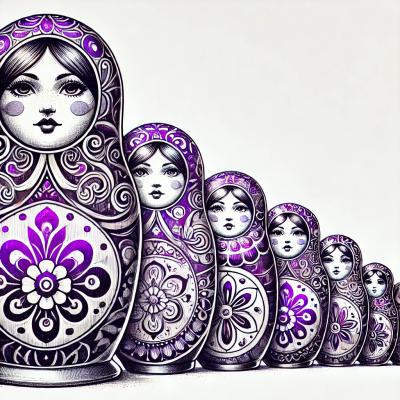
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The 'rw' npm package is a utility library for reading and writing files in Node.js. It provides simple and efficient methods for handling both synchronous and asynchronous file operations, making it easier to work with the file system in Node.js applications.
Reading files synchronously
This feature allows you to read files synchronously. The method 'readFileSync' reads the entire content of the file at the specified path and returns it. This is useful when you need to load file data immediately before proceeding with the rest of your code.
const rw = require('rw');
const data = rw.readFileSync('/path/to/file.txt', 'utf8');
console.log(data);
Writing files synchronously
This feature enables you to write data to a file synchronously. The method 'writeFileSync' writes the specified content to the file at the given path. This is useful for saving data immediately and ensuring that the file write operation completes before moving on in your code.
const rw = require('rw');
rw.writeFileSync('/path/to/file.txt', 'Hello, world!', 'utf8');
Reading files asynchronously
This feature allows you to read files asynchronously. The method 'readFile' reads the file content at the specified path and then executes a callback function with the read data. This is beneficial for non-blocking file operations in Node.js applications.
const rw = require('rw');
rw.readFile('/path/to/file.txt', 'utf8', function(err, data) {
if (err) throw err;
console.log(data);
});
Writing files asynchronously
This feature enables you to write data to a file asynchronously. The method 'writeFile' writes the specified content to the file at the given path and then executes a callback function once the write operation is complete. This helps in performing non-blocking file writes.
const rw = require('rw');
rw.writeFile('/path/to/file.txt', 'Hello, async world!', 'utf8', function(err) {
if (err) throw err;
console.log('File has been written');
});
fs-extra is a package that builds on the native 'fs' module in Node.js, providing additional file system methods not found in the standard library. It offers similar functionalities to 'rw' but includes extra features like copying directories and files, removing directories, and ensuring file paths exist.
graceful-fs is a drop-in replacement for the 'fs' module that makes file system operations more robust by queuing them and avoiding EMFILE errors. It provides similar basic file reading and writing functionalities as 'rw', but with enhancements for handling large numbers of concurrent file system operations.
How do you read a file from stdin? If you thought,
var contents = fs.readFileSync("/dev/stdin", "utf8");
you’d be wrong, because Node only reads up to the size of the file reported by fs.stat rather than reading until it receives an EOF. So, if you redirect a file to your program (cat file | program
), you’ll only read the first 65,536 bytes of your file. Oops.
What about writing a file to stdout? If you thought,
fs.writeFileSync("/dev/stdout", contents, "utf8");
you’d also be wrong, because this tries to close stdout, so you get this error:
Error: UNKNOWN, unknown error
at Object.fs.writeSync (fs.js:528:18)
at Object.fs.writeFileSync (fs.js:975:21)
Shucks. So what should you do?
You could use a different pattern for reading from stdin:
var chunks = [];
process.stdin
.on("data", function(chunk) { chunks.push(chunk); })
.on("end", function() { console.log(chunks.join("").length); })
.setEncoding("utf8");
But that’s a pain, since now your code has two different code paths for reading inputs depending on whether you’re reading a real file or stdin. And the code gets even more complex if you want to read that file synchronously.
You could also try a different pattern for writing to stdout:
process.stdout.write(contents);
Or even:
console.log(contents);
But if you try to pipe your output to head
, you’ll get this error:
Error: write EPIPE
at errnoException (net.js:904:11)
at Object.afterWrite (net.js:720:19)
Huh.
The rw module fixes these problems. It provides an interface just like readFile, readFileSync, writeFile and writeFileSync, but with implementations that work the way you expect on stdin and stdout. If you use these methods on files other than /dev/stdin or /dev/stdout, they simply delegate to the fs methods, so you can trust that they behave identically to the methods you’re used to.
For example, now you can read stdin synchronously like so:
var contents = rw.readFileSync("/dev/stdin", "utf8");
Or to write to stdout:
rw.writeFileSync("/dev/stdout", contents, "utf8");
And rw automatically squashes EPIPE errors, so you can pipe the output of your program to head
and you won’t get a spurious stack trace.
To install, npm install rw
.
# rw.readFile(path[, options], callback)
Reads the file at the specified path completely into memory, invoking the specified callback once the data is available and the file is closed. The callback is invoked with two arguments: the error that occurred during read (hopefully null), and the read data. If options is a string, it specifies the encoding to use, in which case the read data will be a string; otherwise options is an object, and may specify encoding and flag properties. This method is a drop-in replacement for fs.readFile and fixes the behavior of special files such as /dev/stdin.
# rw.readFileSync(path[, options])
Reads the file at the specified path completely into memory, synchronously, returning the data. If an error occurred during read, this function throws an error instead. If options is a string, it specifies the encoding to use, in which case the read data will be a string; otherwise options is an object, and may specify encoding and flag properties. This method is a drop-in replacement for fs.readFileSync and fixes the behavior of special files such as /dev/stdin.
# rw.writeFile(path, data[, options], callback)
Writes the specified data (completely in memory) to a file at the specified path, invoking the specified callback once the data is completely written and the file is closed. The callback is invoked with a single argument: the error that occurred during write (hopefully null). If options is a string, it specifies the encoding to use, in which case the data must be a string; otherwise options is an object, and may specify encoding, mode and flag properties. This method is a drop-in replacement for fs.writeFile and fixes the behavior of special files such as /dev/stdout.
# rw.writeFileSync(path, data[, options])
Writes the specified data (completely in memory) to a file at the specified path, synchronously, returning once the data is completely written and the file is closed. Throws an error if one occurs during write. If options is a string, it specifies the encoding to use, in which case the data must be a string; otherwise options is an object, and may specify encoding, mode and flag properties. This method is a drop-in replacement for fs.writeFileSync and fixes the behavior of special files such as /dev/stdout.
# rw.dash.readFile(path[, options], callback)
Equivalent to rw.readFile, except treats a path of -
as /dev/stdin
. Useful for command-line arguments.
# rw.dash.readFileSync(path[, options])
Equivalent to rw.readFileSync, except treats a path of -
as /dev/stdin
. Useful for command-line arguments.
# rw.dash.writeFile(path, data[, options], callback)
Equivalent to rw.writeFile, except treats a path of -
as /dev/stdout
. Useful for command-line arguments.
# rw.dash.writeFileSync(path, data[, options])
Equivalent to rw.writeFileSync, except treats a path of -
as /dev/stdout
. Useful for command-line arguments.
FAQs
Now stdin and stdout are files.
The npm package rw receives a total of 2,780,036 weekly downloads. As such, rw popularity was classified as popular.
We found that rw demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.