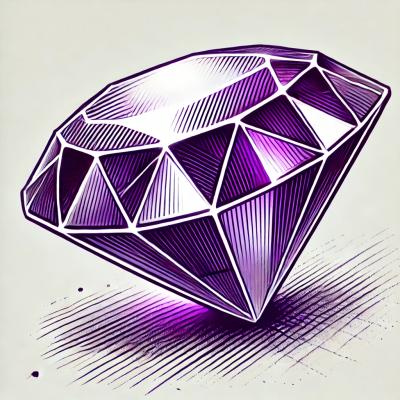
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
serialport
Advanced tools
eeeee eeeee eeeee eeee e eeeee 8 8 8 88 8 8 8 8 8 " 8e 8 8 8 8e 8 8eee 8e 8eeee 88 8 8 8 88 8 88 e 88 88 88 8 8eee8 88ee8 88ee 88 8ee88 8ee88 eeeee eeee eeeee e eeeee e eeeee eeeee eeeee eeeee 8 " 8 8 8 8 8 8 8 8 8 8 88 8 8 8 8eeee 8eee 8eee8e 8e 8eee8 8e 8eee8 8 8 8eee8e 8e 88 88 88 8 88 88 8 88 88 8 8 88 8 88 8ee88 88ee 88 8 88 88 8 88eee 88 8eee8 88 8 88
Version: 0.2.2 - Released March 19, 2011
Imagine a world where you can write JavaScript to control blenders, lights, security systems, or even robots. Yes, I said robots. That world is here and now with node-serialport. It provides a very simple interface to the low level serial port code necessary to program Arduino chipsets, X10 wireless communications, or even the rising Z-Wave and Zigbee standards. The physical world is your oyster with this goodie, don't believe us - watch this presentation from JSConf EU 2010 by Nikolai Onken and Jörn Zaefferer.
Using node-serialport is pretty easy because it is pretty basic. It provides you with the building block to make great things, it is not a complete solution - just a cog in the (world domination) machine.
npm install serialport
Opening a serial port:
var SerialPort = require("serialport").SerialPort var serialPort = new SerialPort("/dev/tty-usbserial1");
When opening a serial port, you can specify (in this order).
The options object allows you to pass named options to the serial port during initialization. The valid attributes for the options object are the following
Out of the box, node-serialport provides two parsers one that simply emits the raw buffer as a data event and the other which provides familiar "readline" style parsing. To use the readline parser, you must provide a delimiter as such:
var serialport = require("serialport"); var SerialPort = serialport.SerialPort; // localize object constructor var sp = new SerialPort("/dev/tty-usbserial1", { parser: serialport.parsers.readline("\n") });
To use the raw parser, you just provide the function definition (or leave undefined):
var serialport = require("serialport"); var SerialPort = serialport.SerialPort; // localize object constructor var sp = new SerialPort("/dev/tty-usbserial1", { parser: serialport.parsers.raw });
You can get updates of new data from the Serial Port as follows:
serialPort.on("data", function (data) { sys.puts("here: "+data); });
You can write to the serial port by sending a string or buffer to the write method as follows:
serialPort.write("OMG IT WORKS\r");
Enjoy and do cool things with this code.
Version 0.2.2
FAQs
Node.js package to access serial ports. Linux, OSX and Windows. Welcome your robotic JavaScript overlords. Better yet, program them!
The npm package serialport receives a total of 0 weekly downloads. As such, serialport popularity was classified as not popular.
We found that serialport demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.