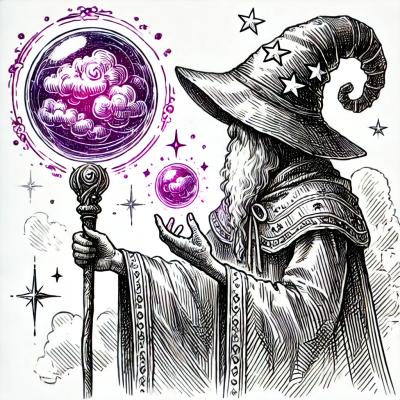
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
A lean and mean web router for node.js.
It is available through npm:
npm install router
The router routes using the method and a .net inspired pattern
var router = require('router').create();
router.get('/', function(request, response) {
response.writeHead(200);
response.end('hello index page');
});
router.listen(8080); // start the server on port 8080
If you want to grap a part of the path you can use capture groups in the pattern:
router.get('/{base}', function(request, response) {
var base = request.params.base; // ex: if the path is /foo/bar, then base = foo
});
The capture patterns matches until the next /
or character present after the group
router.get('/{x}x{y}', function(request, response) {
// if the path was /200x200, then request.params = {x:'200', y:'200'}
});
Optional patterns are supported by adding a ?
at the end
router.get('/{prefix}?/{top}', function(request, response) {
// matches both '/a/b' and '/b'
});
If you want to just match everything you can use a wildcard *
which works like unix wildcards
router.get('/{prefix}/*', function(request, response) {
// matches both '/a/', '/a/b', 'a/b/c' and so on.
// the value of the wildcard is available through request.params.wildcard
});
If the standard capture groups aren't expressive enough for you can specify an optional inline regex
router.get('/{digits}([0-9]+)', function(request, response) {
// matches both '/24' and '/424' but not '/abefest' and so on.
});
You can also use regular expressions and the related capture groups instead:
router.get(/^\/foo\/(\w+)/, function(request, response) {
var group = request.params[1]; // if path is /foo/bar, then group is bar
});
Besides get
the avaiable methods are options
, post
, put
, head
, del
, all
and upgrade
.
all
matches all the standard http methods and upgrade
is usually used for websockets.
FAQs
Simple middleware-style router
The npm package router receives a total of 616,758 weekly downloads. As such, router popularity was classified as popular.
We found that router demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.