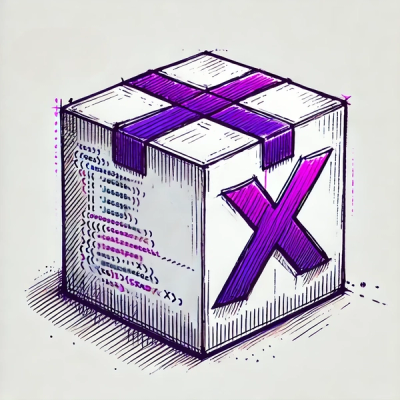
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
scrollscene
Advanced tools
ScrollScene is an extra layer on top of ScrollMagic as well as using IntersectionObserver to achieve similar effects.
ScrollScene is an extra layer on top of ScrollMagic as well as using IntersectionObserver to achieve similar effects.
View the online Storybook.
yarn add scrollscene scrollmagic
or
npm install scrollscene scrollmagic
import { ScrollScene } from 'scrollscene'
import { ScrollObserver } from 'scrollscene'
import { ScrollMagic } from 'scrollscene'
or
import { ScrollMagicSsr } from 'scrollscene'
ScrollMagic
and ScrollMagicSsr
are the exact same thing.
ScrollScene has breakpoints, duration, gsap, scrollMagic, toggle, triggerElement
.
ScrollObserver has breakpoints, gsap, observer, thresholds, toggle, triggerElement, video
.
See below for examples.
gsap
or scrollmagic
. If you plan to use them, you'll need to install them.animation.gsap.js
file from ScrollMagic (though you'll have to install in yourself yarn add gsap
or npm install gsap
).ScrollObserver
and not ScrollScene
if you wanted and your build should exclude ScrollScene
and scrollmagic
(as long as you did import them).jQuery
.setPin
in the future. Though you can import { ScrollMagic } from 'scrollscene'
do a setPin
this way. Just remember you also have to create a controller using this method and attach the scene to it.const scrollScene = new ScrollScene({
triggerElement: domNode,
})
const scrollScene = new ScrollScene({
triggerElement: domNode,
toggle: {
element: anotherDomNode,
className: 'turn-blue',
},
})
const scrollScene = new ScrollScene({
triggerElement: domNode,
toggle: {
element: anotherDomNode,
className: 'turn-blue',
reverse: true,
},
scrollMagic: {
triggerHook: 1,
},
duration: '100%',
})
const scrollScene = new ScrollScene({
triggerElement: domNode,
toggle: {
element: anotherDomNode,
className: 'turn-blue',
},
scrollMagic: {
triggerHook: 1,
},
})
// create a timeline and add a tween
const myTimeline = gsap.timeline({ paused: true })
myTimeline.to(domNode, {
x: -200,
duration: 1,
ease: 'power2.out',
})
const scrollScene = new ScrollScene({
triggerElement: domNode,
gsap: {
timeline: myTimeline,
},
})
// create a timeline and add a tween
const myTimeline = gsap.timeline({ paused: true })
myTimeline.to(domNode, {
x: -200,
duration: 1,
ease: 'power2.out',
})
const scrollScene = new ScrollScene({
triggerElement,
gsap: {
timeline: tl,
reverseSpeed: 4,
},
})
// create a timeline and add a tween
const myTimeline = gsap.timeline({ paused: true })
myTimeline.to(domNode, {
x: -200,
duration: 1,
ease: 'power2.out',
})
const scrollScene = new ScrollScene({
triggerElement,
gsap: {
timeline: tl,
},
duration: 500,
})
const scrollObserver = new ScrollObserver({
triggerElement: domNode,
toggle: {
element: anotherDomNode,
className: 'turn-blue',
},
})
// create a timeline and add a tween
const tl = gsap.timeline({ paused: true })
tl.to(squareElement, {
x: -200,
duration: 1,
ease: 'power2.out',
})
const scrollObserver = new ScrollObserver({
triggerElement: domNode,
gsap: {
timeline: tl,
},
})
// create a timeline and add a tween
const tl = gsap.timeline({ paused: true })
tl.to(squareElement, {
x: -200,
duration: 1,
ease: 'power2.out',
})
const scrollObserver = new ScrollObserver({
triggerElement: domNode,
gsap: {
timeline: tl,
yoyo: true,
delay: 0,
},
})
const scrollObserver = new ScrollObserver({
triggerElement: domNode,
video: {
element: videoTagDomNode,
},
})
Whatever you've named your scene, whether const scrollScene
or const scrollObserver
, you can destroy it with...
scrollScene.destroy()
scrollObserver.destroy()
With React it's best to do this inside either a useEffect
hook or using the componentDidMount
and componentWillUnmount
lifecycle. Whatver you choose, make sure to destroy the scene on the unmount.
See the Storybook source for good examples (story.js) found here.
import { ScrollScene } from 'scrollscene'
const MyComponent = () => {
// init ref
const containerRef = React.useRef(null)
const triggerRef = React.useRef(null)
React.useEffect(() => {
const { current: containerElement } = containerRef
const { current: triggerElement } = triggerRef
if (!containerElement && !triggerElement) {
return undefined
}
const scrollScene = new ScrollScene({
triggerElement: triggerElement,
toggle: {
element: containerElement,
className: 'turn-blue',
},
})
// destroy on unmount
return () => {
scrollScene.destroy()
}
})
return (
<div ref={containerRef}>
<div style={{ height: '50vh' }} />
<h3>Basic Example</h3>
<h1>Scroll Down</h1>
<div style={{ height: '150vh' }} />
<div ref={triggerRef}>When this hits the top the page will turn blue</div>
<div style={{ height: '150vh' }} />
</div>
)
}
You can now set breakpoints
so you scene is more responsive. They work mobile first. The below would set up a scene on tablet, but not mobile, and resizing will init and destroy.
const scrollScene = new ScrollScene({
breakpoints: { 0: false, 768: true },
})
duration
also can be responsive, finally! The below would set up a scene that lasts 50vh on mobile, 100% after
})
const scrollScene = new ScrollScene({
duration: { 0: '50%', 768: '100%' },
})
In order to use ScrollObserver on IE, you'll need a polyfill IntersectionObserver. You can do this with loading a polyfill script from https://polyfill.io/.
With Next.js you can load polyfills another way. See https://github.com/zeit/next.js/blob/canary/examples/with-polyfills/client/polyfills.js.
Add following to client/polyfills.js
/*
* This files runs at the very beginning (even before React and Next.js core)
* https://github.com/zeit/next.js/blob/canary/examples/with-polyfills/client/polyfills.js
*/
// https://www.npmjs.com/package/intersection-observer
import 'intersection-observer'
And then modify the next.config.js
// next.config.js
const nextConfig = {
webpack(config) {
/*
* Add polyfills
* https://github.com/zeit/next.js/blob/canary/examples/with-polyfills/next.config.js
*/
const originalEntry = config.entry
config.entry = async () => {
const entries = await originalEntry()
if (entries['main.js'] && !entries['main.js'].includes('./client/polyfills.js')) {
entries['main.js'].unshift('./client/polyfills.js')
}
return entries
}
return config
},
}
module.exports = nextConfig
For more on ScrollMagic, hit up scrollmagic.io/ and https://github.com/janpaepke/ScrollMagic
FAQs
ScrollScene is an extra layer on top of ScrollMagic as well as using IntersectionObserver to achieve similar effects.
The npm package scrollscene receives a total of 45 weekly downloads. As such, scrollscene popularity was classified as not popular.
We found that scrollscene demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.