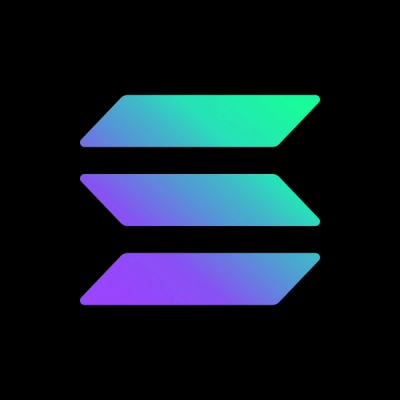
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
A terse & familiar binding to the Neo4j REST API that is idiomatic to node.js.
var db = require("seraph")("http://localhost:7474");
db.save({ name: "Test-Man", age: 40 }, function(err, node) {
if (err) throw err;
console.log("Test-Man inserted.");
db.delete(node, function(err) {
if (err) throw err;
console.log("Test-Man away!");
});
});
Seraph has been tested with Neo4j 1.8. As we progress in development, we will begin adding some legacy support for older versions.
To test Seraph, download Neo4j and extract it in the seraph directory. Rename
the neo4j folder to db
. Then:
npm test
or, if you have started the test instance yourself and don't want the tests to restart and clean the server every time:
npm run-script quick-test
rawQuery
performs a cypher query and returns the results directly from the
REST API.
query
performs a cypher query and map the columns and results together.
Note: if you're performing large queries it may be advantageous to use
queryRaw
, since query
attempts to infer whole nodes and relationships that
are returned (in order to transform them into a nicer format).
Arguments
{}
). Replace {key}
parts in query string. See
cypher documentation for details.Example
Given database:
{ name: 'Jon', age: 23, id: 1 }
{ name: 'Neil', age: 60, id: 2 }
{ name: 'Katie', age: 29, id: 3 }
// 1 --knows--> 2
// 1 --knows--> 3
Return all people Jon knows:
var cypher = "START x = node({id}) "
+ "MATCH x -[r]-> n "
+ "RETURN n "
+ "ORDER BY n.name";
db.query(cypher, {id: 1}, function(err, result) {
if (err) throw err;
assert.deepEqual(result, [
{ name: 'Katie', age: 29, id: 3 },
{ name: 'Neil', age: 60, id: 2 }
]);
};
db.rawQuery(cypher, {id: 3}, function(err, result) {
if (err) throw err;
// result contains the raw response from neo4j's rest API. See
// http://docs.neo4j.org/chunked/milestone/rest-api-cypher.html
// for more info
})
Feature planned for 1.1.0
Create an operation object that will be passed to call.
Arguments
'GET'
|'POST'
) - the HTTP method to use. When
data
is an object, method
defaults to 'POST'. Otherwise, method
defaults to GET
.Example
var operation = db.operation('node/4285/properties', 'PUT', { name: 'Jon' });
db.call(operation, function(err) {
if (!err) console.log('Set `name` to `Jon` on node 4285!')
});
Perform an HTTP request to the server.
If the body is some JSON, it is parsed and passed to the callback.If the status code is not in the 200's, an error is passed to the callback.
Arguments
result
is the JSON parsed body
from the server (otherwise empty). response
is the response object from the
request.Example
var operation = db.operation('node/4285/properties');
db.call(operation, function(err, properties) {
if (err) throw err;
// `properties` is an object containing the properties from node 4285
});
Feature planned for 1.1.0
Create or update a node. If object
has an id property, the node with that id
is updated. Otherwise, a new node is created. Returns the newly created/updated
node to the callback.
Arguments
node
is the newly saved or updated node. If
a create was performed, node
will now have an id property. The returned
object is not the same reference as the passed object (the passed object will
never be altered).Example
// Create a node
db.save({ name: 'Jon', age: 22, likes: 'Beer' }, function(err, node) {
console.log(node); // -> { name: 'Jon', age: 22, likes: 'Beer', id: 1 }
// Update it
delete node.likes;
node.age++;
db.save(node, function(err, node) {
console.log(node); // -> { name: 'Jon', age: 23, id: 1 }
})
})
Read a node.
Arguments
node
is an object containing the properties
of the node with the given id.Example
db.save({ make: 'Citroen', model: 'DS4' }, function(err, node) {
db.read(node.id, function(err, node) {
console.log(node) // -> { make: 'Citroen', model: 'DS4', id: 1 }
})
})
Delete a node.
Arguments
err
is falsy, the node has been deleted.Example
db.save({ name: 'Jon' }, function(err, node) {
db.delete(node, function(err) {
if (!err) console.log('Jon has been deleted!');
})
})
Perform a query based on a predicate. The predicate is translated to a cypher query.
Arguments
false
) - If true, elements need only match on one
attribute. If false, elements must match on all attributes.results
is an array of the resulting
nodes.Example
Given database content:
{ name: 'Jon' , age: 23, australian: true }
{ name: 'Neil' , age: 60, australian: true }
{ name: 'Belinda', age: 26, australian: false }
{ name: 'Katie' , age: 29, australian: true }
Retrieve all australians:
var predicate = { australian: true };
var people = db.find(predicate, function (err, objs) {
if (err) throw err;
assert.equals(3, people.length);
};
Read the relationships involving the specified node.
Arguments
type
is passed,
default='all'
) - the direction of relationships to read.''
(match all relationships)) - the relationship
type to findrelationships
is an array of the
matching relationshipsExample
db.relationships(452, 'out', 'knows', function(err, relationships) {
// relationships = all outgoing `knows` relationships from node 452
})
Create a relationship between two nodes.
Arguments
{}
) - properties of the relationshiprelationship
is the newly created
relationshipExample
db.relate(1, 'knows', 2, { for: '2 months' }, function(err, relationship) {
assert.deepEqual(relationship, {
start: 1,
end: 2,
type: 'knows',
properties: { for: '2 months' },
id: 1
});
});
Update the properties of a relationship. Note that you cannot use this method to update the base properties of the relationship (start, end, type) - in order to do that you'll need to delete the old relationship and create a new one.
Arguments
Example
var props = { for: '2 months', location: 'Bergen' };
db.rel.create(1, 'knows', 2, props, function(err, relationship) {
delete relationship.properties.location;
relationship.properties.for = '3 months';
db.rel.update(relationship, function(err) {
// properties on this relationship in the database are now equal to
// { for: '3 months' }
});
});
Read a relationship.
Arguments
relationship
is an object
representing the read relationship.Example
db.rel.create(1, 'knows', 2, { for: '2 months' }, function(err, newRelationship) {
db.rel.read(newRelationship.id, function(err, readRelationship) {
assert.deepEqual(newRelationship, readRelationship);
assert.deepEqual(readRelationship, {
start: 1,
end: 2,
type: 'knows',
id: 1,
properties: { for: '2 months' }
});
});
});
Delete a relationship.
Arguments
err
is falsy, the relationship has been
deleted.Example
db.rel.create(1, 'knows', 2, { for: '2 months' }, function(err, rel) {
db.rel.delete(rel.id, function(err) {
if (!err) console.log("Relationship was deleted");
});
});
Create a new index. If you're using the default index configuration, this method is not necessary - you can just start using the index with index.add as if it already existed.
NOTE for index functions: there are two different types on index in neo4j -
node indexes and relationship indexes. When you're working with node
indexes, you use the functions on node.index
. Similarly, when you're working
on relationship indexes you use the functions on rel.index
. All of the
functions on both of these are identical, but one acts upon node
indexes, and the other upon relationship indexes.
Arguments
{}
) - the configuration of the index. See the neo4j docs
for more information.err
is falsy, the index has been created.Example
var indexConfig = { type: 'fulltext', provider: 'lucene' };
db.node.index.create('a_fulltext_index', indexConfig, function(err) {
if (!err) console.log('a fulltext index has been created!');
});
Add a node/relationship to an index.
NOTE for index functions: there are two different types on index in neo4j -
node indexes and relationship indexes. When you're working with node
indexes, you use the functions on node.index
. Similarly, when you're working
on relationship indexes you use the functions on node.rel
. All of the
functions on both of these are identical, but one acts upon node
indexes, and the other upon relationship indexes.
Arguments
err
is falsy, the node/relationship has
been indexed.Example
db.save({ name: 'Jon', }, function(err, node) {
db.index('people', node, 'name', node.name, function(err) {
if (!err) console.log('Jon has been indexed!');
});
});
Read the object(s) from an index that match a key-value pair.
NOTE for index functions: there are two different types on index in neo4j -
node indexes and relationship indexes. When you're working with node
indexes, you use the functions on node.index
. Similarly, when you're working
on relationship indexes you use the functions on node.rel
. All of the
functions on both of these are identical, but one acts upon node
indexes, and the other upon relationship indexes.
Arguments
results
is a node or relationship object
(or an array of them if there was more than one) that matched the given
key-value pair in the given index. If nothing matched, results === false
.Example
db.rel.index.read('friendships', 'location', 'Norway', function(err, rels) {
// `rels` is an array of all relationships indexed in the `friendships`
// index, with a value `Norway` for the key `location`.
});
Remove a node/relationship from an index.
NOTE for index functions: there are two different types on index in neo4j -
node indexes and relationship indexes. When you're working with node
indexes, you use the functions on node.index
. Similarly, when you're working
on relationship indexes you use the functions on node.rel
. All of the
functions on both of these are identical, but one acts upon node
indexes, and the other upon relationship indexes.
Arguments
err
is falsy, the specified references have
been removed.Example
db.node.index.remove(6821, 'people', function(err) {
if (!err) console.log("Every reference of node 6821 has been removed from the people index");
});
db.rel.index.remove(351, 'friendships', 'in', 'Australia', function(err) {
if (!err) console.log("Relationship 351 is no longer indexed as a friendship in Australia");
})
Delete an index.
NOTE for index functions: there are two different types on index in neo4j -
node indexes and relationship indexes. When you're working with node
indexes, you use the functions on node.index
. Similarly, when you're working
on relationship indexes you use the functions on node.rel
. All of the
functions on both of these are identical, but one acts upon node
indexes, and the other upon relationship indexes.
Arguments
err
is falsy, the index has been deleted.Example
db.rel.index.delete('friendships', function(err) {
if (!err) console.log('The `friendships` index has been deleted');
})
FAQs
A thin and familiar layer between node and neo4j's REST api.
The npm package seraph receives a total of 95 weekly downloads. As such, seraph popularity was classified as not popular.
We found that seraph demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.