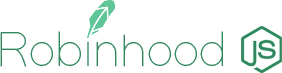

NodeJS Framework to make trades with the private Robinhood API. Using this API is not encouraged, since it's not officially available and it has been reverse engineered.
See @Sanko's Unofficial Documentation for more information.
FYI Robinhood's Terms and Conditions
- Features
- Installation
- Usage
- API
auth_token()
expire_token(callback)
investment_profile(callback)
instruments(symbol, callback)
quote_data(stock, callback) // Not authenticated
accounts(callback)
user(callback)
dividends(callback)
earnings(option, callback)
orders(options, callback)
positions(callback)
nonzero_positions(callback)
place_buy_order(options, callback)
place_sell_order(options, callback)
fundamentals(symbol, callback)
cancel_order(order, callback)
watchlists(name, callback)
create_watch_list(name, callback)
sp500_up(callback)
sp500_down(callback)
splits(instrument, callback)
historicals(symbol, intv, span, callback)
url(url, callback)
news(symbol, callback)
tag(tag, callback)
popularity(symbol, callback)
options_positions
get_currency_pairs
get_crypto
options_orders
- Contributors
Features
- Quote Data
- Buy, Sell Orders
- Daily Fundamentals
- Daily, Weekly, Monthly Historicals
Tested on the latest versions of Node 6, 7 & 8.
Installation
$ npm install robinhood --save
Usage
To authenticate, you can either use your username and password to the Robinhood app or a previously authenticated Robinhood api token:
Robinhood API Auth Token
var credentials = {
token: ''
};
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.quote_data('GOOG', function(error, response, body) {
if (error) {
console.error(error);
process.exit(1);
}
console.log(body);
});
});
Username & Password
This type of login may have been deprecated in favor of the API Token above.
var credentials = {
username: '',
password: ''
};
MFA code
var Robinhood = robinhood({
username : '',
password : ''
}, (err, data) => {
if(err) {
console.log(err);
} else {
if (data && data.mfa_required) {
var mfa_code = '123456';
Robinhood.set_mfa_code(mfa_code, () => {
console.log(Robinhood.auth_token());
});
}
else {
console.log(Robinhood.auth_token());
}
}
});
API
Before using these methods, make sure you have initialized Robinhood using the snippet above.
auth_token()
Get the current authenticated Robinhood api authentication token
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function(err, data){
console.log(Robinhood.auth_token());
}
expire_token()
Expire the current authenticated Robinhood api token (logout).
NOTE: After expiring a token you will need to reinstantiate the package with username & password in order to get a new token!
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.expire_token(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("Successfully logged out of Robinhood and expired token.");
}
})
});
investment_profile(callback)
Get the current user's investment profile.
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.investment_profile(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("investment_profile");
console.log(body);
}
})
});
instruments(symbol, callback)
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.instruments('AAPL',function(err, response, body){
if(err){
console.error(err);
}else{
console.log("instruments");
console.log(body);
}
})
});
Get the user's instruments for a specified stock.
quote_data(stock, callback) // Not authenticated
Get the user's quote data for a specified stock.
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.quote_data('AAPL', function(err, response, body){
if(err){
console.error(err);
}else{
console.log("quote_data");
console.log(body);
}
})
});
accounts(callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.accounts(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("accounts");
console.log(body);
}
})
});
Get the user's accounts.
user(callback)
Get the user information.
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.user(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("user");
console.log(body);
}
})
});
dividends(callback)
Get the user's dividends information.
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.dividends(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("dividends");
console.log(body);
}
})
});
earnings(option, callback)
Get the earnings information. Option should be one of:
let option = { range: X }
OR
let option = { instrument: URL }
OR
let option = { symbol: SYMBOL }
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.earnings(option, function(err, response, body){
if(err){
console.error(err);
}else{
console.log("earnings");
console.log(body);
}
})
});
orders(options, callback)
Get the user's orders information.
Retreive a set of orders
Send options hash (optional) to limit to specific instrument and/or earliest date of orders.
let options = {
updated_at: '2017-08-25',
instrument: 'https://api.robinhood.com/instruments/df6c09dc-bb4f-4495-8c59-f13e6eb3641f/'
}
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.orders(options, function(err, response, body){
if(err){
console.error(err);
}else{
console.log("orders");
console.log(body);
}
})
});
Retreive a particular order
Send the id of the order to retreive the data for a specific order.
let order_id = "string_identifier";
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.orders(order_id, function(err, response, body){
if(err){
console.error(err);
}else{
console.log("order");
console.log(body);
}
})
});
positions(callback)
Get the user's position information.
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.positions(function(err, response, body){
if (err){
console.error(err);
}else{
console.log("positions");
console.log(body);
}
});
});
nonzero_positions(callback)
Get the user's nonzero position information only.
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.nonzero_positions(function(err, response, body){
if (err){
console.error(err);
}else{
console.log("positions");
console.log(body);
}
});
});
place_buy_order(options, callback)
Place a buy order on a specified stock.
var Robinhood = require('robinhood')(credentials, function(err, data){
var options = {
type: 'limit',
quantity: 1,
bid_price: 1.00,
instrument: {
url: String,
symbol: String
}
}
Robinhood.place_buy_order(options, function(error, response, body){
if(error){
console.error(error);
}else{
console.log(body);
}
})
});
For the Optional ones, the values can be:
[Disclaimer: This is an unofficial API based on reverse engineering, and the following option values have not been confirmed]
trigger
A trade trigger is usually a market condition, such as a rise or fall in the price of an index or security.
Values can be:
gfd
: Good For Day
gtc
: Good Till Cancelled
oco
: Order Cancels Other
time
The time in force for an order defines the length of time over which an order will continue working before it is canceled.
Values can be:
immediate
: The order will be cancelled unless it is fulfilled immediately.
day
: The order will be cancelled at the end of the trading day.
place_sell_order(options, callback)
Place a sell order on a specified stock.
var Robinhood = require('robinhood')(credentials, function(err, data){
var options = {
type: 'limit',
quantity: 1,
bid_price: 1.00,
instrument: {
url: String,
symbol: String
},
}
Robinhood.place_sell_order(options, function(error, response, body){
if(error){
console.error(error);
}else{
console.log(body);
}
})
});
For the Optional ones, the values can be:
[Disclaimer: This is an unofficial API based on reverse engineering, and the following option values have not been confirmed]
trigger
A trade trigger is usually a market condition, such as a rise or fall in the price of an index or security.
Values can be:
gfd
: Good For Day
gtc
: Good Till Cancelled
oco
: Order Cancels Other
time
The time in force for an order defines the length of time over which an order will continue working before it is canceled.
Values can be:
immediate
: The order will be cancelled unless it is fulfilled immediately.
day
: The order will be cancelled at the end of the trading day.
fundamentals(symbol, callback)
Get fundamental data about a symbol.
Response
An object containing information about the symbol:
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.fundamentals("SBPH", function(error, response, body){
if(error){
console.error(error);
}else{
console.log(body);
}
})
});
cancel_order(order, callback)
Cancel an order with the order object
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.orders(function(error, response, body){
if(error){
console.error(error);
}else{
var orderToCancel = body.results[0];
Robinhood.cancel_order(orderToCancel, function(err, response, body){
if(err){
console.error(err);
}else{
console.log("Cancel Order Successful");
console.log(body)
}
})
}
})
})
Cancel an order by order id
var order_id = 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.cancel_order(order_id, function(err, response, body){
if(err){
console.error(err);
}else{
console.log("Cancel Order Successful");
console.log(body)
}
})
})
watchlists(name, callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.watchlists(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("got watchlists");
console.log(body);
}
})
});
create_watch_list(name, callback)
//Your account type must support multiple watchlists to use this endpoint otherwise will get { detail: 'Request was throttled.' } and watchlist is not created.
Robinhood.create_watch_list('Technology', function(err, response, body){
if(err){
console.error(err);
}else{
console.log("created watchlist");
console.log(body);
// {
// "url": "https://api.robinhood.com/watchlists/Technology/",
// "user": "https://api.robinhood.com/user/",
// "name": "Technology"
// }
}
})
sp500_up(callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.sp500_up(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("sp500_up");
console.log(body);
}
})
});
sp500_down(callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.sp500_down(function(err, response, body){
if(err){
console.error(err);
}else{
console.log("sp500_down");
console.log(body);
}
})
});
splits(instrument, callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.splits("7a3a677d-1664-44a0-a94b-3bb3d64f9e20", function(err, response, body){
if(err){
console.error(err);
}else{
console.log("got splits");
console.log(body);
}
})
})
historicals(symbol, intv, span, callback)
var Robinhood = require('robinhood')(credentials, function(err, data){
Robinhood.historicals("AAPL", '5minute', 'week', function(err, response, body){
if(err){
console.error(err);
}else{
console.log("got historicals");
console.log(body);
}
})
})
url(url, callback)
url
is used to get continued or paginated data from the API. Queries with long results return a reference to the next sete. Example -
next: 'https://api.robinhood.com/orders/?cursor=cD0yMD82LTA0LTAzKzkwJVNCNTclM0ExNC45MzYyKDYlMkIwoCUzqtAW' }
The url returned can be passed to the url
method to continue getting the next set of results.
tag(tag, callback)
Retrieve Robinhood's new Tags: In 2018, Robinhood Web will expose more Social and Informational tools.
You'll see how popular a security is with other Robinhood users, MorningStar ratings, etc.
Known tags:
- 10 Most Popular Instruments:
10-most-popular
- 100 Most Popular Instruments:
100-most-popular
Response sample:
{
"slug":"10-most-popular",
"name":"10 Most Popular",
"description":"",
"instruments":[
"https://api.robinhood.com/instruments/6df56bd0-0bf2-44ab-8875-f94fd8526942/",
"https://api.robinhood.com/instruments/50810c35-d215-4866-9758-0ada4ac79ffa/",
"https://api.robinhood.com/instruments/450dfc6d-5510-4d40-abfb-f633b7d9be3e/",
"https://api.robinhood.com/instruments/e39ed23a-7bd1-4587-b060-71988d9ef483/",
"https://api.robinhood.com/instruments/1e513292-5926-4dc4-8c3d-4af6b5836704/",
"https://api.robinhood.com/instruments/39ff611b-84e7-425b-bfb8-6fe2a983fcf3/",
"https://api.robinhood.com/instruments/ebab2398-028d-4939-9f1d-13bf38f81c50/",
"https://api.robinhood.com/instruments/940fc3f5-1db5-4fed-b452-f3a2e4562b5f/",
"https://api.robinhood.com/instruments/c74a93bc-58f3-4ccb-b4e3-30c65e2f88c8/",
"https://api.robinhood.com/instruments/fdf46795-2a81-4506-880f-514c8010c163/"
]
}
popularity(symbol, callback)
Get the popularity for a specified stock.
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.popularity('GOOG', function(error, response, body) {
if (error) {
console.error(error);
} else {
console.log(body);
}
});
});
options_positions
Obtain list of options positions
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.options_positions((err, response, body) => {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
});
options_orders
Obtain list of history of option orders
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.options_orders((err, response, body) => {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
});
options_dates
Obtain list of options expirations for a ticker
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.options_positions("MSFT", (err, response, {tradable_chain_id, expiration_dates}) => {
if (err) {
console.error(err);
} else {
Robinhood.options_available(tradable_chain_id, expiration_dates[0])
}
});
});
options_available
Obtain list of options expirations for a ticker
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.options_positions("MSFT", (err, response, {tradable_chain_id, expiration_dates}) => {
if (err) {
console.error(err);
} else {
Robinhood.options_available(tradable_chain_id, expiration_dates[0])
}
});
});
news(symbol, callback)
Return news about a symbol.
get_currency_pairs
Get crypto - currency pairs
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.get_currency_pairs((err, response, body) => {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
});
get_crypto
Get cryptocurrency quote information from symbol
var credentials = require("../credentials.js")();
var Robinhood = require('robinhood')(credentials, function() {
Robinhood.get_crypto('DOGE', (err, response, body) => {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
});
Documentation lacking sample response
Feel like contributing? :)
Contributors
Alejandro U. Alvarez (@aurbano)
Related Projects
Even though this should be obvious: I am not affiliated in any way with Robinhood Financial LLC. I don't mean any harm or disruption in their service by providing this. Furthermore, I believe they are working on an amazing product, and hope that by publishing this NodeJS framework their users can benefit in even more ways from working with them.

License
