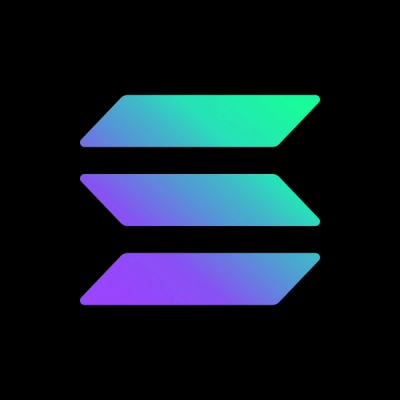
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
sendinblue-v3-node-client
Advanced tools
The Best Unofficial SendinBlue V3 API Node.js Client
sendinblue-v3-node-client exposes the entire SendinBlue API. Please refer to the full official documentation to learn more.
This is an unofficial wrapper for the API. It implements all the features of the API v3. It supports promises and callbacks.
If you are unfamiliar with npm, see the npm docs.
npm i -S sendinblue-v3-node-client
Please follow the installation instruction and execute the following JS code:
let sib = require('sendinblue-v3-node-client')("YOUR_API_KEY")
sib.account.get(function(error, account) {
if(error) console.log("Error: ", error)
else console.log("Your Account Object: ", account)
})
// OR
sib.account.get().then(function(account) {
console.log("Your Account Object: ", account)
}).catch(function(error) {
console.log("Error: ", error)
})
All URIs are relative to https://api.sendinblue.com/v3
Method | HTTP request | Description |
---|---|---|
account.get | GET /account | Get your account informations, plans and credits details |
resellers.children.all | GET /reseller | Gets the list of all reseller's children accounts |
resellers.children.create | POST /reseller | Creates a reseller child |
resellers.children.get | GET /reseller | Gets the info about a specific child account |
resellers.children.update | PUT /reseller | Updates infos of reseller's child based on the childAuthKey supplied |
resellers.children.delete | DELETE /reseller | Deletes a single reseller child based on the childAuthKey supplied |
resellers.children.ips.associate | POST /reseller | Associate a dedicated IP to the child |
resellers.children.ips.dissociate | POST /reseller | Dissociate a dedicated IP to the child |
resellers.children.credits.add | POST /reseller | Add Email and/or SMS credits to a specific child account |
resellers.children.credits.remove | POST /reseller | Remove Email and/or SMS credits from a specific child account |
senders.all | GET /senders | Return all the dedicated IPs for your account |
senders.create | POST /senders | Create a new sender |
senders.update | PUT /senders | Update a sender |
senders.delete | DELETE /senders | Delete a sender |
senders.ips.get | GET /senders | Return all the dedicated IPs for a sender |
senders.ips.all | GET /senders | Get the list of all your senders |
processes.all | GET /processes | Return all the processes for your account |
processes.get | GET /processes | Return the informations for a process |
campaigns.all | GET /emailCampaigns | Return all your created campaigns |
campaigns.get | GET /emailCampaigns | Get campaign informations |
campaigns.create | POST /emailCampaigns | Create an email campaign |
campaigns.update | PUT /emailCampaigns | Update a campaign |
campaigns.delete | DELETE /emailCampaigns | Delete an email campaign |
campaigns.send | POST /emailCampaigns | Send an email campaign id of the campaign immediately |
campaigns.test | POST /emailCampaigns | Send an email campaign to your test list |
campaigns.export | POST /emailCampaigns | Export the recipients of a campaign |
campaigns.report | POST /emailCampaigns | Send the report of a campaigns |
campaigns.status | PUT /emailCampaigns | Update a campaign status |
smtp.activity.day | GET /smtp | Get your SMTP activity aggregated per day |
smtp.activity.custom | GET /smtp | Get your SMTP activity aggregated over a period of time |
smtp.activity.all | GET /smtp | Get all your SMTP activity (unaggregated events) |
smtp.templates.all | GET /smtp | Get the list of SMTP templates |
smtp.templates.create | POST /smtp | Create an smtp template |
smtp.templates.get | GET /smtp | Returns the template informations |
smtp.templates.update | PUT /smtp | Updates an smtp templates |
smtp.templates.delete | DELETE /smtp | Delete an inactive smtp template |
smtp.templates.test | POST /smtp | Send a template to your test list |
smtp.templates.send | POST /smtp | Send a template |
smtp.transactionals.send | POST /smtp | Send a transactional email |
smtp.hardbounces.delete | POST /smtp | Delete hardbounces |
webhooks.all | GET /webhooks | Get all webhooks |
webhooks.create | POST /webhooks | Create a webhook |
webhooks.get | GET /webhooks | Get a webhook details |
webhooks.update | PUT /webhooks | Update a webhook |
webhooks.delete | DELETE /webhooks | Delete a webhook |
contacts.all | GET /contacts | Get all the contacts |
contacts.create | POST /contacts | Create a contact |
contacts.get | GET /contacts | Retrieves contact informations |
contacts.update | PUT /contacts | Updates a contact |
contacts.delete | DELETE /contacts | Deletes a contact |
contacts.statistics | GET /contacts | Get the campaigns statistics for a contact |
contacts.attributes.all | GET /contacts | Lists all attributes |
contacts.attributes.create | POST /contacts | Creates contact attribute |
contacts.attributes.update | PUT /contacts | Updates contact attribute |
contacts.attributes.delete | DELETE /contacts | Deletes an attribute |
contacts.folders.all | GET /contacts | Get all the folders |
contacts.folders.create | POST /contacts | Create a folder |
contacts.folders.get | GET /contacts | Returns folder details |
contacts.folders.update | PUT /contacts | Update a contact folder |
contacts.folders.delete | DELETE /contacts | Delete a folder (and all its lists) |
contacts.folders.lists | GET /contacts | Get the lists in a folder |
contacts.lists.all | GET /contacts | Get all the lists |
contacts.lists.create | POST /contacts | Create a list |
contacts.lists.get | GET /contacts | Get the details of a list |
contacts.lists.update | PUT /contacts | Update a list |
contacts.lists.delete | DELETE /contacts | Delete a list |
contacts.lists.contacts.get | GET /contacts | Get the contacts in a list |
contacts.lists.contacts.add | POST /contacts | Add existing contacts to a list |
contacts.lists.contacts.remove | POST /contacts | Remove existing contacts from a list |
contacts.export | POST /contacts | Export contacts |
contacts.import | POST /contacts | Import contacts |
sms.send | POST /transactionalSMS | Send the SMS campaign to the specified mobile number |
sms.all | GET /transactionalSMS | Get all the SMS activity (unaggregated events) |
sms.custom | GET /transactionalSMS | Get your SMS activity aggregated over a period of time |
sms.day | GET /transactionalSMS | Get your SMS activity aggregated per day |
Using path
, query
, and body
parameters.
// sib.path.to.endpoint([path_params],[query_params],[body_params],[callback])
// Get all contacts
sib.contacts.all({
limit: 50, // query_param
offset: 0 // query_param
}, function(error, contacts) {
if(error) console.log("Error: ", error)
else console.log("All Contacts: ", JSON.stringify(contacts, null, 2))
})
// Create new contact
sib.contacts.create({
email: "johndoe@gmail.com", // body_param
}, function(error, newContact) {
if(error) console.log("Error: ", error)
else console.log("New Contact: ", JSON.stringify(newContact, null, 2))
})
// Updates a contact
sib.contacts.update("johndoe%40gmail.com", {
emailBlacklisted: true
}, function(error, newContact) {
if(error) console.log("Error: ", error)
else console.log("Updated Contact: ", JSON.stringify(newContact, null, 2))
})
Be sure to visit the SendinBlue official documentation website for additional information about the official API.
If you find a bug, please post the issue on Github.
If you have any questions or comments, feel free to drop us a note here.
FAQs
Unofficial Node.js SendInBlue V3 API
The npm package sendinblue-v3-node-client receives a total of 2 weekly downloads. As such, sendinblue-v3-node-client popularity was classified as not popular.
We found that sendinblue-v3-node-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.