0.3s linear
}
This works with other values as well, such as gradients. For example:
.use(rework.prefixValue('linear-gradient'))
.use(rework.prefixValue('radial-gradient'))
button {
background: linear-gradient(#eee, #ddd);
}
button.round {
border-radius: 50%;
background-image: radial-gradient(#cde6f9, #81a8cb);
}
body {
background: -webkit-linear-gradient(#fff, #eee);
}
yields:
button {
background: -webkit-linear-gradient(#eee, #ddd);
background: -moz-linear-gradient(#eee, #ddd);
background: linear-gradient(#eee, #ddd)
}
button.round {
border-radius: 50%;
background-image: -webkit-radial-gradient(#cde6f9, #81a8cb);
background-image: -moz-radial-gradient(#cde6f9, #81a8cb);
background-image: radial-gradient(#cde6f9, #81a8cb)
}
body {
background: -webkit-linear-gradient(#fff, #eee)
}
Prefix selectors with the given string
.
h1 {
font-weight: bold;
}
a {
text-decoration: none;
color: #ddd;
}
yields:
#dialog h1 {
font-weight: bold;
}
#dialog a {
text-decoration: none;
color: #ddd;
}
Add IE opacity support.
ul {
opacity: 1 !important;
}
yields:
ul {
opacity: 1 !important;
-ms-filter: progid:DXImageTransform.Microsoft.Alpha(Opacity=100) !important;
filter: alpha(opacity=100) !important
}
Map url()
calls, useful for inlining images as data-uris, converting
relative paths to absolute etc.
function rewrite(url) {
return 'http://example.com' + url;
}
rework(str)
.use(rework.url(rewrite))
.toString()
Add user-defined mixins, functions that are invoked for a given property, and passed the value. Returning an object that represents one or more properties.
For example the following overflow
mixin allows the designer
to utilize overflow: ellipsis;
to automatically assign associated
properties preventing wrapping etc.
var css = rework(css)
.use(rework.mixin({ overflow: ellipsis }))
.toString()
function ellipsis(type) {
if ('ellipsis' == type) {
return {
'white-space': 'nowrap',
'overflow': 'hidden',
'text-overflow': 'ellipsis'
}
}
return type;
}
Mixins in use look just like regular CSS properties:
h1 {
overflow: ellipsis;
}
yields:
h1 {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis
}
Add property reference support.
button {
width: 120px;
}
button.round {
width: 50px;
height: @width;
line-height: @height;
background-size: @width @height;
}
yields:
button {
width: 120px
}
button.round {
width: 50px;
height: 50px;
line-height: 50px;
background-size: 50px 50px
}
Add variable support. Note that this does not cascade like the CSS variable spec does, thus this is not some sort of fallback mechanism, just a useful feature.
:root {
var-header-color: #06c;
var-main-color: #c06;
}
div {
var-accent-background: linear-gradient(to top, var(main-color), white);
}
h1 {
background-color: var(header-color);
}
.content {
background: var(accent-background) !important;
}
yields:
:root {
var-header-color: #06c;
var-main-color: #c06
}
div {
var-accent-background: linear-gradient(to top, #c06, white)
}
h1 {
background-color: #06c
}
.content {
background: linear-gradient(to top, #c06, white) !important
}
Add color manipulation helpers such as rgba(#fc0, .5)
.
button {
background: rgba(#ccc, .5);
}
yields:
button {
background: rgba(204, 204, 204, .5);
}
Prefix @keyframes with vendors
defaulting to .vendors()
.
Ordering with .keyframes()
is important, as other plugins
may traverse into the newly generated rules, for example the
following will allow .prefix()
to prefix keyframe border-radius
property, .prefix()
is also smart about which keyframes definition
it is within, and will not add extraneous vendor definitions.
var css = rework(read('examples/keyframes.css', 'utf8'))
.vendors(['-webkit-', '-moz-'])
.use(rework.keyframes())
.use(rework.prefix('border-radius'))
.toString()
@keyframes animation {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
yields:
@keyframes animation {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
@-webkit-keyframes animation {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
example.js:
var rework = require('rework')
, read = require('fs').readFileSync
, str = read('example.css', 'utf8');
var css = rework(str)
.vendors(['-webkit-', '-moz-'])
.use(rework.keyframes())
.use(rework.prefix('border-radius'))
.toString()
console.log(css);
example.css:
@keyframes animation {
from { opacity: 0; border-radius: 5px }
to { opacity: 1; border-radius: 5px }
}
stdout:
@keyframes animation {
from {
opacity: 0;
border-radius: 5px
}
to {
opacity: 1;
border-radius: 5px
}
}
@-webkit-keyframes animation {
from {
opacity: 0;
-webkit-border-radius: 5px;
border-radius: 5px
}
to {
opacity: 1;
-webkit-border-radius: 5px;
border-radius: 5px
}
}
@-moz-keyframes animation {
from {
opacity: 0;
-moz-border-radius: 5px;
border-radius: 5px
}
to {
opacity: 1;
-moz-border-radius: 5px;
border-radius: 5px
}
}
Suppose for example you wanted to create your own properties for positions, allowing you to write them as follows:
#logo {
absolute: top left;
}
#logo {
relative: top 5px left;
}
#logo {
fixed: top 5px left 10px;
}
yielding:
#logo {
position: absolute;
top: 0;
left: 0
}
#logo {
position: relative;
top: 5px;
left: 0
}
#logo {
position: fixed;
top: 5px;
left: 10px
}
This is how you could define the plugin:
var rework = require('rework')
, read = require('fs').readFileSync;
function positions() {
var positions = ['absolute', 'relative', 'fixed'];
return function(style){
style.rules.forEach(function(rule){
rule.declarations.forEach(function(decl, i){
if (!~positions.indexOf(decl.property)) return;
var args = decl.value.split(/\s+/);
var arg, n;
// remove original
rule.declarations.splice(i, 1);
// position prop
rule.declarations.push({
property: 'position',
value: decl.property
});
// position
while (args.length) {
arg = args.shift();
n = parseFloat(args[0]) ? args.shift() : 0;
rule.declarations.push({
property: arg,
value: n
});
}
});
});
}
}
var css = rework(read('positions.css', 'utf8'))
.use(positions())
.toString()
console.log(css);
MIT
FAQs
Plugin framework for CSS preprocessing
The npm package rework receives a total of 760,106 weekly downloads. As such, rework popularity was classified as popular.
We found that rework demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.