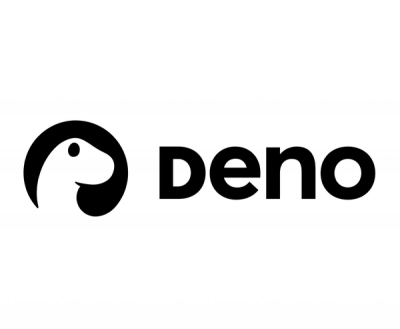
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Super performant and feature rich shortcuts management library.
This library is just a thin wrapper over ShoSho
that provides a VSCode-like interface for managing shortcuts. Definitely check out ShoSho
, which provides more features and it may offer a lower-level but maybe more convenient API for your use case.
npm install --save shortcuts
The following interface is provided:
type Descriptor = {
handler?: ( event?: Event ): boolean | void,
shortcut: string
};
type Disposer = {
(): void
};
type Options = {
capture?: boolean,
target?: Window | Document | HTMLElement | SVGElement | MathMLElement,
shouldHandleEvent?: ( event: Event ) => boolean
};
class Shortcuts {
constructor ( options: Options );
get (): Descriptor[];
add ( descriptor: Descriptor | Descriptor[] ): void;
register ( descriptor: Descriptor | Descriptor[] ): Disposer;
remove ( descriptor: Descriptor | Descriptor[] ): void;
reset (): void;
trigger ( shortcut: string ): boolean;
start (): void;
stop (): void;
}
This is how you'd use the library:
import {Shortcuts} from 'shortcuts';
// Let's create a new shortcuts manager instance
const shortcuts = new Shortcuts ({
capture: true, // Handle events during the capturing phase
target: document, // Listening for events on the document object
shouldHandleEvent ( event ) {
return true; // Handle all possible events
}
});
// Let's register and unregister some shortcuts
// Handlers are filtered by shortcut and executed from bottom to top, stopping at the first handler that returns true
const onCtrlB = () => {};
shortcuts.add ([
{
shortcut: 'Ctrl+A',
handler: () => {
// Doing something...
return true; // Returning true if we don't want other handlers for the same shortcut to be called later
}
},
{
shortcut: 'Ctrl+B',
handler: onCtrlB
},
{
shortcut: 'CmdOrCtrl+K Shift+B',
handler: () => {
// Doing something...
return true; // Returning true if we don't want other handlers for the same shortcut to be called later
}
},
{
shortcut: '-Ctrl+A' // Removing all previously-registered handlers for "Ctrl+A"
}
]);
shortcuts.remove ({ shortcut: 'Ctrl+B', handler: onCtrlB }); // Removing a specific handler bound to this shortcut
shortcuts.remove ({ shortcut: 'Ctrl+A' }); // Removing all handlers bound to this shortcut
// Let's actually start listening for shortcuts
shortcuts.start ();
// Let's stop listening for shortcuts
shortcuts.stop ();
// Let's dispose of all registered shortcuts
shortcuts.reset ();
shortcuts
package name on NPM! If you're looking for the previous package published under that name you can find it here (v0.x
).MIT © Fabio Spampinato
FAQs
Super performant and feature rich shortcuts management library.
The npm package shortcuts receives a total of 1,457 weekly downloads. As such, shortcuts popularity was classified as popular.
We found that shortcuts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.