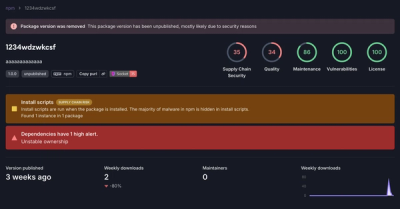
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
sequelize
Advanced tools
Sequelize is a promise-based Node.js ORM (Object-Relational Mapping) library for Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. It features solid transaction support, relations, eager and lazy loading, read replication and more. Sequelize follows the Active Record paradigm and allows developers to define models and their relationships in a way that abstracts database access, making it easier to maintain and evolve the application codebase.
Model Definition
This feature allows you to define models in Sequelize, which represent tables in the database. Each model can have various attributes and their respective data types.
const User = sequelize.define('user', { username: Sequelize.STRING, birthday: Sequelize.DATE });
CRUD Operations
Sequelize provides methods for creating, reading, updating, and deleting records in the database, which correspond to the CRUD operations.
User.create({ username: 'alice', birthday: new Date(1986, 6, 20) }); User.findAll(); User.update({ username: 'alicejr' }, { where: { id: 1 } }); User.destroy({ where: { id: 1 } });
Associations
This feature allows you to define associations between models. For example, a user can have many posts, and a post belongs to a user.
User.hasMany(Post); Post.belongsTo(User);
Transactions
Sequelize supports transactions which allow you to execute multiple queries in an atomic way, ensuring data integrity.
sequelize.transaction(transaction => { return User.create({ username: 'bob' }, { transaction }); });
Migrations
Sequelize has a migration tool that allows you to define changes to the database schema, which can be applied and rolled back programmatically.
module.exports = { up: (queryInterface, Sequelize) => { return queryInterface.createTable('users', { id: { allowNull: false, autoIncrement: true, primaryKey: true, type: Sequelize.INTEGER }, username: { type: Sequelize.STRING } }); }, down: (queryInterface, Sequelize) => { return queryInterface.dropTable('users'); } };
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It manages relationships between data, provides schema validation, and is used to translate between objects in code and the representation of those objects in MongoDB. Compared to Sequelize, Mongoose is specific to MongoDB, whereas Sequelize supports multiple SQL databases.
TypeORM is an ORM that can run in Node.js and be used with TypeScript and JavaScript (ES5, ES6, ES7, ES8). It supports the Data Mapper pattern, unlike Sequelize which is more Active Record. TypeORM is highly influenced by other ORMs, such as Hibernate, Doctrine, and Entity Framework.
Knex.js is a SQL query builder for Postgres, MSSQL, MySQL, MariaDB, SQLite3, Oracle, and Amazon Redshift, designed to be flexible, portable, and fun to use. It does not provide full ORM capabilities but allows you to build and run SQL queries in a more programmatic and database-agnostic way. It is often used with objection.js, which is an ORM built on top of Knex.
Bookshelf.js is a JavaScript ORM for Node.js, built on the Knex SQL query builder. It features transaction support, eager/nested-eager relation loading, and polymorphic associations. Bookshelf follows a somewhat similar pattern to Sequelize but is built on top of Knex, which gives it a different flavor in terms of query building.
The Sequelize library provides easy access to a MySQL database by mapping database entries to objects and vice versa. To put it in a nutshell... it's an ORM (Object-Relational-Mapper). The library is written entirely in JavaScript and can be used in the Node.JS environment.
Sequelize will have a Kiwi package in future. For now, you can install it via NPM or just download the code from the git repository and require sequelize.js:
# npm:
npm install sequelize
var Sequelize = require("sequelize").Sequelize
# checkout:
cd <path/to/lib>
git clone git://github.com/sdepold/sequelize.git
var Sequelize = require(__dirname + "/lib/sequelize/src/sequelize").Sequelize
This will make the class Sequelize available.
To get the ball rollin' you first have to create an instance of Sequelize. Use it the following way:
var sequelize = new Sequelize('database', 'username', 'password')
This will save the passed database credentials and provide all further methods.
To define mappings between a class (Stop telling me that JavaScript don't know classes. Name it however you want to!) and a table use the define method:
var Project = sequelize.define('Project', {
title: Sequelize.STRING,
description: Sequelize.TEXT
})
var Task = sequelize.define('Task', {
title: Sequelize.STRING,
description: Sequelize.TEXT,
deadline: Sequelize.DATE
})
Sequelize currently supports the following datatypes:
Sequelize.STRING ===> VARCHAR(255)
Sequelize.TEXT ===> TEXT
Sequelize.INTEGER ===> INT
Sequelize.DATE ===> DATETIME
Sequelize.BOOLEAN ===> TINYINT(1)
You can also store your model definitions in a single file using the import method:
// app.js
var Project = sequelize.import(__dirname + "/path/to/models/Project").Project
// Project.js
exports.getProjectClass = function(Sequelize, sequelize) {
return sequelize.define("Project", {
name: Sequelize.STRING,
description: Sequelize.TEXT
})
}
Choose the name of the exported function the way you want. It doesn't matter at all. You can also specify multiple models in one file. The import method will return a hash, which stores the result of sequelize.define under the key Project.
As you are able to specify an objects skeleton, the next step is to push it to a database:
// create my nice tables:
Project.sync(callback)
Task.sync(callback)
// drop the tables:
Project.drop(callback)
Task.drop(callback)
Because synchronizing and dropping all of your tables might be a lot of line to write, you can also let Sequelize do the work for you:
// create all tables... now!
sequelize.sync(callback)
// and drop it!
sequelize.drop(callback)
In order to create instances of defined classes just do it as follows:
var project = new Project({
title: 'my awesome project',
description: 'woot woot. this will make me a rich man'
})
var task = new Task({
title: 'specify the project idea',
description: 'bla',
deadline: new Date()
})
To save it in the database use the save method and pass a callback to it, if needed:
project.save(function() {
// my nice callback stuff
})
task.save(function() {
// some other stuff
})
new Task({ title: 'foo', description: 'bar', deadline: new Date()}).save(function(anotherTask) {
// you can now access the currently saved task with the variable _anotherTask_... nice!
})
Now lets change some values and save changes to the database... There are two ways to do that:
// way 1
task.title = 'a very different title now'
task.save(function(){})
// way 2
task.updateAttributes({
title: 'a very different title now'
}, function(){})
Sequelize allows you to pass custom class and instance methods. Just do the following:
var Foo = sequelize.define('Foo', { /* attributes */}, {
classMethods: {
method1: function(){ return 'smth' }
},
instanceMethods: {
method2: function() { return 'foo' }
}
})
// ==>
Foo.method1()
new Foo({}).method2()
Because you will want to save several items at once and just go on after all of them are saved, Sequelize provides a handy helper for that:
Sequelize.chainQueries([
// push your items + method calls here
], function() {
// and here do some callback stuff
})
And a real example:
Sequelize.chainQueries([
{save: project}, {save: task}
], function() {
// woot! saved.
})
You can also pass params to the method... and of course you can also call other methods with a callback:
Sequelize.chainQueries([
{ methodWithParams: project, params: [1,2,3] }
], function() {
// the method call will equal: project.methodWithParams(1,2,3, callback)
})
With Sequelize you can also specify associations between multiple classes. Doing so will help you to easily access and set those associated objects. The library therefore provides for each defined class the method belongsTo, hasOne and hasMany:
Project.hasMany("tasks", Task)
Task.belongsTo("project", Project)
Because Sequelize is doing a lot of magic, you have to call Sequelize#sync after setting the associations! Doing so will allows you the following:
var project = new Project...
var task1 = new Task...
var task2 = new Task
// save them... and then:
project.setTasks([task1, task2], function(associatedTasks) {
// the associatedTasks are the very same as task1 and task2
})
// ok now they are save... how do I get them later on?
project.tasks(function(associatedTasks) {
// bam
})
To remove created associations you can just call the set method without a specific id:
// remove task1 (only the association!)
project.setTasks([task2], function(associatedTasks) {
// you will get task2 only
})
// remove 'em all
projects.setTasks([], function(associatedTasks) {
// you will get an empty array
})
You can also do it vice versa:
// project is associated with task1 and task2
task2.setProject(null, function(associatedProject) {
// will return no associations
})
For hasOne its basically the same as with belongsTo:
Task.hasOne('author', Author)
Task.setAuthor(anAuthor)
In order to specify many-to-many associations you can use the following syntax:
Project.hasMany('members', Member)
Member.hasMany('projects', Project)
This will create a table named according to the table names of Project and Member (= ProjectsMembers) which just stores the id of a project and a member. Don't forget to call the sync method of the sequelize instance.
OK... you can define classes and associations. You can save them. You would probably like to get them from the database again :) Easy:
Project.find(123, function(project) {
// project will be an instance of Project and stores the content of the table entry with id 123
// if such an entry is not defined you will get null
})
Project.find({ title: 'aProject' }, function(project) {
// project will be the first entry of the Projects table with the title 'aProject' || null
})
Project.findAll(function(projects) {
// projects will be an array of all Project instances
})
FAQs
Sequelize is a promise-based Node.js ORM tool for Postgres, MySQL, MariaDB, SQLite, Microsoft SQL Server, Amazon Redshift and Snowflake’s Data Cloud. It features solid transaction support, relations, eager and lazy loading, read replication and more.
The npm package sequelize receives a total of 1,808,577 weekly downloads. As such, sequelize popularity was classified as popular.
We found that sequelize demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.