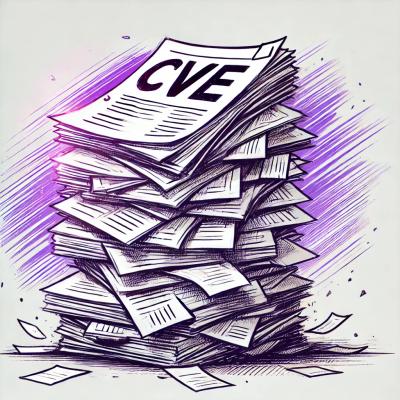
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
shape-collection
Advanced tools
Utility for manipulating data in arrays of objects.
Run npm install shape-collection --save
import { shape } from 'shape-collection';
const arr = [{
users: ['aa', 'bb']
}, {
users: ['kk', 'zz', 'xyz']
}];
const users = shape(arr).reduceTo('users').fetch();
// users contains:
// ["aa", "bb", "kk", "zz", "xyz"]
const arr = [{ id: 'abc' }, { id: 'xyz' }]
shape(arr).filterByProp('id', 'abc').fetchIndex(0);
Function
. Returns the shaped collection.
Function
. Returns item at a specific index from the shaped collection.
Function
. Filters the collection by unique value:
import { shape } from 'shape-collection';
const arr = [{
id: 1,
users: ['aa', 'bb']
}, {
id: 2,
users: ['kk', 'zz', 'xyz']
}];
const user = shape(arr).filterByUnique('id', 1).fetchIndex(0);
// user contains:
// {id: 1, users: ['aa', 'bb']}
Function
. Filter and extract duplicate items from collection:
const arr = [{
id: 1,
type: 'b',
users: ['aa', 'bb']
}, {
id: 1,
type: 'a',
users: ['kk', 'zz', 'xyz']
}, , {
id: 3,
type: 'a',
users: ['kk', 'zz', 'xyz']
}];
const duplicates = shape(arr).filterByDuplicate('id').fetch();
// duplicates contains:
// [{ id: 1, type: 'b' ... }, { id: 1, type: 'a' ... }]
Function
. Filter collection by property value:
const arr = [{
id: 1,
type: 'b',
users: ['aa', 'bb']
}, {
id: 1,
type: 'a',
users: ['kk', 'zz', 'xyz']
}, , {
id: 3,
type: 'a',
users: ['kk', 'zz', 'xyz']
}];
const item = shape(arr).filterByProp('id', 3).fetch();
// item contains:
// [{ id: 3, type: 'a' ... }]
Function
. Sort collection by key, type and direction:
const arr = [{
id: 1,
type: 'b',
users: ['aa', 'bb']
}, {
id: 1,
type: 'a',
users: ['kk', 'zz', 'xyz']
}, , {
id: 3,
type: 'a',
users: ['kk', 'zz', 'xyz']
}];
const sortedById = shape(arr).sortBy({
key: 'id', // string, **REQUIRED**
type: 'number', // string, default = 'string'
direction = 'desc' // string, defaul = 'asc'
}).fetch();
// sortedById contains:
// [{ id: 3 ... }, { id: 1 ... }, { id: 1 ... }]
Available options for sortBy
are:
type
number
string
date
- new Date() fieldscombo
- combination of two string fieldsdirection
asc
desc
Function
. Reduce collection to another collection:
const arr = [{
id: 1,
type: 'b',
users: ['aa', 'bb']
}, {
id: 2,
type: 'a',
users: ['kk', 'zz', 'xyz']
}, {
id: 3,
type: 'a',
users: ['ff', 'hhh', 'eeee', 'kk']
}];
const users = shape(arr).reduceTo('users').fetch();
// users contains:
// ["aa", "bb", "kk", "zz", "xyz", "ff", "hhh", "eeee", "kk"]
const groups = [{
id: 1,
type: 'day_group',
events: {
summerBreak: {
startDate: '22/06/2018',
endDate: '22/09/2018'
},
winterBreak: {
startDate: '22/12/2018',
endDate: '02/01/2019'
}
},
users: [{
id: 'abc',
name: 'John Doe',
age: 33,
exams: [{
name: 'English B1',
score: 5
}, {
name: 'Exam B',
score: 10
}]
}, {
id: 'xyz',
name: 'Teddy Williams',
age: 42,
exams: [{
name: 'English B1',
score: 6
}, {
name: 'Exam B',
score: 3
}]
}, {
id: 'zzz',
name: 'Jake McCoy',
age: 14,
exams: []
}]
}, {
id: 2,
type: 'evening_group',
events: {
summerBreak: {
startDate: '12/06/2018',
endDate: '12/09/2018'
},
winterBreak: {
startDate: '01/12/2018',
endDate: '01/02/2019'
}
},
users: [{
id: 'yyy',
name: 'Teddy Smith',
age: 23,
exams: [{
name: 'English B1',
score: 3
}, {
name: 'Exam B',
score: 7
}]
}, {
id: 'jjj',
name: 'Jane Doe',
age: 42,
exams: [{
name: 'English B1',
score: 4
}, {
name: 'Exam B',
score: 1
}]
}]
}, {
id: 3,
type: 'weekend_group',
users: []
}];
const examsSortedByName = shape(groups)
.reduceTo('users')
.reduceTo('exams')
.sortBy('name')
.fetch();
// examsSortedByName contains
/*
[
{name: "English B1", score: 5},
{name: "English B1", score: 6},
{name: "English B1", score: 3},
{name: "English B1", score: 4},
{name: "Exam B", score: 10},
{name: "Exam B", score: 3},
{name: "Exam B", score: 7},
{name: "Exam B", score: 1}
]
*/
const examsSortedByScore = shape(groups)
.reduceTo('users')
.reduceTo('exams')
.sortBy('score', 'number', 'desc')
.fetch();
// examsSortedByScore contains
/*
[
{name: "Exam B", score: 10},
{name: "Exam B", score: 7},
{name: "English B1", score: 6},
{name: "English B1", score: 5},
{name: "English B1", score: 4},
{name: "Exam B", score: 3},
{name: "English B1", score: 3},
{name: "Exam B", score: 1}
]
*/
// getting nested props from object fields, using comma-separated keys
const summerBreaks = shape(groups).reduceTo('events.summerBreak').fetch();
// summerBreaks contains
/*
[
{startDate: "22/06/2018", endDate: "22/09/2018"},
{startDate: "12/06/2018", endDate: "12/09/2018"}
]
*/
FAQs
Utility for manipulating data in arrays of objects
The npm package shape-collection receives a total of 8 weekly downloads. As such, shape-collection popularity was classified as not popular.
We found that shape-collection demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.