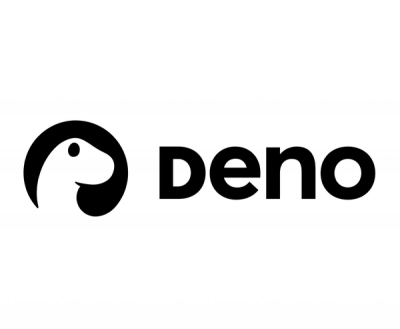
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Pluggable and middleware-oriented HTTP/S proxy with versatile routing layer, traffic interceptor and replay to multiple backends, built-in balancer and more. Built for node.js. Compatible with connect/express.
rocky
was originally designed as strategic lightweight utility for a progressive HTTP service migration, however it could be a good choice for more purposes. It can be used programmatically or via command-line interface.
For getting started, take a look to the how does it works, basic usage, examples and third-party middlewares
Still beta
rocky
is a good choice?Migrating systems if not a trivial thing, and it's even more complex if we're talking about production systems with requires high availability. Taking care about consistency and public interface contract should be a premise in most cases.
That's the main reason why rocky
borns: it was designed to become an useful tool to assist you during a backend migration strategy. You could use it as a frontend proxy server or integrated in your existent node.js
backend.
rocky
will take care about HTTP routing discerning the traffic and forwarding/replaying it accordingly to your desired new backend.
You can use it as well for multiple purposes, like A/B testing and more.
|==============|
| Dark World |
|==============|
||||
|==============|
| HTTP proxy |
|--------------|
| Rocky Router |
|~~~~~~~~~~~~~~|
| Middleware |
|==============|
|| |
(duplex) // \ (one-way)
// \
// \
/----------\ /----------\ /----------\
| target | | replay 1 | -> | replay 2 | (*N)
\----------/ \----------/ \----------/
npm install rocky --save
For command-line interface usage, install it as global package:
npm install -g rocky
Start rocky HTTP proxy server
Usage: rocky [options]
Options:
--help, -h Show help [boolean]
--config, -c File path to TOML config file [required]
--port, -p rocky HTTP server port
--forward, -f Default forward server URL
--replay, -r Define a replay server URL
--key, -k Path to SSL key file
--cert, -e Path to SSL certificate file
--secure, -s Enable SSL certification validation
--balance, -b Define server URLs to balance between, separated by commas
--debug, -d Enable debug mode [boolean]
-v, --version Show version number [boolean]
Examples:
rocky -c rocky.toml \
-f http://127.0.0.1:9000 \
-r http://127.0.0.1
rocky --config rocky.toml --port 8080 --debug
Supported params
string
- Default forward URLarray<string>
- Optional replay server URLsboolean
- Enable debug mode. Default false
boolen
- Enable SSL certificate validation. Default to false
number
- TCP port to listen. Default to 3000
boolean
- Enable/disable x-forward headers. Default true
string
- Passes the absolute URL as the path (useful for proxying to proxies)boolean
- Always forward the target hostname as Host
headerboolen
- Rewrites the location hostname on (301/302/307/308) redirectsarray<url>
- Define the URLs to balancehttps.Agent
- HTTPS agent instance. See node.js https
docsstring
- Path to SSL certificate filestring
- Path to SSL key filestring
- HTTP method for the route. Default to all
string
- Default forward URLarray<string>
- Optional replay server URLsThe configuration file must be in TOML format
port = 8080
forward = "http://google.com"
replay = ["http://duckduckgo.com"]
[ssl]
cert = "server.crt"
key = "server.key"
[/users/:id]
method = "all"
forward = "http://new.server"
[/oauth]
method = "all"
forward = "http://auth.server"
[/download/:file]
method = "GET"
balance = ["http://1.file.server", "http://2.file.server"]
[/*]
method = "GET"
forward = "http://old.server"
Example using Express
var rocky = require('rocky')
var express = require('express')
// Set up the express server
var app = express()
// Set up the rocky proxy
var proxy = rocky()
// Default proxy config
proxy
.forward('http://new.server')
.replay('http://old.server')
.replay('http://log.server')
.options({ forwardHost: true })
// Configure the routes to forward/replay
proxy
.get('/users/:id')
proxy
.get('/download/:file')
.balance(['http://1.file.server', 'http://2.file.server'])
// Plug in the rocky middleware
app.use(proxy.rr())
// Old route (won't be called since it will be intercepted by rocky)
app.get('/users/:id', function () { /* ... */ })
app.listen(3000)
Example using the built-in HTTP server
var rocky = require('rocky')
var proxy = rocky()
// Default proxy config
proxy
.forward('http://new.server')
.replay('http://old.server')
.options({ forwardHost: true })
// Configure the routes to forward/replay
proxy
.get('/users/:id')
// Overwrite the path
.toPath('/profile/:id')
// Add custom headers
.headers({
'Authorization': 'Bearer 0123456789'
})
proxy
.get('/search')
// Overwrite the forward URL for this route
.forward('http://another.server')
// Use a custom middleware for validation purposes
.use(function (req, res, next) {
if (req.headers['Autorization'] !== 'Bearer 012345678') {
res.statusCode = 401
return res.end()
}
next()
})
// Intercept and transform the response body before sending it to the client
.transformResponseBody(function (req, res, next) {
// Get the body buffer and parse it (assuming it's a JSON)
var body = JSON.parse(res.body.toString())
// Compose the new body
var newBody = JSON.stringify({ salutation: 'hello ' + body.hello })
// Send the new body in the request
next(null, newBody)
})
proxy.listen(3000)
For more usage case, take a look to the examples
Creates a new rocky instance with the given options.
You can pass any of the allowed params at configuration level and any supported http-proxy options
Alias: target
Define a default target URL to forward the request
Add a server URL to replay the incoming request
Define/overwrite rocky server options.
You can pass any of the supported options by http-proxy
.
Use the given middleware function for all http methods on the given path, defaulting to the root path.
Define a set of URLs to balance between with a simple round-robin like scheduler.
Subscribe to a proxy event. See support events here
Remove an event by its handler function. See support events here
Remove an event by its handler function. See support events here
Remove all the subscribers to the given event. See support events here
Return: Function(req, res, next)
Return a connect/express compatible middleware
Raw HTTP request/response handler.
Starts a HTTP proxy server in the given port
Close the HTTP proxy server, if exists.
A shortcut to rocky.server.close(cb)
Return: Route
Add a route handler for the given path for all HTTP methods
Return: Route
Configure a new route the given path with GET
method
Return: Route
Configure a new route the given path with POST
method
Return: Route
Configure a new route the given path with DELETE
method
Return: Route
Configure a new route the given path with PUT
method
Return: Route
Configure a new route the given path with PATCH
method
Return: Route
Configure a new route the given path with HEAD
method
http-proxy instance
HTTP router instance
HTTP/HTTPS server instance.
Only present if listen()
was called starting the built-in server.
Alias: target
Overwrite forward server for the current route.
Overwrite replay servers for the current route.
Define a set of URLs to balance between with a simple round-robin like scheduler.
Overwrite the request path, defining additional optional params.
Define or overwrite request headers
Overwrite the target hostname (defined as host
header)
Caution: using this middleware could generate negative performance side-effects since the whole payload data will be buffered in the stack until it's finished. Don't use it if you need to handle large payloads
Experimental request body interceptor and transformer middleware for the given route. This allows you to change, replace or map the response body sent from the target server before sending it to the client.
The middleware must a function accepting the following arguments: function(req, res, next)
You can see an usage example here.
You must call the next
function, which accepts the following arguments: err, newBody, encoding
The body will be exposed as raw Buffer
or String
on both properties body
and rawBody
in http.ClientRequest
:
rocky
.post('/users')
.transformRequestBody(function (req, res, next) {
// Get the body buffer and parse it (assuming it's a JSON)
var body = JSON.parse(req.body.toString())
// Compose the new body
var newBody = JSON.stringify({ salutation: 'hello ' + body.hello })
// Set the new body
next(null, newBody, 'utf8')
})
Caution: using this middleware could generate negative performance side-effects since the whole payload data will be buffered in the stack until it's finished. Don't use it if you need to handle large payloads
Experimental response body interceptor and transformer middleware for the given route. This allows you to change, replace or map the response body sent from the target server before sending it to the client.
The middleware must a function accepting the following arguments: function(req, res, next)
You can see an usage example here.
The next
function accepts the following arguments: err, newBody, encoding
The body will be exposed as raw Buffer
or String
on both properties body
and rawBody
in http.ClientResponse
:
rocky
.post('/users')
.transformResponseBody(function (req, res, next) {
// Get the body buffer and parse it (assuming it's a JSON)
var body = JSON.parse(res.body.toString())
// Compose the new body
var newBody = JSON.stringify({ salutation: 'hello ' + body.hello })
// Set the new body
next(null, newBody, 'utf8')
})
Overwrite default proxy options for the current route. You can pass any supported option by http-proxy
Add custom middlewares to the specific route.
Subscribes to a specific event for the given route. Useful to incercept the status or modify the options on-the-fly
opts, proxyReq, req, res
- Fired when the request forward startsopts, proxyRes, req, res
- Fired when the target server responderr, req, res
- Fired when the forward request failsopts, proxyReq, req, res
- Fired when a replay request startsopts, proxyRes, req, res
- Fired when a replay server respondopts, err, req, res
- Fired when the replay request failsFor more information about events, see the events fired by http-proxy
Subscribes to a specific event for the given route, and unsubscribe after dispatched
Remove an event by its handler function in the current route
Create a standalone rocky
server with the given config
options.
See the supported config fields
var config = {
'forward': 'http://google.com',
'/search': {
method: 'GET',
forward: 'http://duckduckgo.com'
replay: ['http://bing.com', 'http://yahoo.com']
},
'/users/:id': {
method: 'all'
},
'/*': {
method: 'all',
forward: 'http://bing.com'
}
}
rocky.create(config)
Expose multiple middleware functions to plugin in different level of your proxy.
Accessor for the http-proxy API
Current rocky package semver
MIT - Tomas Aparicio
FAQs
Full-featured, middleware-oriented, hackable HTTP and WebSocket proxy
The npm package rocky receives a total of 608 weekly downloads. As such, rocky popularity was classified as not popular.
We found that rocky demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.