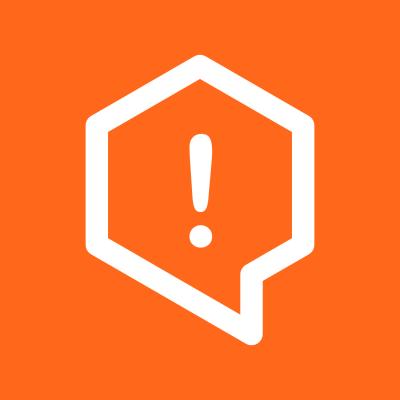
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
rx-http-request
Advanced tools
The world-famous HTTP client Request now RxJS compliant, wrote in full ES2015 for client and server side.
The world-famous HTTP client Request now RxJS compliant, wrote in full ES2015 for client and server side.
RX-HTTP-Request is designed to be the simplest way possible to make http calls.
It's fully ES2015
wrotten so you can import it :
import {RxHttpRequest} from 'rx-http-request';
or use CommonJS
:
const RxHttpRequest = require('rx-http-request').RxHttpRequest;
Now, it's easy to perform a HTTP
request:
RxHttpRequest.get('http://www.google.fr').subscribe(
(data) => {
if (data.response.statusCode === 200) {
console.log(data.body); // Show the HTML for the Google homepage.
}
},
(err) => console.error(err) // Show error in console
);
RX-HTTP-Request can be used in your favorite browser to have all features in your own front application.
Just import browser.js
script and enjoy:
<script src="node_modules/rx-http-request/browser.js" type="application/javascript"></script>
<script type="application/javascript">
const RxHttpRequest = rhr.RxHttpRequest;
RxHttpRequest.get('http://www.google.fr').subscribe(
function(data){
if (data.response.statusCode === 200) {
console.log(data.body); // Show the HTML for the Google homepage.
}
},
function(err){
console.error(err) // Show error in console
}
);
</script>
RX-HTTP-Request uses Request API to perform calls and returns RxJS.Observable.
All options to pass to API methods can be found here.
All methods to execute on response object can be found here.
.request
Returns the original Request API to perform calls without RxJS.Observable
response but with a callback method.
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.request({uri: 'http://www.google.fr'}, (error, response, body) => {
if (!error && response.statusCode == 200) {
console.log(body); // Show the HTML for the Google homepage.
}
});
.defaults(options)
This method returns a wrapper around the normal RX-HTTP-Request API that defaults to whatever options you pass to it.
Parameters:
options (required): Original Request
options
object with default values foreach next requests
Response:
new
RxHttpRequest
instance
Note: RxHttpRequest.defaults()
does not modify the global API; instead, it returns a wrapper that has your default settings applied to it.
Note: You can call .defaults()
on the wrapper that is returned from RxHttpRequest.defaults()
to add/override defaults that were previously defaulted.
For example:
// requests using baseRequest will set the 'x-token' header
const baseRequest = RxHttpRequest.defaults({
headers: {'x-token': 'my-token'}
});
// requests using specialRequest will include the 'x-token' header set in
// baseRequest and will also include the 'special' header
const specialRequest = baseRequest.defaults({
headers: {special: 'special value'}
});
.get(uri[, options])
Performs a request with get
http method.
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.get('http://www.google.fr').subscribe(
(data) => {
if (data.response.statusCode === 200) {
console.log(data.body); // Show the HTML for the Google homepage.
}
},
(err) => console.error(err) // Show error in console
);
import {RxHttpRequest} from 'rx-http-request';
const options = {
qs: {
access_token: 'xxxxx xxxxx' // -> uri + '?access_token=xxxxx%20xxxxx'
},
headers: {
'User-Agent': 'RX-HTTP-Request'
},
json: true // Automatically parses the JSON string in the response
};
RxHttpRequest.get('https://api.github.com/user/repos', options).subscribe(
(data) => {
if (data.response.statusCode === 200) {
console.log(data.body); // Show the JSON response object.
}
},
(err) => console.error(err) // Show error in console
);
.post(uri[, options])
Performs a request with post
http method.
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
import {RxHttpRequest} from 'rx-http-request';
const options = {
body: {
some: 'payload'
},
json: true // Automatically stringifies the body to JSON
};
RxHttpRequest.post('http://posttestserver.com/posts', options).subscribe(
(data) => {
if (data.response.statusCode === 201) {
console.log(data.body); // Show the JSON response object.
}
},
(err) => console.error(err) // Show error in console
);
import {RxHttpRequest} from 'rx-http-request';
const options = {
form: {
some: 'payload' // Will be urlencoded
},
headers: {
/* 'content-type': 'application/x-www-form-urlencoded' */ // Set automatically
}
};
RxHttpRequest.post('http://posttestserver.com/posts', options).subscribe(
(data) => {
if (data.response.statusCode === 201) {
console.log(data.body); // POST succeeded...
}
},
(err) => console.error(err) // Show error in console
);
.put(uri[, options])
Performs a request with put
http method.
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.put(uri).subscribe(...);
.patch(uri[, options])
Performs a request with patch
http method.
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.patch(uri).subscribe(...);
.delete(uri[, options])
Performs a request with delete
http method.
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.delete(uri).subscribe(...);
.head(uri[, options])
Parameters:
- uri (required): The
uri
where request will be performed- options (optional): Original Request
options
object
Response:
RxJS.Observable instance
Performs a request with head
http method.
import {RxHttpRequest} from 'rx-http-request';
RxHttpRequest.head(uri).subscribe(...);
To set up your development environment:
cd
to the main folder,npm install
,npm install gulp -g
if you haven't installed gulp globally yet, andgulp
. (Or run node ./node_modules/.bin/gulp if you don't want to install gulp globally.)gulp
watches all source files and if you save some changes it will lint the code and execute all tests. The test coverage report can be viewed from ./coverage/lcov-report/index.html
.
Copyright (c) 2016 Nicolas Jessel Licensed under the MIT license.
FAQs
The world-famous HTTP client Request now RxJS compliant, wrote in full ES2015 for client and server side.
We found that rx-http-request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.