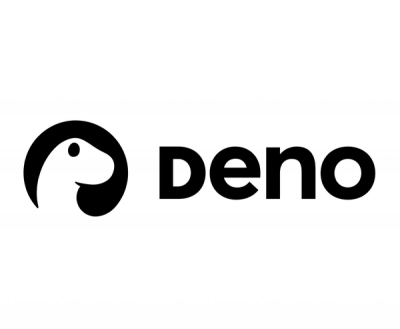
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This library provides a client for the Signalwire LaML and REST services.
It allows you to create calls, send messages, and generate LAML responses.
Install the package using NPM:
npm install signalwire
In order to use the client you must set the environment variable SIGNALWIRE_API_HOSTNAME
!
Puts in your .env
file your SignalWire host, project and token:
SIGNALWIRE_API_HOSTNAME=changeme.signalwire.com
SIGNALWIRE_API_PROJECT=XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
SIGNALWIRE_API_TOKEN=PTXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
// Here we are using Project and Token from ENV
const RestClient = require('signalwire').RestClient
const client = new RestClient(process.env.SIGNALWIRE_API_PROJECT, process.env.SIGNALWIRE_API_TOKEN)
client.calls.create({
to: '+19999999999', // Call this number
from: '+18888888888', // From a valid SignalWire number
url: 'https://example.com/laml/voice.xml' // Valid LaML
}).then(call => {
process.stdout.write('Call ID: ' + call.sid)
}).catch(error => {
// Inspecting error...
})
client.messages.create({
body: 'Welcome to SignalWire!',
to: '+19999999999', // Text this number
from: '+18888888888' // From a valid SignalWire number
}).then(message => {
process.stdout.write('Message ID: ' + message.sid)
}).catch(error => {
// Inspecting error...
})
const RestClient = require('signalwire').RestClient
const response = new RestClient.LaML.VoiceResponse()
response.dial({ callerId: '+18888888888' }, '+19999999999')
response.say("Welcome to SignalWire!")
process.stdout.write(response.toString())
LaML output:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Dial callerId="+18888888888">+19999999999</Dial>
<Say>Welcome to SignalWire!</Say>
</Response>
Do you want to start using SignalWire in your current application? You can easily migrate the code with minimal changes!
Make sure you've set the env variable SIGNALWIRE_API_HOSTNAME
as described in Usage and then:
To use the Rest client:
// Replace these lines:
const twilio = require('twilio')
const client = new twilio(sid, token)
// With ...
const signalwire = require('signalwire')
const client = new signalwire.RestClient(project, token)
// Now use client variable like you did before!
For calls and messages you should also change the
from
numbers with a valid SignalWire number!
To generate LaML
:
// Replace these lines..
const twilio = require('twilio')
const response = new twilio.twiml.VoiceResponse()
// With ..
const signalwire = require('signalwire')
const response = new signalwire.RestClient.LaML.VoiceResponse()
// Now use response like you did before!
response.say('Hey, Welcome at SignalWire!')
A Dockerfile is provided for testing purposes. Run docker run -it $(docker build -q .)
to execute the test suite.
Copyright (c) 2018 SignalWire Inc. See LICENSE for further details.
FAQs
Client library for connecting to SignalWire.
The npm package signalwire receives a total of 39 weekly downloads. As such, signalwire popularity was classified as not popular.
We found that signalwire demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.