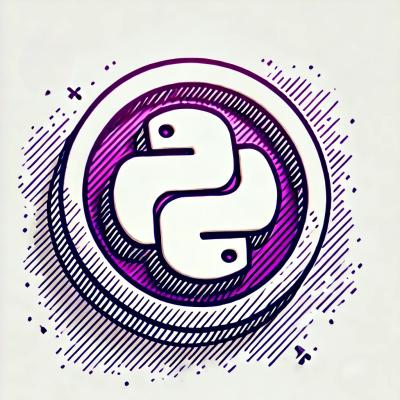
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
shopify-api-node
Advanced tools
Shopify API bindings for Node.js.
$ npm install --save shopify-api-node
This module exports a constructor function which takes an options object.
Shopify(options)
Creates a new Shopify
instance.
options
- Required - A plain JavaScript object that contains the
configuration options.shopName
- Required - A string that specifies the shop name. The shop's
"myshopify.com" domain is also accepted.apiKey
- Required for private apps - A
string that specifies the API key of the app. This option must be used in
conjunction with the password
option and is mutually exclusive with the
accessToken
option.password
- Required for private apps - A
string that specifies the private app password. This option must be used in
conjunction with the apiKey
option and is mutually exclusive with the
accessToken
option.accessToken
- Required for public apps - A string representing the permanent
OAuth 2.0 access token. This option is mutually exclusive with the
apiKey
and password
options. If you are looking for a premade solution to
obtain an access token, take a look at the shopify-token module.apiVersion
- Optional - A string to specify the Shopify API
version to use for requests. Defaults to the oldest supported
stable version.autoLimit
- Optional - This option allows you to regulate the request rate
in order to avoid hitting the rate limit. Requests are
limited using the token bucket algorithm. Accepted values are a boolean or a
plain JavaScript object. When using an object, the calls
property and the
interval
property specify the refill rate and the bucketSize
property the
bucket size. For example { calls: 2, interval: 1000, bucketSize: 35 }
specifies a limit of 2 requests per second with a burst of 35 requests. When
set to true
requests are limited as specified in the above example. Defaults
to false
.parseJson
- Optional - The function used to parse JSON. The function is
passed a single argument. This option allows the use of a custom JSON parser
that might be needed to properly handle long integer IDs. Defaults to
JSON.parse()
.presentmentPrices
- Optional - Whether to include the header to pull
presentment prices for products. Defaults to false
.stringifyJson
- Optional - The function used to serialize to JSON. The
function is passed a single argument. This option allows the use of a custom
JSON serializer that might be needed to properly handle long integer IDs.
Defaults to JSON.stringify()
.timeout
- Optional - The number of milliseconds before the request times
out. If the request takes longer than timeout
, it will be aborted. Defaults
to 60000
, or 1 minute.A Shopify
instance.
Throws an Error
exception if the required options are missing.
const Shopify = require('shopify-api-node');
const shopify = new Shopify({
shopName: 'your-shop-name',
apiKey: 'your-api-key',
password: 'your-app-password'
});
shopify.callLimits
The callLimits
property allows you to monitor the API call limit. The value is
an object like this:
{
remaining: 30,
current: 10,
max: 40
}
Values start at undefined
and are updated every time a request is made. After
every update the callLimits
event is emitted with the updated limits as
argument.
shopify.on('callLimits', (limits) => console.log(limits));
When using the GraphQL API, a different property is used to track the API call
limit: callGraphqlLimits
.
Keep in mind that the autoLimit
option is ignored while using GraphQL API.
shopify.on('callGraphqlLimits', (limits) => console.log(limits));
Every resource is accessed via your shopify
instance:
const shopify = new Shopify({
shopName: 'your-shop-name',
accessToken: 'your-oauth-token'
});
// shopify.<resource_name>.<method_name>
Each method returns a Promise
that resolves with the result:
shopify.order
.list({ limit: 5 })
.then((orders) => console.log(orders))
.catch((err) => console.error(err));
The Shopify API requires that you send a valid JSON string in the request body including the name of the resource. For example, the request body to create a country should be:
{
"country": {
"code": "FR",
"tax": 0.25
}
}
When using shopify-api-node
you don't have to specify the full object but only
the nested one as the module automatically wraps the provided data. Using the
above example this translates to:
shopify.country
.create({ code: 'FR', tax: 0.25 })
.then((country) => console.log(country))
.catch((err) => console.error(err));
Similarly, the Shopify API includes the resource name in the JSON string that is returned in the response body:
{
"country": {
"id": 1070231510,
"name": "France",
"tax": 0.2,
"code": "FR",
"tax_name": "TVA",
"provinces": []
}
}
shopify-api-node
automatically unwraps the parsed object and returns:
{
id: 1070231510,
name: 'France',
tax: 0.2,
code: 'FR',
tax_name: 'TVA',
provinces: []
}
This behavior is valid for all resources.
Shopify allows for adding metafields to various resources. You can use the
owner_resource
and owner_id
properties to work with metafields that belong
to a particular resource as shown in the examples below.
Get metafields that belong to a product:
shopify.metafield
.list({
metafield: { owner_resource: 'product', owner_id: 632910392 }
})
.then(
(metafields) => console.log(metafields),
(err) => console.error(err)
);
Create a new metafield for a product:
shopify.metafield
.create({
key: 'warehouse',
value: 25,
value_type: 'integer',
namespace: 'inventory',
owner_resource: 'product',
owner_id: 632910392
})
.then(
(metafield) => console.log(metafield),
(err) => console.error(err)
);
Pagination in API version 2019-07 and above can be done as shown in the following example:
(async () => {
let params = { limit: 10 };
do {
const products = await shopify.product.list(params);
console.log(products);
params = products.nextPageParameters;
} while (params !== undefined);
})().catch(console.error);
Each set of results may have the nextPageParameters
and
previousPageParameters
properties. These properties specify respectively the
parameters needed to fetch the next and previous page of results.
This feature is only available on version 2.24.0 and above.
list()
delete()
activate(id[, params])
create(params)
get(id[, params])
list([params])
create(params)
get(id[, params])
list([params])
authors()
count(blogId[, params])
create(blogId, params)
delete(blogId, id)
get(blogId, id[, params])
list(blogId[, params])
tags([blogId][, params])
update(blogId, id, params)
create(themeId, params)
delete(themeId, params)
get(themeId, params)
list(themeId[, params])
update(themeId, params)
list()
transactions([params])
count()
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
accept(fulfillmentOrderId[, message])
create(fulfillmentOrderId[, message])
reject(fulfillmentOrderId[, message])
create(params)
delete(id)
get(id)
list()
update(id, params)
complete(token)
count([params])
create(params)
get(token)
list([params])
shippingRates(token)
update(token, params)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
get(id[, params])
products(id[, params])
get(id)
list([params])
productIds(id[, params])
approve(id)
count([params])
create(params)
get(id[, params])
list([params])
notSpam(id)
remove(id)
restore(id)
spam(id)
update(id, params)
count()
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
list()
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
accountActivationUrl(id)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
orders(id[, params])
search(params)
sendInvite(id[, params])
update(id, params)
create(customerId, params)
default(customerId, id)
delete(customerId, id)
get(customerId, id)
list(customerId[, params])
set(customerId, params)
update(customerId, id, params)
count([params])
create(params)
customers(id[, params])
delete(id)
get(id[, params])
list([params])
update(id, params)
create(priceRuleId, params)
delete(priceRuleId, id)
get(priceRuleId, id)
list(priceRuleId)
lookup(params)
update(priceRuleId, id, params)
create(priceRuleId, params)
discountCodes(priceRuleId, id)
get(priceRuleId, id)
get(id)
list([params])
complete(id[, params])
count()
create(params)
delete(id)
get(id[, params])
list([params])
sendInvoice(id[, params])
update(id, params)
count([params])
get(id[, params])
list([params])
cancel(orderId, id)
complete(orderId, id)
count(orderId[, params)
create(orderId, params)
createV2(params)
get(orderId, id[, params])
list(orderId[, params])
open(orderId, id)
update(orderId, id, params)
updateTracking(id, params)
create(orderId, fulfillmentId, params)
delete(orderId, fulfillmentId, id)
get(orderId, fulfillmentId, id)
list(orderId, fulfillmentId[, params])
update(orderId, fulfillmentId, id, params)
cancel(id, params)
close(id[, message])
get(id)
list([params])
locationsForMove(id)
move(id, locationId)
accept(fulfillmentOrderId[, message])
create(fulfillmentOrderId, params)
reject(fulfillmentOrderId[, message])
create(params)
delete(id)
get(id)
list([params])
update(id, params)
count([params])
create(params)
disable(id)
get(id)
list([params])
search(params)
update(id, params)
create(giftCardId, params)
get(giftCardId, id)
list(giftCardId)
get(id)
list(params)
update(id, params)
adjust(params)
connect(params)
delete(params)
list(params)
set(params)
count
get(id)
inventoryLevels(id[, params])
list()
count()
create(params)
delete(id)
get(id)
list([params])
update(id, params)
engagements(id, params)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
cancel(id[, params])
close(id)
count([params])
create(params)
delete(id)
fulfillmentOrders(id)
get(id[, params])
list([params])
open(id)
update(id, params)
create(orderId, params)
delete(orderId, id)
get(orderId, id)
list(orderId)
update(orderId, id, params)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
count(checkoutToken)
create(checkoutToken, params)
get(checkoutToken, id)
list(checkoutToken)
get(id)
list([params])
list([params])
create(params)
delete(id)
get(id)
list([params])
update(id, params)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
count(productId[, params])
create(productId, params)
delete(productId, id)
get(productId, id[, params])
list(productId[, params])
update(productId, id, params)
count()
create(productId[, params])
delete(productId)
get(productId)
list([params])
productIds([params])
create(productId[, params])
list(productId)
count(productId)
create(productId, params)
delete(productId, id)
get(id[, params])
list(productId[, params])
update(id, params)
count(countryId[, params])
get(countryId, id[, params])
list(countryId[, params])
update(countryId, id, params)
activate(id, params)
create(params)
delete(id)
get(id[, params])
list([params])
customize(id, params)
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
calculate(orderId, params)
create(orderId, params)
get(orderId, id[, params])
list(orderId[, params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
create(params)
list()
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
list([params])
get([params])
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
order(id, params)
products(id[, params])
update(id, params)
create(params)
delete(id)
list()
list([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
count(orderId)
create(orderId, params)
get(orderId, id[, params])
list(orderId[, params])
create(recurringApplicationChargeId, params)
get(recurringApplicationChargeId, id[, params])
list(recurringApplicationChargeId[, params])
current()
get(id)
list()
count([params])
create(params)
delete(id)
get(id[, params])
list([params])
update(id, params)
where params
is a plain JavaScript object. See
https://help.shopify.com/api/reference?ref=microapps for parameters details.
The shopify
instance also allows to use the GraphQL API through the graphql
method, which returns a promise that resolves with the result data:
const shopify = new Shopify({
shopName: 'your-shop-name',
accessToken: 'your-oauth-token'
});
const query = `{
customers(first: 5) {
edges {
node {
displayName
totalSpent
}
}
}
}`;
shopify
.graphql(query)
.then((customers) => console.log(customers))
.catch((err) => console.error(err));
(add yours!)
Used in our live products: MoonMail & MONEI
FAQs
Shopify API bindings for Node.js
The npm package shopify-api-node receives a total of 26,949 weekly downloads. As such, shopify-api-node popularity was classified as popular.
We found that shopify-api-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.