sheetify
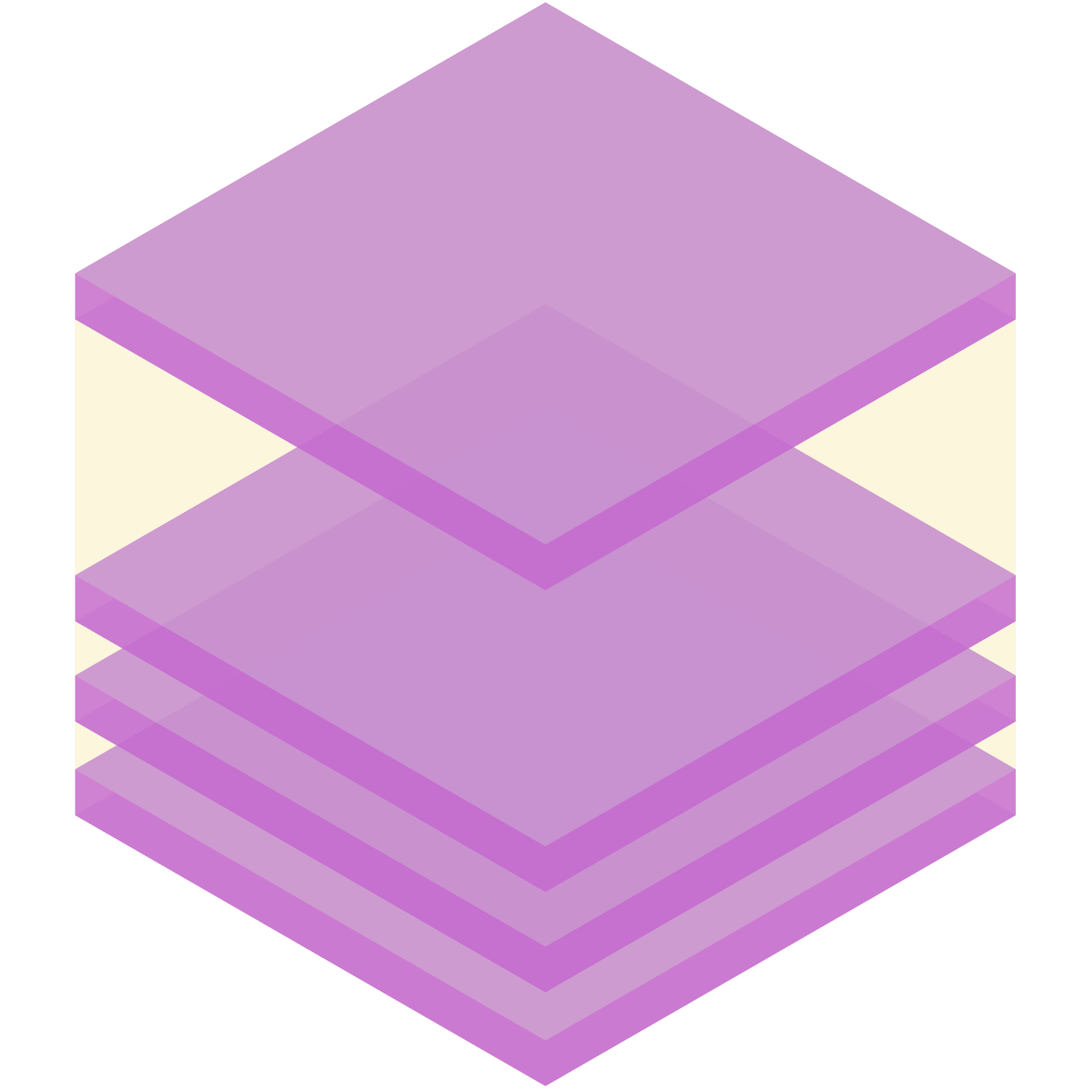

Modular CSS bundler for browserify. Works with npm modules
like browserify does.
Features
- clarity: namespace CSS, no more need for naming schemes
- modularity: import and reuse CSS packages from npm
- extensibility: transform CSS using existing plugins, or write your own
- transparency: inline CSS in your views
- simplicity: tiny API surface and a minimal code base
Example
Given some inline CSS:
const vdom = require('virtual-dom')
const hyperx = require('hyperx')
const sf = require('sheetify')
const hx = hyperx(vdom.h)
const prefix = sf`
h1 {
text-align: center;
}
`
const tree = hx`
<section className=${prefix}>
<h1>My beautiful, centered title</h1>
</section>
`
document.body.appendChild(vdom.create(tree))
Compile with browserify using -t sheetify/transform
:
$ browserify -t sheetify/transform index.js > bundle.js
CSS classes are namespaced based on the content hash:
._60ed23ec9f h1 {
text-align: center;
}
And the rendered HTML includes the namespace:
<section class="._60ed23e">
<h1>My beautiful, centered title</h1>
</section>
External files
To include an external CSS file you can pass a path to sheetify as
sheetify('./my-file.css')
:
const vdom = require('virtual-dom')
const hyperx = require('hyperx')
const sf = require('sheetify')
const hx = hyperx(vdom.h)
const prefix = sf('./my-styles.css')
const tree = hx`
<section className=${prefix}>
<h1>My beautiful, centered title</h1>
</section>
`
document.body.appendChild(vdom.create(tree))
Use npm packages
To consume a package from npm that has .css
file in its main
or style
field:
const sf = require('sheetify')
sf('normalize.css')
Packages from npm will not be namespaced by default.
Toggle namespaces
To toggle namespaces for external files or npm packages:
const sf = require('sheetify')
sf('./my-file.css', { global: true })
Write to separate file on disk
To write the compiled CSS to a separate file, rather than keep it in the
bundle:
$ browserify -t [ sheetify/transform -o bundle.css ] index.js > bundle.js
Write to stream
To write the compiled CSS to a stream:
const browserify = require('browserify')
const fs = require('fs')
const b = browserify(path.join(__dirname, 'transform/source.js'))
const ws = fs.createWriteStream('/bundle.css')
b.transform('sheetify', { out: ws })
const r = b.bundle().pipe(fs.createWriteStream('./bundle.js'))
r.on('end', () => ws.end())
Browserify transforms don't know when browserify
is done, so we have to
attach a listener on browserify for the 'end'
event to close the writeStream
accordingly.
Plugins
Sheetify uses plugins that take CSS and apply a transform.
For example include
sheetify-cssnext to support
autoprefixing, variables and more:
const vdom = require('virtual-dom')
const hyperx = require('hyperx')
const sf = require('sheetify')
const hx = hyperx(vdom.h)
const prefix = sf`
h1 {
transform: translate(0, 0);
}
`
const tree = hx`
<section className=${prefix}>
<h1>My beautiful, centered title</h1>
</section>
`
document.body.appendChild(vdom.create(tree))
Compile with browserify using -t [ sheetify/transform -u sheetify-cssnext ]
:
$ browserify -t [ sheetify/transform -u sheetify-cssnext ] index.js > bundle.js
Transforms the CSS into:
h1 {
-webkit-transform: translate(0, 0);
transform: translate(0, 0);
}
The following plugins are available:
API
Browserify transforms accept either flags from the command line using
subargs:
$ browserify -t [ sheetify/transform -o bundle.css ] index.js > bundle.js
Or the equivalent options by passing in a configuration object in the
JavaScript API:
const browserify = require('browserify')
const b = browserify(path.join(__dirname, 'transform/source.js'))
b.transform('sheetify', { out: path.join(__dirname, '/bundle.css') })
b.bundle().pipe(process.stdout)
The following options are available:
Options:
-o, --out Specify an output file
-u, --use Consume a sheetify plugin
Installation
$ npm install sheetify
License
MIT