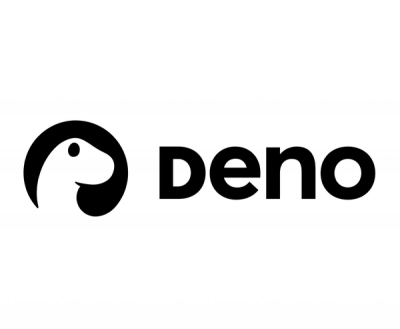
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Offline-first database with Reactive, Sync, Schema, Promises, Mongo-Query, Encryption, LevelDown
The offline-first database for your next applications.
RxDB is a Javascript-based database with..
..these features.. | ..for these plattforms | |
|
![]() |
|
Installation:
npm install rxdb --save
ES6:
import * as RxDB from 'rxdb';
RxDB.create('heroesDB', 'websql', 'myLongAndStupidPassword', true)
.then(db => db.collection('mycollection', mySchema))
.then(collection => collection.insert({name: 'Bob'}))
ES5:
var RxDB = require('rxdb');
RxDB.create('heroesDB', 'websql', 'myLongAndStupidPassword', true)
.then(function(db) {return db.collection('mycollection', mySchema);})
.then(function(collection) {collection.insert({name: 'Bob'});})
To find data in your collection, you can use chained mango-queries, which you maybe know from mongoDB or mongoose. Example:
myCollection
.find()
.where('name').ne('Alice')
.where('age').gt(18).lt(67)
.limit(10)
.sort('-age')
.exec().then( docs => {
console.dir(docs);
});
RxDB implements rxjs to make your data reactive. This makes it easy to always show the real-time database-state in the dom without manually re-submitting your queries.
heroCollection
.find()
.sort('name')
.$() // <- returns observable of query
.subscribe( docs => {
myDomElement.innerHTML = docs
.map(doc => '<li>' + doc.get('name') + '</li>')
.join();
});
When two instances of RxDB use the same storage-engine, their state and action-stream will be broadcasted. This means with two browser-windows the change of window #1 will automatically affect window #2. This works completely serverless.
Because RxDB relies on glorious PouchDB, it is easy to replicate the data between devices and servers. And yes, the changeEvents are also synced.
Schemas are defined via jsonschema and are used to describe your data. Beside the jsonschema-keywords, you can also use primary and encrypted. Example:
var mySchema = {
title: "hero schema",
description: "describes a simple hero",
type: "object",
properties: {
name: {
type: "string",
primary: true // <- this means: unique, required, string and will be used as '_id'
},
secret: {
type: "string",
encrypted: true // <- this means that the value of this field is stored encrypted
},
skills: {
type: "array",
maxItems: 5,
uniqueItems: true,
item: {
type: "object",
properties: {
name: {
type: "string"
},
damage: {
type: "number"
}
}
}
}
},
required: ["color"]
};
By setting a schema-field to encrypted: true
, the value of this field will be stored in encryption-mode and can't be read without the password. Of course you can also encrypt nested objects. Example:
"secret": {
"type": "string",
"encrypted": true
}
The underlaying pouchdb can use different adapters as storage engine. You can so use RxDB in different environments by just switching the adapter. For example you can use websql in the browser, localstorage in mobile-browsers and a leveldown-adapter in nodejs.
// this requires the localstorage-adapter
RxDB.plugin(require('pouchdb-adapter-localstorage'));
// this creates a database with the localstorage-adapter
RxDB.create('heroesDB', 'localstorage');
RxDB lets you import and export the whole database or single collections into json-objects. This is helpful to trace bugs in your application or to move to a given state in your tests.
```js// export a single collection myCollection.dump() .then(json => { console.dir(json); });
// export the whole database myDatabase.dump() .then(json => { console.dir(json); });
// import the dump to the collection emptyCollection.importDump(json) .then(() => { console.log('done'); });
// import the dump to the database emptyDatabase.importDump(json) .then(() => { console.log('done'); });
<h2>Getting started</h2>
Get started now by [reading the docs](./docs/README.md) or exploring the [example-projects](./examples).
FAQs
A local-first realtime NoSQL Database for JavaScript applications - https://rxdb.info/
The npm package rxdb receives a total of 14,534 weekly downloads. As such, rxdb popularity was classified as popular.
We found that rxdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.