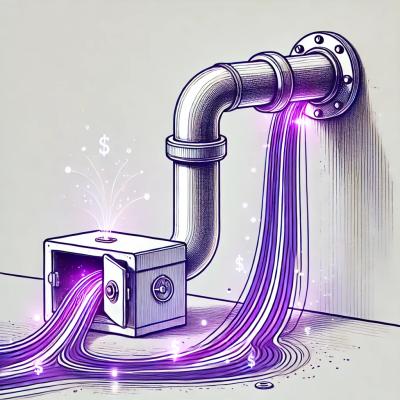
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
sb-js-data-structures
Advanced tools
JavaScript Data Structure written in TypeScript
Using npm:
npm install sb-ds-data-structures
Then where needed:
import { LinkedList } from 'sb-js-data-structures'
const list = new LinkedList()
list.addToLast(1)
list.addToLast(2)
Creates a Linked List, a data structure where each element is a separate object and the elements are linked using pointers.
import { LinkedList } from 'sb-js-data-structures'
const list = new LinkedList()
public addToLast(element: T): void
T
- The element to be insertedInserts an element in the ending of the Linked List.
public addToHead(element: T): void
T
- The element to be insertedInserts an element in the beginning of the Linked List.
public addElementAtPosition(element: T, position: number): void
T
- The element to be insertednumber
- position where the element should be insertedInserts an element in a specific position of the Linked List, since position
is less then the number of elements of the Linked List.
public removeFromLast(): void
Removes the element from the ending of the Linked List.
public removeFromHead(): void
Removes the element from the beginning of the Linked List.
public removeFirstElementFoundFromList(element: T): void
T
- The element to be removedRemoves the first element found in the Linked List.
public removeAllElementsFromList(element: T): void
T
- The element to be removedRemoves all the elements found in the Linked List.
public reverse(): void
Reverse the Linked List.
public fromArray(element: T[]): void
T[]
- Array of elementsInserts all the elements
at the ending of the Linked List.
public toArray(): T[]
Creates an array with all the elements of the Linked List.
public getLength(): number
Gets the length of the Linked List.
public isEmpty(): boolean
true
if the Linked List has no elements, otherwise, returns false
.Informs if the Linked List is empty.
public isEmpty(): boolean
Display the Linked List elements.
Creates a Sorted List, a data structure where each element is a separate object, the elements linked using pointers and the elements are sorted.
import { SortedList } from 'sb-js-data-structures'
const list = new SortedList()
public add(element: T): void
T
- The element to be insertedInserts an element in the Sorted List.
public removeFromLast(): void
Removes the element from the ending of the Sorted List.
public removeFromHead(): void
Removes the element from the beginning of the Sorted List.
public removeFirstElementFoundFromList(element: T): void
T
- The element to be removedRemoves the first element found in the Sorted List.
public removeAllElementsFromList(element: T): void
T
- The element to be removedRemoves all the elements found in the Sorted List.
public fromArray(element: T[]): void
T[]
- Array of elementsInserts all the elements
at the ending of the Sorted List.
public toArray(): T[]
Creates an array with all the elements of the Sorted List.
public getLength(): number
Gets the length of the Sorted List.
public isEmpty(): boolean
true
if the Sorted List has no elements, otherwise, returns false
.Informs if the Sorted List is empty.
public isEmpty(): boolean
Creates a Doubly Linked List, a data structure where each element is a separate object and the elements linked using pointers to the next and the previous node.
import { DoublyLinkedList } from 'sb-js-data-structures'
const list = new DoublyLinkedList()
public insertToHead(element: T): void
T
- The element to be insertedInserts an element in the beginning of the Doubly Linked List.
public insertToTail(element: T): void
T
- The element to be insertedInserts an element in the ending of the Doubly Linked List.
public insertAtPosition(element: T, position: number): void
T
- The element to be insertednumber
- position where the element should be insertedInserts an element in a specific position of the Doubly Linked List, since position
is less than the number of elements of the Doubly Linked List.
public deleteFromHead(): void
Deletes an element in the beginning of the Doubly Linked List.
public deleteFromTail(): void
Deletes an element in the ending of the Doubly Linked List.
public deleteFirstFound(element: T): void
T
- The element to be deletedDeletes the element
found in the Doubly Linked List.
public deleteAllFound(element: T): void
T
- The element to be deletedDeletes all the element
s found in the Doubly Linked List.
public displayForward(): void
Displays all elements in the Doubly Linked List from the first to the last element.
public displayBackward(): void
Displays all elements in the Doubly Linked List from the last to the first element.
public search(element: T): number
T
- The element to be searchedSearches the element
in the Doubly Linked List.
public fromArray(element: T[]): void
T[]
- Array of elementsInserts all the elements
at the ending of the Doubly Linked List.
public getLength(): number
Gets the length of the Doubly Linked List.
public isEmpty(): boolean
true
if the Doubly Linked List has no elements, otherwise, returns false
.Informs if the Doubly Linked List is empty.
Creates a Queue, a first-in-first-out (FIFO) data structure. In a FIFO data structure, the first element added to the queue will be the first one to be removed.
import { Queue } from 'sb-js-data-structures'
const queue = new Queue()
public enqueue(element: T): void
T
- The element to be insertedInserts an element in the Queue.
public dequeue(): void
Removes an element from the Queue.
public peek(): void
Gets the element at the front of the Queue without removing it.
public getLength(): number
Gets the length of the Queue.
public isEmpty(): boolean
true
if the Queue has no elements, otherwise, returns false
.Informs if the Queue is empty.
Creates a Stack, a last-in-first-out (LIFO) data structure. In a LIFO data structure, the last element added to the stack will be the first one to be removed.
import { Stack } from 'sb-js-data-structures'
const stack = new Stack()
public push(element: T): void
T
- The element to be insertedInserts an element in the Stack.
public pop(): void
Removes an element from the Stack.
public peek(): void
Gets the last element at the Stack without removing it.
public getLength(): number
Gets the length of the Stack.
public isEmpty(): boolean
true
if the Stack has no elements, otherwise, returns false
.Informs if the Stack is empty.
Creates a Hash Table, a data structure that stores in an array format, where each data value has its own unique index value.
import { HashTable } from 'sb-js-data-structures'
const hash = new HashTable()
public insert(key: string, value: T): void
string
- The keyT
- The value to be insertedInserts an element in the Hash Table.
public delete(key: string): void
string
- The keyRemoves an element from the Hash Table.
public search(key: string): void
string
- The keySearches an element in a hash table.
Creates a Binary Search Tree, a last-in-first-out (LIFO) data structure. In a LIFO data structure, the last element added to the tree will be the first one to be removed.
Creates a Binary Search Tree, a data structure that is a tree in which all the nodes follow: (1) The value of the key of the left sub-tree is less than the value of its parent (root) node's key; (2) The value of the key of the right sub-tree is greater than or equal to the value of its parent (root) node's key.
import { BinarySearchTree } from 'sb-js-data-structures'
const tree = new BinarySearchTree()
export interface INode<T> {
element: T
right: INode<T> | null
left: INode<T> | null
}
public insert(element: T): void
T
- The element to be insertedInserts an element in the Binary Search Tree.
public delete(element: T): void
T
- The element to be removedRemoves an element from the Binary Search Tree.
public search(element: T): INode
T
- The element to be searchedGets the last element at the Binary Search Tree without removing it.
public getLength(): number
Gets the number of elements of the Binary Search Tree.
public isEmpty(): boolean
true
if the Binary Search Tree has no elements, otherwise, returns false
.Informs if the Binary Search Tree is empty.
public fromArray(element: T[]): void
T[]
- Array of elementsInserts all the elements
in the Binary Search Tree.
MIT
FAQs
JavaScript Data Structure written in TypeScript
We found that sb-js-data-structures demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.