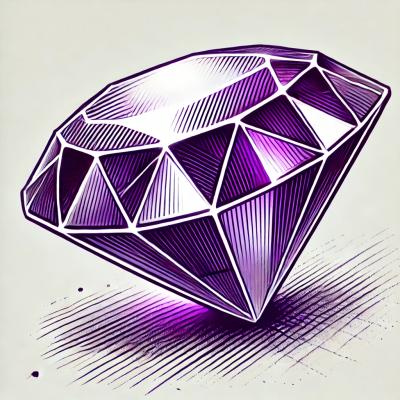
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
scuttle-testbot
Advanced tools
Spins up an empty, temporary ssb-server server that stores data in your temp folder
Spins up an empty, temporary ssb-server that stores data in your temp folder
const TestBot = require('scuttle-testbot')
const piet = TestBot()
const content = { type: 'test', text: "a test message" }
piet.db.create publish({ content }, (err, msg) => {
console.log(msg)
piet.close()
})
Outputs:
{
key: '%FQ2auS8kVY9qPgpTWNY3le/JG5+IlO6JHDjBIQcSPSc=.sha256',
value: {
previous: null,
sequence: 1,
author: '@UreG2i/rf4mz7QAVOtg0OML5SRRB42Cwwl3D1ct0mbU=.ed25519',
timestamp: 1517190039755,
hash: 'sha256',
content: { type: 'test', content: 'a test message' },
signature: '0AxMJ7cKjHQ6vJDPkVNWcGND4gUwv2Z8barND5eha7ZXH/s5T0trFqcratIqzmhE3YJU2FY61Rf1S/Za2foLCA==.sig.ed25519'
},
timestamp: 1517190039758
}
const TestBot = require('scuttle-testbot')
TestBot(opts = {})
Returns a ssb-server instance.
By default, CreateTestSbot deletes an existing database of the same name
before starting.
Valid opts
keys include:
opts.name
String (optional) (default: ssb-test + Number(new Date)
)opts.path
String (optional) (default: /tmp/${name}
, where name
is the above)
~/.ssb-test
: Sets the database in ~/.ssb-test
opts.keys
String (optional) (default: scuttle-testbot generates a new set of random keys)
ssbKeys.generate()
opts.rimraf
(default: true
)
false
: Don't delete an existing database before starting up.name
and keys
options to be connecting to the same logopts.startUnclean
is still acceptedopts.db1
(default: false
)
ssb-db2
by default, but if true
will use ssb-db
SSB_DB1=true
opts.noDefaultUse
(default: false
)
TestBot.use(plugin)
CreateTestSbot.use
lets you add ssb-server plugins. use
can be chained the same as the ssb-server api.
Example:
function Server (opts) {
const stack = Testbot
.use('ssb-master')
.use('ssb-tribes')
return stack(opts)
}
Testbot.replicate({ from, to, feedId?, live?, name?, log? }, done)
Replicates data from one testbot to another, which is sometimes needed when you have functions which are only triggered by another feedId, e.g. when I am added to a private group someone else started.
Example:
function Server (opts) {
const stack = require('scuttle-testbot')
// required for replicate
.use(require('ssb-db2/compat/feedstate'))
.use(require('ssb-db2/compat/history-stream'))
return stack(opts)
}
const piet = Server({ name: 'piet' })
const katie = Server({ name: 'katie' })
const content = {
type: 'direct-message',
text: 'oh hey'
}
piet.db.create({ content }, (err, msg) => {
TestBot.replicate({ from: piet, to: katie }, (err) => {
katie.db.getMsg(msg.key, private: true }, (err, value) => {
console.log(value.content)
// should be same as content piet sent, if katie can decrypt
piet.close()
katie.close()
})
})
})
arguments:
from
SSB - an ssb instance to be replicated from. This will replicate only this feeds messages (not everything in log)to
SSB - an ssb instance being replicate to.feedId
String (optional)
from.id
live
Boolean (optional)- whether or not to keep replication running (default: false
).
false
console.log
name
Function (optional) - makes logged output easier to read by allowing you to replace feedIds with human readable names
// example
const name = (feedId) => {
if (feedId === piet.id) return 'piet'
if (feedId === katie.id) return 'katie'
}
const piet = TestBot()
piet.name = 'piet'
const piet = TestBot({ name: 'katie ' })
log
Function|false (optional)done
Function - an optional callback which is triggered when the replication is complete or if there is an error.
live === true
this will ony be called on an error. Signature done (err) { ... }
Also supports promise style.
await TestBot.replicate({ from: piet, to: katie })
This requires that live: false
Under the hood this function just uses createHistoryStream
directly from one peer to another
Testbot.connect(peers, { names, friends }, done)
Connects all listed peers.
Example:
const crypto = require('crypto')
function Server (opts) {
const stack = require('scuttle-testbot')
.use('ssb-conn')
.use('ssb-friends') // only needed if opts.friends
return stack(opts)
}
const caps = { shs: crypto.randomBytes(32).toString('base64') }
const piet = Server({ caps, name: 'piet' })
const katie = Server({ caps, name: 'katie' })
// all peers need to have same caps to be able to connect to each other
Testbot.connect([piet, katie], { friends: true }, (err) => {
// as friends: true - piet now follows katie + vice versa
// and there is a connection live between these two
piet.close()
katie.close()
})
arguments:
peers
Array
opts
Object
opts.friends
Boolean (optional)
ssb-friends
to be installedfalse
opts.name
Function (optional) - makes logged output easier to read by allowing you to replace feedIds with human readable names
// example
const name = (feedId) => {
if (feedId === piet.id) return 'piet'
if (feedId === katie.id) return 'katie'
}
opts.log
Function|false (optional)
false
console.log
done
Function - an optional callback which is triggered when the replication is complete or if there is an error.
live === true
this will ony be called on an error. Signature done (err) { ... }
Under the hood this just uses createHistoryStream
directly from one peer to another
Also supports promise style.
await Testbot.connect([piet, katie], { friends: true })
TestBot.colorLog(obj, ...)
Like console.log(JSON.stringify(obj, null, 2))
but also:
MIT
FAQs
Spins up an empty, temporary ssb-server server that stores data in your temp folder
The npm package scuttle-testbot receives a total of 10 weekly downloads. As such, scuttle-testbot popularity was classified as not popular.
We found that scuttle-testbot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.