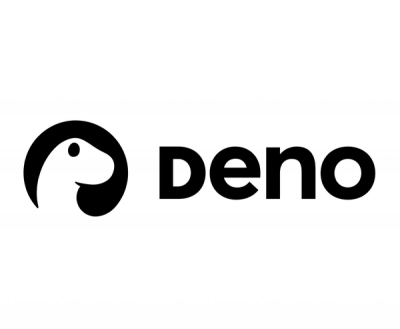
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
simple-pdf-generator
Advanced tools
Generator of PDF files from HTML templates using TS decorators
Node module that converts HTML5 files to PDFs.
This is a Node.js module available through the npm registry. Before installing, download and install Node.js.
Installation is done using the npm install command:
$ npm install simple-pdf-generator
Simple PDF Generator:
In order to have a template you must create a class that extends the PdfFiller
abstract class:
import path from 'path';
import { PdfField, PdfFiller, PdfTable, PdfTemplate } from 'simple-pdf-generator';
@PdfTemplate({
templatePath: path.join(__dirname, 'template.html'),
})
export class Template extends PdfFiller {
@PdfField()
firstField = '';
@PdfField()
secondField = '';
}
And add the HTML file:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div class="container-fluid">
<div class="container">
<div class="row justify-content-center">
<div class="col-10 text-center">
<h1>Hello %%firstField%%</h1>
<h2>Welcome in %%secondField%%</h2>
</div>
</div>
</div>
</div>
</body>
</html>
Then it's finally possible to use the template by calling the fill()
method in order to generate the PDF:
import path from 'path';
import { Template } from './Template';
(async () => {
const doc = new Template();
doc.firstField = 'World';
doc.secondField = 'Simple PDF Generator';
await doc.fill(path.join(__dirname, 'doc.pdf'));
})();
To generate a table you must to use the PdfTable
decorator on your data property:
import path from 'path';
import { PdfField, PdfFiller, PdfTable, PdfTemplate } from 'simple-pdf-generator';
interface TableRow {
index: number;
name: string;
surname: string;
email: string;
}
@PdfTemplate({
templatePath: path.join(__dirname, 'template.html'),
})
export class Template extends PdfFiller {
@PdfField()
field = '';
@PdfTable()
data = new Array<TableRow>();
}
In the HTML file write this:
<inject-table items="data" class="table">
<inject-column prop="index" label="#" />
<inject-column prop="name" label="Name" />
<inject-column prop="surname" label="Surname" />
<inject-column prop="email" label="Email" />
</inject-table>
And then, as above, use the fill()
method like this:
import path from 'path';
import { Template } from './Template';
(async () => {
const doc = new Template();
doc.field = 'World';
doc.tableData = [
{ index: 1, name: 'James', surname: 'Smith', email: 'james@smith.com' },
{ index: 2, name: 'Robert', surname: 'Johnson', email: 'robert@johnson.com' },
];
await doc.fill(path.join(__dirname, 'doc.pdf'));
})();
Through the class decorator is possible to include CSS and JS files. Do not import them in the HTML file, they will be automatically imported from the @PdfTemplate()
decorator includes[]
property.
import path from 'path';
import { PdfField, PdfFiller, PdfTable, PdfTemplate } from 'simple-pdf-generator';
@PdfTemplate({
templatePath: path.join(__dirname, 'template.html'),
includes: [{ path: path.join(__dirname, 'template.css') }, { path: path.join(__dirname, 'template.js') }],
})
export class Template extends PdfFiller {
@PdfField()
firstField = '';
@PdfField()
secondField = '';
}
PdfFiller
Extend abstract class PdfFiller
and use the following decorators on the properties:
Decorator | HTML use |
---|---|
PdfField | %%propertyName%% |
PdfTable | <inject-table items="propertyName"> <inject-column prop="name" label="Name"/> <inject-column prop="surname" label="Surname"/> </inject-table> |
fill
PdfFiller fill()
method returns the buffer of the PDF file.
Parameter | Description |
---|---|
outputPath: string | PDF output dir |
pdfOptions: puppeteer.PDFOptions | Object with Puppeteer PDF Options |
PdfFiller fill()
method accept two optional parameters:
outputPath
for the generated file;pdfOptions
that accept Puppeteer PDF Options
;PdfTemplate
Use: decorator to be applied to PdfFiller
class.
Parameter | Description |
---|---|
templatePath: string | Path to the HTML file |
pdfOptions: puppeteer.PDFOptions | Object with Puppeteer PDF Options |
xssProtection: boolean | Enable or disable XSS protection (default: true ) |
includes: Asset[] | Assets (css and js ) to be included in the HTML file |
export interface Asset {
path?: string;
content?: string;
type?: 'css' | 'js';
}
Puppeteer PDF Options
For Puppeteer PDF Options
look at their documentation, we show only a brief example:
pdfOptions = {
background: true,
displayHeaderFooter: true,
headerTemplate: `
<style>
div { margin-left: 15px !important }
</style>
<div>Header</div>
`,
footerTemplate: `
<div>Footer</div>
`,
margin: {
top: '5cm',
bottom: '4cm',
left: '1cm',
right: '1cm',
},
};
Puppeteer PDF Options
used in the decorator of the class.
@PdfTemplate({
templatePath: path.join(__dirname, 'template.html'),
includes: [{ path: path.join(__dirname, 'template.css') }, { path: path.join(__dirname, 'template.js') }],
pdfOptions: pdfOptions,
})
export class Template extends PdfFiller {
@PdfField()
firstField = '';
@PdfField()
secondField = '';
}
Puppeteer PDF Options
passed to fill
method.
const doc = new Template();
doc.firstField = 'World';
doc.secondField = 'Simple PDF Generator';
doc.fill(path.join(__dirname, 'doc.pdf'), pdfOptions);
It's possible to use environment variables to modify the behaviour of the library.
Environment variable | Possible values | Description |
---|---|---|
PUPPETEER_NO_HEADLESS | true , false | Used to run Chromium in non headless mode. |
PUPPETEER_NO_SANDBOX | true , false | Used to run Chromium in containers where a root user is used. |
PUPPETEER_PRODUCT | chrome , firefox | Specify which browser to download and use. |
PUPPETEER_CHROMIUM_REVISION | a chromium version | Specify a version of Chromium you’d like Puppeteer to use. |
PUPPETEER_SKIP_CHROMIUM_DOWNLOAD | true , false | Puppeteer will not download the bundled Chromium. You must provide PUPPETEER_EXECUTABLE_PATH . |
PUPPETEER_EXECUTABLE_PATH | a path | Specify an executable path to be used in puppeteer.launch . To be specified if PUPPETEER_SKIP_CHROMIUM_DOWNLOAD is set to true . |
HTTP_PROXY , HTTPS_PROXY , NO_PROXY | proxy url | Defines HTTP proxy settings that are used to download and run Chromium. |
This library is developed by:
FAQs
Generator of PDF files from HTML templates using TS decorators
The npm package simple-pdf-generator receives a total of 17 weekly downloads. As such, simple-pdf-generator popularity was classified as not popular.
We found that simple-pdf-generator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.