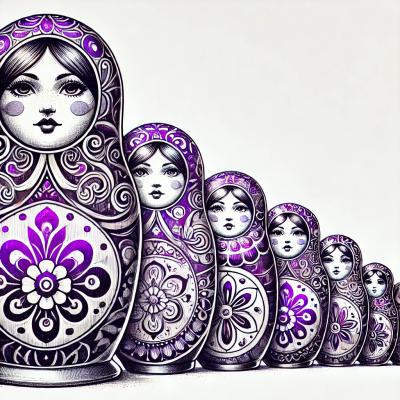
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Framework for transforming cascading style sheets (CSS) from left-to-right (LTR) to right-to-left (RTL)
RTLCSS is a framework for transforming CSS from left-to-right (LTR) to right-to-left (RTL). It is particularly useful for creating websites that need to support both LTR and RTL languages, such as English and Arabic.
Basic LTR to RTL conversion
This feature allows you to convert basic CSS properties from LTR to RTL. For example, 'margin-left' is converted to 'margin-right'.
const rtlcss = require('rtlcss');
const ltrCSS = 'body { margin-left: 10px; }';
const rtlCSS = rtlcss.process(ltrCSS);
console.log(rtlCSS); // Outputs: body { margin-right: 10px; }
Custom Directives
RTLCSS supports custom directives that allow you to control the transformation process. In this example, the '/*rtl:ignore*/' directive prevents the 'float: left;' rule from being converted.
const rtlcss = require('rtlcss');
const ltrCSS = '/*rtl:ignore*/ .example { float: left; }';
const rtlCSS = rtlcss.process(ltrCSS);
console.log(rtlCSS); // Outputs: .example { float: left; }
Plugins
RTLCSS supports plugins that can extend its functionality. This example shows how to use a plugin with RTLCSS.
const rtlcss = require('rtlcss');
const rtlcssPlugin = require('rtlcss-plugin');
const ltrCSS = 'body { margin-left: 10px; }';
const rtlCSS = rtlcss.process(ltrCSS, {}, [rtlcssPlugin]);
console.log(rtlCSS); // Outputs: body { margin-right: 10px; }
PostCSS-RTL is a plugin for PostCSS that transforms LTR CSS to RTL. It offers similar functionality to RTLCSS but is designed to work within the PostCSS ecosystem, making it a good choice if you are already using PostCSS.
RTLCSS Webpack Plugin is a Webpack plugin that uses RTLCSS to generate RTL stylesheets from LTR stylesheets. It is specifically designed for use with Webpack, making it a good option if you are using Webpack for your build process.
FlipCSS is a tool for converting LTR CSS to RTL. It offers similar functionality to RTLCSS but is a standalone tool rather than a library, making it a good choice for command-line usage.
RTLCSS is a framework for converting LTR CSS to RTL.
CSS Syntax
A CSS rule has two main parts: a selector, and one or more declarations:
The selector is normally the HTML element you want to style. Each declaration consists of a property and a value. The property is the style attribute you want to change. Each property has a value.
npm install rtlcss
var rtlcss = require('rtlcss');
var result = rtlcss.process("body { direction:ltr; }");
//result == body { direction:rtl; }
RTLCSS preserves original input formatting and indentations.
Convert LTR CSS files to RTL using the command line.
$ rtlcss input.css output.rtl.css
For usage and available options see CLI documentaion.
background
background-image
background-position
background-position-x
border-bottom-left-radius
border-bottom-right-radius
border-color
border-left
border-left-color
border-left-style
border-left-width
border-radius
border-right
border-right-color
border-right-style
border-right-width
border-style
border-top-left-radius
border-top-right-radius
border-width
box-shadow
clear
cursor
direction
float
left
margin
margin-left
margin-right
padding
padding-left
padding-right
right
text-align
text-shadow
transform
transform-origin
transition
transition-property
When RTLing a CSS document, there are cases where its impossible to know if the property value should be mirrored or not! If the rule selector need to be changed! Or a none directional property have to be updated. In such cases, RTLCSS provides processing directives in the form of CSS comments, both standard /*rtl:...*/
and special/important /*!rtl:...*/
notations are supported.
Two sets of processing directives are available, on Rule and Declaration level.
Rule level directives are placed before the CSS rule.
Directive | Description |
---|---|
/*rtl:ignore*/ | Ignores processing of this rule. |
/*rtl:rename*/ | Forces selector renaming by swapping left, right, ltr, rtl, west and east. |
Example
/*rtl:ignore*/
.code{
direction:ltr;
text-align:left;
}
Output
.code{
direction:ltr;
text-align:left;
}
Declaration level directives are placed any where inside the declaration value.
Directive | Description |
---|---|
/*rtl:ignore*/ | Ignores processing of this declaration. |
/*rtl:{value}*/ | Replaces the declaration value with {value} . |
/*rtl:append:{value}*/ | Appends {value} to the end of the declaration value. |
/*rtl:prepend:{value}*/ | Prepends {value} to the begining of the declaration value. |
/*rtl:insert:{value}*/ | Inserts {value} to where the directive is located inside the declaration value. |
Example
body{
font-family:"Droid Sans", "Helvetica Neue", Arial/*rtl:prepend:"Droid Arabic Kufi", */;
font-size:16px/*rtl:14px*/;
}
Output
body{
font-family:"Droid Arabic Kufi", "Droid Sans", "Helvetica Neue", Arial;
font-size:14px;
}
// directly processes css and return a string containing the processed css
output = rtlcss.process(css [, options , rules, declarations, properties]);
output // processed CSS
// create a new RTLCSS instance, then process css with postcss options (such as source map)
result = rtlcss([options , rules, declarations, properties]).process(css, postcssOptions);
result.css // Processed CSS
result.map // Source map
// you can also group all configuration settings into a single object
result = rtlcss.configure(config).process(css, postcssOptions);
result.css // Processed CSS
result.map // Source map
Built on top of PostCSS, an awesome framework, providing you with the power of manipulating CSS via a JS API.
It can be combined with other processors, such as autoprefixer:
var processed = postcss()
.use(rtlcss([options , rules, declarations, properties]).postcss)
.use(autoprefixer().postcss)
.process(css);
Option | Default | Description |
---|---|---|
preserveComments | true | Preserves modified declarations comments as much as possible. |
preserveDirectives | false | Preserves processing directives. |
swapLeftRightInUrl | true | Swaps left and right in URLs. |
swapLtrRtlInUrl | true | Swaps ltr and rtl in URLs. |
swapWestEastInUrl | true | Swaps west and east in URLs. |
autoRename | true | Applies to CSS rules containing no directional properties, it will update the selector by swapping left, right, ltr, rtl, west and east.(more info) |
greedy | false | A false value forces selector renaming and url updates to respect word boundaries, for example: .ultra { ...} will not be changed to .urtla {...} |
stringMap | see String Map | Applies to string replacement in renamed selectors and updated URLs |
enableLogging | false | Outputs information about mirrored declarations to the console. |
minify | false | Minifies output CSS, when set to true both preserveDirectives and preserveComments will be set to false . |
String map is a collection of map objects, each defines mapping between directional strings, it is used in selectors renaming and URL updates. The following is the default string map:
[
{
'name' : 'left-right',
'search' : ['left', 'Left', 'LEFT'],
'replace' : ['right', 'Right', 'RIGHT'],
'options' : {
'scope': options.swapLeftRightInUrl ? '*' : 'selector',
'ignoreCase': false
}
},
{
'name' : 'ltr-rtl',
'search' : ['ltr', 'Ltr', 'LTR'],
'replace' : ['rtl', 'Rtl', 'RTL'],
'options' : {
'scope': options.swapLtrRtlInUrl ? '*' : 'selector',
'ignoreCase': false
}
},
{
'name':'west-east',
'search': ['west', 'West', 'WEST'],
'replace': ['east', 'East', 'EAST'],
'options' : {
'scope': options.swapWestEastInUrl ? '*' : 'selector',
'ignoreCase': false
}
}
]
To override any of the default maps, just add your own with the same name. A map object consists of the following:
Property | Type | Description |
---|---|---|
name | string | Name of the map object |
search | string or Array | The string or list of strings to search for or replace with. |
replace | string or Array | The string or list of strings to search for or replace with. |
options | object | Defines map options. |
The map options
is optional, and consists of the following:
Property | Type | Default | Description |
---|---|---|---|
scope | string | * | Defines the scope in which this map is allowed, 'selector' for selector renaming, 'url' for url updates and '*' for both. |
ignoreCase | Boolean | true | Indicates if the search is case-insensitive or not. |
greedy | Boolean | reverts to options.greedy | A false value forces selector renaming and url updates to respect word boundaries. |
Example
// a simple map, for swapping "prev" with "next" and vice versa.
{
"name" : "prev-next",
"search" : "prev",
"replace" : "next"
}
// a more detailed version, considering the convention used in the authored CSS document.
{
"name" : "prev-next",
"search" : ["prev", "Prev", "PREV"],
"replace" : ["next", "Next", "NEXT"],
options : {"ignoreCase":false}
}
Array of RTLCSS rule Processing Instructions (PI), these are applied on the CSS rule level:
Property | Type | Description |
---|---|---|
name | string | Name of the PI (used in logging). |
expr | RegExp | Regular expression object that will be matched against the comment preceeding the rule. |
important | boolean | Controls whether to insert the PI at the start or end of the rules PIs list. |
action | function | The action to be called when a match is found, and it will be passed a rule node. the functions is expected to return a boolean, true to stop further processing of the rule, otherwise false . |
Visit PostCSS to find out more about rule
node.
// RTLCSS rule processing instruction
{
"name" : "ignore",
"expr" : /\/\*rtl:ignore\*\//img,
"important" : true,
"action" : function (rule) {
return true;
}
}
Array of RTLCSS declaration Processing Instructions (PI), these are applied on the CSS declaration level:
Property | Type | Description |
---|---|---|
name | string | Name of the PI (used in logging). |
expr | RegExp | Regular expression object that will be matched against the declaration raw value. |
important | boolean | Controls whether to insert the PI at the start or end of the declarations PIs list. |
action | function | The action to be called when a match is found, and it will be passed a decl node. the functions is expected to return a boolean, true to stop further processing of the declaration, otherwise false . |
Visit PostCSS to find out more about decl
node.
// RTLCSS declaration processing instruction
{
"name" : "ignore",
"expr" : /(?:[^]*)(?:\/\*rtl:ignore)([^]*?)(?:\*\/)/img,
"important" : true,
"action" : function (decl) {
if (!config.preserveDirectives)
decl._value.raw = decl._value.raw.replace(/\/\*rtl:[^]*?\*\//img, "");
return true;
}
},
Array of RTLCSS properties Processing Instructions (PI), these are applied on the CSS property level:
Property | Type | Description |
---|---|---|
name | string | Name of the PI (used in logging). |
expr | RegExp | Regular expression object that will be matched against the declaration property name. |
important | boolean | Controls whether to insert the PI at the start or end of the declarations PIs list. |
action | function | The action to be called when a match is found, it will be passed a prop (string holding the CSS property name) and value (string holding the CSS property raw value). If options.preserveComments == true , comments in the raw value will be replaced by the Unicode Character 'REPLACEMENT CHARACTER' (U+FFFD) � (this is to simplify pattern matching). The function is expected to return an object containing the modified version of the property and its value. |
// RTLCSS property processing instruction
{
"name" : "direction",
"expr" : /direction/im,
"important" : true,
"action" : function (prop, value) {
return { 'prop': prop, 'value': util.swapLtrRtl(value) };
}
}
Have a bug or a feature request? please feel free to open a new issue .
v1.3.1 [29 Sep. 2014]
v1.3.0 [28 Sep. 2014]
v1.2.0 [26 Sep. 2014]
v1.1.0 [26 Sep. 2014]
border-color
, border-style
and background-position-x
v1.0.0 [24 Aug. 2014]
v0.9.0 [10 Aug. 2014]
rtlcssConfig
property.rtlcssrc
or .rtlcssrc.json
v0.8.0 [8 Aug. 2014]
v0.7.0 [4 Jul. 2014]
v0.6.0 [4 Jul. 2014]
ignore
/rename
rule level directives.v0.5.0 [11 Jun. 2014]
v0.4.0 [5 Apr. 2014]
v0.3.0 [5 Apr. 2014]
v0.2.1 [20 Mar. 2014]
v0.2.0 [20 Mar. 2014]
v0.1.3 [7 Mar. 2014]
v0.1.2 [5 Mar. 2014]
v0.1.1 [4 Mar. 2014]
1.3.1 - 29 Sep. 2014
FAQs
Framework for transforming cascading style sheets (CSS) from left-to-right (LTR) to right-to-left (RTL)
The npm package rtlcss receives a total of 575,209 weekly downloads. As such, rtlcss popularity was classified as popular.
We found that rtlcss demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.